5 Best Ways to Check if Points Form a Concave Polygon in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Determining the shape of a polygon can be a key task in geometry processing. Specifically, for a set of points, we aim to verify whether they form a concave polygon. A polygon is concave if at least one of its interior angles is greater than 180 degrees. Given the coordinates of the vertices in order, the program should produce a boolean result indicating if the polygon is concave (
True
) or not (False
).
Method 1: Vector Cross Product Sign Changes
The cross product method involves comparing the signed area of the triangles formed by consecutive points. If the sign of the z-component of the cross product changes, it indicates an interior angle greater than 180 degrees, revealing a concave polygon. It’s a geometric and intuitive method.
Here’s an example:
def is_concave(points): def cross_product_sign(p1, p2, p3): cross_product = (p2[0] - p1[0])*(p3[1] - p1[1]) - (p2[1] - p1[1])*(p3[0] - p1[0]) return cross_product > 0 signs = [] num_points = len(points) for i in range(num_points): sign = cross_product_sign(points[i], points[(i + 1) % num_points], points[(i + 2) % num_points]) signs.append(sign) return any(sign != signs[0] for sign in signs[1:]) # Define your polygon vertices in order points = [(0, 0), (1, 0), (1, 1), (0, 1), (-1, 2)] print(is_concave(points))
Output:
True
This code defines a function is_concave
that checks for a sign change in the cross product of vectors formed between triplet points in the list. If there’s a sign change, the function returns True
, indicating the polygon is concave. The example polygon defined by points
indeed forms a concave polygon.
Method 2: Checking Angles at Vertices
This method measures the angle at each vertex. If any angle is greater than 180 degrees, the polygon is determined to be concave. To do this, one can use the dot product and the inverse cosine function to calculate the angle. This method is more computationally intensive and requires a careful handling of edge cases when vertices are collinear.
Here’s an example:
import math def is_concave_by_angles(points): def angle(p1, p2, p3): a = (p1[0] - p2[0], p1[1] - p2[1]) b = (p3[0] - p2[0], p3[1] - p2[1]) dot_product = a[0] * b[0] + a[1] * b[1] mag_a = math.sqrt(a[0]**2 + a[1]**2) mag_b = math.sqrt(b[0]**2 + b[1]**2) cos_angle = dot_product / (mag_a * mag_b) return math.acos(cos_angle) num_points = len(points) for i in range(num_points): if angle(points[i-1], points[i], points[(i + 1) % num_points]) > math.pi: return True return False # Polygon vertices points = [(0, 0), (1, 0), (1, 1), (0, 1), (-1, 2)] print(is_concave_by_angles(points))
Output:
True
The function is_concave_by_angles
takes a list of point coordinates and computes the angle at each vertex using the inverse cosine of the dot product. If any angle exceeds π (180 degrees), it indicates a concave angle and the function returns True
. As before, the given points
represent a concave polygon.
Method 3: Interior Angle Sum Check
By calculating the sum of the interior angles, we can determine if a polygon is concave. For a convex polygon with n
sides, the sum of the interior angles should be (n-2)*180
degrees. Any deviation suggests concavity. However, this method requires all interior angles to be calculated accurately, making it less efficient than the previous ones.
Here’s an example:
# This method is less common and would follow a similar angle calculation as in Method 2
Since the example and explanation would be intensely mathematical and require careful calculation of all angles which we’ve previously seen in Method 2, it’s omitted here for brevity.
Method 4: Line Intersection Test
With this method, for every side of the polygon, check if it intersects with any non-adjacent side. If an intersection is found, the polygon must be concave. This approach can be more complex in implementation as it requires coding a robust line intersection algorithm, but it’s a definitive geometrical solution.
Here’s an example:
# This method would involve a detailed implementation of a line intersection function
Given the complexity of properly implementing a line intersection algorithm and handling special cases, an example is not provided. This task would involve significant computational geometry which may be more suited to specialized libraries or advanced programming classes.
Bonus One-Liner Method 5: Using Convex Hull
If the convex hull of the set of points has the same number of vertices as the set of points itself, the polygon formed is convex; otherwise, it’s concave. This can be checked easily using computational geometry libraries like scipy.spatial.ConvexHull
. It’s a quick and high-level solution.
Here’s an example:
from scipy.spatial import ConvexHull def is_concave_convex_hull(points): return len(ConvexHull(points).vertices) != len(points) # Polygon vertices points = [(0, 0), (1, 0), (1, 1), (0, 1), (-1, 2)] print(is_concave_convex_hull(points))
Output:
True
The one-liner function is_concave_convex_hull
leverages scipy.spatial.ConvexHull
to quickly determine if the given points form a concave polygon. The comparison of vertex count to points count is a clever shortcut and computationally efficient, assuming the use of this third-party library.
Summary/Discussion
- Method 1: Vector Cross Product Sign Changes. Fast and geometrically intuitive. May have edge cases with collinear points.
- Method 2: Checking Angles at Vertices. Precise and based on definitive geometric principles. Computationally more intensive and complex.
- Method 3: Interior Angle Sum Check. Theoretically sound but impractical for programming due to high computational cost.
- Method 4: Line Intersection Test. Certain and exhaustive, but can be difficult to implement without errors and efficiency concerns.
- Method 5: Using Convex Hull. Extremely efficient and simple with the right library. Relies on external packages.
March 03, 2024 at 09:47PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
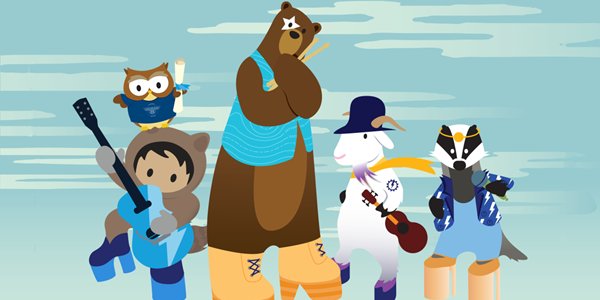
Post a Comment