5 Pythonic Ways to Check if a String Is Empty : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: This article addresses how to determine whether a string in Python is empty—a common task while handling text data or input validation. An empty string is defined as one that contains no characters and has a length of zero. An example of an input and desired output scenario might be: given the variable
greeting = ""
, verifying that greeting
is indeed an empty string would return True
.
Method 1: Using the Equality Operator
The equality operator ==
in Python can be used to check if a string is empty by comparing it directly to an empty string literal ""
. This is the most straightforward and readable method. It is also the direct way to convey intent in your code.
Here’s an example:
string_to_check = "" is_empty = string_to_check == "" print(f'Is the string empty? {is_empty}')
Output:
Is the string empty? True
This code snippet evaluates whether string_to_check
is equal to ""
. If so, is_empty
becomes True
, and the print statement confirms the string is empty.
Method 2: Using the not Operator
Python’s not
operator can be applied to the string. If the string is empty, it will equate to True
because empty strings are considered “Falsy” in Python. It’s an implicit way to check for emptiness without comparing directly.
Here’s an example:
name = "" if not name: print("The name string is empty!") else: print("The name string has content!")
Output:
The name string is empty!
In this example, if not name
implicitly checks if name
is empty. As it is, “The name string is empty!” gets printed.
Method 3: Using the len() Function
The len()
function in Python determines the number of items in an object. When used on a string, it counts the number of characters. An empty string will have a length of zero, and thus, len()
can be a clear numeric way to check for emptiness.
Here’s an example:
message = "" is_empty = len(message) == 0 print("The message is empty:", is_empty)
Output:
The message is empty: True
This snippet uses len()
to check the length of message
. Since the length is zero, is_empty
is set to True
.
Method 4: Using the strip() Method
In scenarios where you might consider strings with whitespace as empty, the strip()
method can be used. It removes whitespace from the start and end of a string. When there’s nothing but whitespaces, the result is an empty string, which can then be checked using Method 1 or 2.
Here’s an example:
saying = " " is_empty = not saying.strip() print("Is the saying actually empty?", is_empty)
Output:
Is the saying actually empty? True
The code checks if saying.strip()
, which is an empty string after stripping whitespace, is empty using the not
operator.
Bonus One-Liner Method 5: Use a Boolean Cast
For a quick one-liner, you could use the Boolean cast method which relies on the truthiness of the string in Python. An empty string will return False
when converted to a Boolean, which you can then negate to get a direct answer.
Here’s an example:
motto = "" is_empty = not bool(motto) print("Is the motto empty?", is_empty)
Output:
Is the motto empty? True
This compact snippet effectively checks for an empty string using a cast to a Boolean where not bool(motto)
would be True
for an empty string.
Summary/Discussion
- Method 1: Equality Operator. Simple and very readable. Does not strip whitespace.
- Method 2: Not Operator. Falsy truthiness of Python objects utilized. Also doesn’t strip whitespace.
- Method 3: Length Check. Numerically explicit. Can be overkill for such a simple check.
- Method 4: Strip Method. Takes care of strings with whitespaces deemed empty. Adds an extra step if whitespace is not a concern.
- Method 5: Boolean Cast. Very concise one-liner but can be less readable to beginners.
February 14, 2024 at 11:40PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
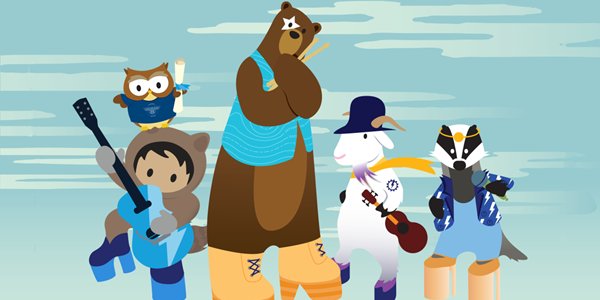
Post a Comment