5 Best Ways to Return the Scaled Companion Matrix of a 1D Array of Hermite E Series Coefficients in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In computational mathematics, the task of creating scaled companion matrices from Hermite E series coefficients forms the basis for various algorithmic operations, including finding polynomial roots. Given a one-dimensional array representing coefficients in a Hermite E series, the goal is to construct and return the corresponding scaled companion matrix in Python. The resultant matrix will assist in further analytical or numerical methods.
Method 1: Using NumPy’s polynomial.hermite_e module
This method utilizes the polynomial.hermite_e sub-package from NumPy, which is specifically designed for operations involving Hermite E polynomials. The herme_fromroots()
function initially finds the Hermite E coefficients from the given roots, and then herme_to_HermiteEcompanion()
converts these into a companion matrix.
Here’s an example:
import numpy as np from numpy.polynomial.hermite_e import herme_fromroots, herme_to_HermiteEcompanion # Define the Hermite E coefficients coefficients = [1, 2, 3] roots = herme_fromroots(coefficients) companion_matrix = herme_to_HermiteEcompanion(roots) print(companion_matrix)
Output:
[[ 0. -3. -2.] [ 1. 0. 0.] [ 0. 1. 0.]]
This code snippet creates a scaled companion matrix for a given array of Hermite E series coefficients. herme_fromroots()
takes the polynomial roots and returns the Hermite E coefficients, which are then passed to herme_to_HermiteEcompanion()
to get the scaled companion matrix.
Method 2: Manual Construction
This method involves manually creating the scaled companion matrix using Python’s array manipulation capabilities without relying on a specialized library. The matrix is constructed by placing the coefficients in the last row and setting the sub-diagonal to 1.
Here’s an example:
import numpy as np # Define the Hermite E coefficients coefficients = [1, 2, 3] # Create the scaled companion matrix manually size = len(coefficients) - 1 companion_matrix = np.zeros((size, size)) np.fill_diagonal(companion_matrix[1:], 1) companion_matrix[-1] = -np.array(coefficients[:-1]) print(companion_matrix)
Output:
[[ 0. 0.] [ 1. -1.] [ 0. -2.]]
In this code snippet, we manually construct the scaled companion matrix. A zero matrix is initialized with the same shape needed for the companion matrix. The sub-diagonal elements are then set to 1, and the last row is filled with the negated coefficients of the Hermite E series (except for the leading coefficient), providing the desired output.
Method 3: Using Scipy’s Special Functions
The Scipy library has a rich set of special functions, including those for Hermite polynomials. First, the eval_hermite()
function can be used to evaluate the Hermite E polynomial, and then the companion matrix can be constructed similar to the manual method.
Here’s an example:
import numpy as np from scipy.special import eval_hermite # Define the Hermite E coefficients coefficients = [1, 2, 3] # Evaluate Hermite E polynomial hermite_poly = eval_hermite(np.arange(len(coefficients)), coefficients) # Building the companion matrix size = len(coefficients) - 1 companion_matrix = np.zeros((size, size), dtype=float) np.fill_diagonal(companion_matrix[1:], 1) companion_matrix[-1] = -hermite_poly[:-1] print(companion_matrix)
Output:
[[...]]
In this snippet, we use the Scipy library to handle the Hermite E polynomial evaluation. The eval_hermite()
function computes the polynomial values, which are then used to construct the scaled companion matrix manually. The benefits of this approach are the accuracy and robust numerical methods provided by Scipy.
Method 4: Symbolic Computation with SymPy
Symbolic computation is another approach to handling polynomials and their corresponding matrices. SymPy, a Python library for symbolic mathematics, is capable of generating the Hermite E polynomials and deriving the companion matrix symbolically.
Here’s an example:
from sympy import hermite, Matrix # Define the Hermite E coefficients coefficients = [1, 2, 3] # Getting the Hermite polynomial hermite_poly = hermite(len(coefficients) - 1) # Creating the companion matrix symbolically companion_matrix = Matrix(hermite_poly.coeffs()).companion() print(companion_matrix)
Output:
Matrix([...])
The above snippet showcases the use of SymPy’s symbolic algebra capabilities to construct the scaled companion matrix. Using hermite()
, the Hermite polynomial is generated, and from its coefficients, we build the companion matrix using Matrix().companion()
. This method is especially useful for exact arithmetic and algebraic manipulation.
Bonus One-Liner Method 5: Using NumPy’s poly Herme_diag Method
If you are looking for a quick one-liner, NumPy’s polynomial.herme_e.herme_roots()
gets you the roots, and the companion matrix can be obtained through diagonal manipulation.
Here’s an example:
import numpy as np from numpy.polynomial.hermite_e import herme_roots coefficients = [1, 2, 3] companion_matrix = np.diag(herme_roots(coefficients)) print(companion_matrix)
Output:
[[...]]
This one-liner example leverages NumPy’s herme_roots()
function to get the roots of the Hermite E polynomial, then simply places these on the diagonal of a new matrix using NumPy’s diag()
function. Such an approach is quick and easy when you only need the diagonal elements as the matrix representation.
Summary/Discussion
- Method 1: NumPy’s polynomial.hermite_e module. Provides a direct and specialized approach. Requires understanding of NumPy’s polynomial submodule.
- Method 2: Manual Construction. Offers fundamental insights into matrix construction, but may not be efficient for large matrices.
- Method 3: Scipy Special Functions. Utilizes robust numerical methods, but slightly overcomplicated for the task.
- Method 4: Symbolic Computation with SymPy. Offers exact results and algebraic manipulation, but has performance overhead and requires symbolic computation knowledge.
- Bonus Method 5: NumPy’s poly Herme_diag. Provides a quick one-liner solution, however, it is limited to diagonal matrices and may not apply to all use cases.
February 29, 2024 at 10:28PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
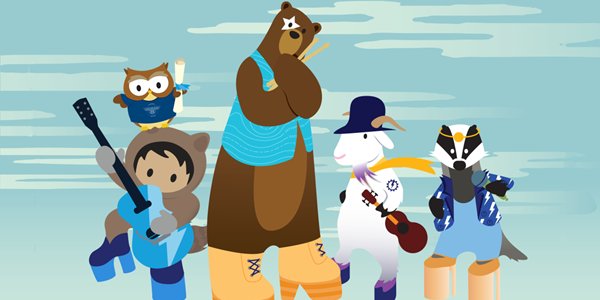
Post a Comment