5 Best Ways to Join a List of Strings with Quotes in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In Python programming, developers often need to combine a list of strings into a single string, with each element enclosed in quotes. For example, given the input list
['apple', 'banana', 'cherry']
, the desired output would be "'apple', 'banana', 'cherry'"
. The quoted strings are particularly useful for generating SQL queries, JSON strings, or preparing data for display. This article illustrates five effective methods to accomplish this task.
Method 1: Using join()
with map()
and Formatting
Combining the built-in join()
method with map()
and string formatting allows for efficient concatenation of list elements within quotes. It maps each element to a quoted version and then joins them together.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] quoted_fruits = ", ".join(map(lambda f: f"'{f}'", fruits)) print(quoted_fruits)
Output:
'apple', 'banana', 'cherry'
This code snippet uses map()
to apply a lambda function that surrounds each fruit with single quotes. The join()
method is then used to concatenate these quoted strings with a comma and a space.
Method 2: List Comprehension with join()
List comprehension offers a concise way to create a new list by applying an expression to each item in the original list. When combined with join()
, it elegantly adds quotes to list elements during concatenation.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] quoted_fruits = ", ".join([f"'{fruit}'" for fruit in fruits]) print(quoted_fruits)
Output:
'apple', 'banana', 'cherry'
In this snippet, a new list of strings is generated, with each string in the list enclosed in single quotes, using list comprehension. The join()
method concatenates these quoted elements into a single string.
Method 3: Using join()
with Expression Interpolation
Expression interpolation with f-strings (introduced in Python 3.6) simplifies the insertion of variables or expressions within string literals. Used with join()
, it creates a readable and direct approach for adding quotes.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] quoted_fruits = ", ".join(f"'{fruit}'" for fruit in fruits) print(quoted_fruits)
Output:
'apple', 'banana', 'cherry'
This snippet employs a generator expression with f-strings. Each fruit is enclosed in quotes, and the resulting generator is passed to the join()
method to form the quoted, comma-separated string.
Method 4: Using str.join()
with Manual Iteration and Concatenation
Manually iterating over list elements and concatenating them into one string with quotes guarantees full control over the process, albeit requiring more code than other methods.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] quoted_fruits = '' for fruit in fruits: quoted_fruits += f"'{fruit}', " quoted_fruits = quoted_fruits.strip(', ') # Removes the trailing comma and space print(quoted_fruits)
Output:
'apple', 'banana', 'cherry'
The provided code snippet manually constructs a string of quoted fruit names with a trailing comma and space after each fruit. Finally, strip()
is used to remove the extraneous characters at the end of the string.
Bonus One-Liner Method 5: Using repr()
with join()
The function repr()
returns the official string representation of an object, which includes quotes. This can be cleverly combined with join()
for a one-liner solution.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] quoted_fruits = ", ".join(repr(fruit) for fruit in fruits) print(quoted_fruits)
Output:
'apple', 'banana', 'cherry'
This method uses repr()
within a generator expression to get a string representation of each list item, including quotes, before joining them with commas and spaces.
Summary/Discussion
- Method 1:
join()
withmap()
and Formatting. Strengths: It’s efficient and functional. Weaknesses: May be less readable to those unfamiliar withmap()
or lambda functions. - Method 2: List Comprehension with
join()
. Strengths: Highly readable and idiomatic Python. Weaknesses: Slightly less performant than the map-based approach. - Method 3: Using
join()
with Expression Interpolation. Strengths: Very readable, modern Python syntax. Weaknesses: Only available in Python 3.6 and later. - Method 4: Manual Iteration and Concatenation. Strengths: Offers fine-grained control. Weaknesses: Verbosity and potential for inefficiency with large lists.
- Method 5: Using
repr()
withjoin()
. Strengths: Simple and clever one-liner. Weaknesses: May include additional characters for certain objects’s repr() that aren’t ideal for all contexts.
February 18, 2024 at 07:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
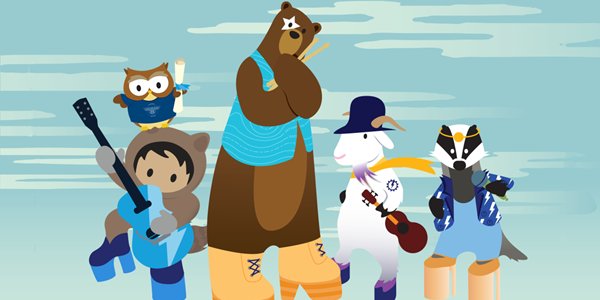
Post a Comment