Python Sort List of Strings by Length : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When working with lists of strings in Python, you may encounter a scenario where you need to sort the list not by alphabetical order, but by the length of the strings. For instance, given a list
["apple", "fig", "banana"]
, you’d want to reorganize the list to ["fig", "apple", "banana"]
, arranging the words by ascending length.
Method 1: Using sorted() with len()
The built-in sorted()
function can be used to sort any iterable. When sorting a list of strings by length, you can pass len
as the key
argument, which instructs sorted()
to sort by the length of the strings.
Here’s an example:
fruits = ["apple", "fig", "banana"] sorted_fruits = sorted(fruits, key=len) print(sorted_fruits) # Output: ["fig", "apple", "banana"]
In this snippet, sorted(fruits, key=len)
tells Python to sort the fruits
list, using the length of each string as the sorting key. The sorted list is ["fig", "apple", "banana"]
, ordered by ascending string length.
Method 2: Using the sort() Method with a Lambda Function
The sort()
method sorts a list in place. If you want to sort a list by the lengths of its strings, you can use a lambda function as the key, which will be used to determine the strings’ lengths during the sort.
Here’s an example:
fruits = ["apple", "fig", "banana"] fruits.sort(key=lambda fruit: len(fruit)) print(fruits) # Output: ["fig", "apple", "banana"]
By calling fruits.sort(key=lambda fruit: len(fruit))
, we sort fruits
in place, using a lambda function that returns the length of each string as the key. The list is modified to ["fig", "apple", "banana"]
, with shorter strings coming first.
Method 3: Using the sort() Method with len()
Similarly to the sorted function, the sort()
method can also be used with len()
to sort the list of strings by their lengths, manipulating the original list rather than creating a copy.
Here’s an example:
fruits = ["apple", "fig", "banana"] fruits.sort(key=len) print(fruits) # Output: ["fig", "apple", "banana"]
The expression fruits.sort(key=len)
sorts the fruits
list in place, using the length of the strings as the key for sorting. The manipulated list appears as ["fig", "apple", "banana"]
, sorted by ascending length.
Method 4: Using List Comprehension With Tuple Sorting
Python sorts tuples based on the first element by default. You can create a list of tuples, where the first element is the string length, and then sort it. Finally, use list comprehension to extract the sorted strings.
Here’s an example:
fruits = ["apple", "fig", "banana"] fruits_with_length = [(len(fruit), fruit) for fruit in fruits] fruits_with_length.sort() sorted_fruits = [fruit for _, fruit in fruits_with_length] print(sorted_fruits) # Output: ["fig", "apple", "banana"]
This code first pairs each string with its length in a tuple, sorts the list of tuples, and then strips away the lengths, leaving a list of strings sorted by length: ["fig", "apple", "banana"]
.
Bonus One-Liner Method 5: Using sorted() and a Generator Expression
For a concise one-liner, you can combine sorted()
with a generator expression, which creates an iterable of tuples with string lengths and strings.
Here’s an example:
fruits = ["apple", "fig", "banana"] sorted_fruits = [fruit for _, fruit in sorted((len(fruit), fruit) for fruit in fruits)] print(sorted_fruits) # Output: ["fig", "apple", "banana"]
This one-liner sorts a generator expression that produces tuples of string lengths and strings. The result is a new list of strings sorted by length: ["fig", "apple", "banana"]
.
Summary/Discussion
- Method 1 (
sorted()
withlen()
): Returns a new sorted list; non-destructive. - Method 2 (
sort()
with Lambda): Sorts the list in place using a lambda function; efficient for long lists. - Method 3 (
sort()
withlen()
): Sorts the list in place; fewer keystrokes than a lambda function. - Method 4 (List Comprehension with Tuple Sorting): More complex; helpful when additional sorting conditions are needed.
- Bonus Method 5 (One-Liner with
sorted()
and Generator): Extremely concise; best for those who prefer one-liners.
For immutable sorting, Method 1 is preferred, while Method 2 and 3 provide in-place sorting. Method 4 and the Bonus Method 5 offer a different syntax style but might be less readable for beginners.
February 09, 2024 at 06:21PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
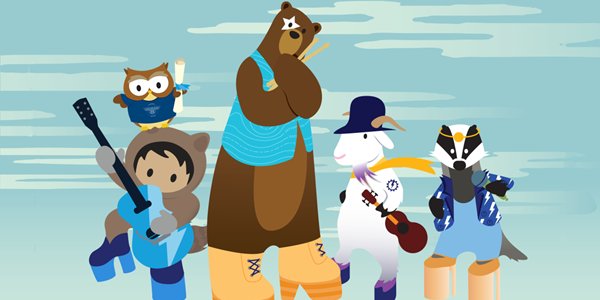
Post a Comment