5 Best Ways to Join a List of Strings with a Comma in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In Python programming, it is a common requirement to convert a list of strings into a single string separated by a delimiter, such as a comma. For example, given an input list
['apple', 'banana', 'cherry']
, the desired output is the string 'apple,banana,cherry'
. This article discusses different methods to achieve this concatenation.
Method 1: Using the join()
function
The join()
function in Python is specifically designed to concatenate the elements of an iterable (like a list or tuple) into a new string, separated by a string that join()
is called on. This method is straightforward and highly efficient for concatenating strings.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] result = ','.join(fruits) print(result)
Output:
apple,banana,cherry
This code snippet creates a list of fruit names and uses the join()
function, called on a string containing a single comma, to concatenate the list elements into one string, with each element separated by a comma.
Method 2: Using a for loop
A for loop can be used to iterate through each element in the list and manually add it to a new string, along with a comma. This is a more manual approach and is useful if additional processing is required during the join operation.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] result = '' for fruit in fruits: result += fruit + ',' result = result.rstrip(',') # Remove trailing comma print(result)
Output:
apple,banana,cherry
The for loop adds each fruit name to the result string followed by a comma. The trailing comma is removed at the end using the rstrip()
method.
Method 3: Using list comprehension
In Python, list comprehension offers a compact way of creating lists. It can be combined with the join()
function to achieve the same result as in Method 1, but with a more “Pythonic” approach.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] result = ','.join([fruit for fruit in fruits]) print(result)
Output:
apple,banana,cherry
The list comprehension creates a new list on the fly, which is immediately used by the join()
function to form the comma-separated string.
Method 4: Using the map()
function
The map()
function applies a given function to each item of an iterable and returns a list of the results. When combined with join()
, it is especially useful if the original list contains non-string data types that need to be converted to strings first.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] result = ','.join(map(str, fruits)) print(result)
Output:
apple,banana,cherry
The map()
function applies the str()
function to each item in the list, ensuring all elements are strings, which can then be joined by the join()
function.
Bonus One-Liner Method 5: Using the str.join()
function with a Generator Expression
A generator expression is similar to list comprehension but does not store the entire list in memory. This can be more memory-efficient when dealing with large datasets.
Here’s an example:
fruits = ['apple', 'banana', 'cherry'] result = ','.join(fruit for fruit in fruits) print(result)
Output:
apple,banana,cherry
This one-liner uses a generator expression directly inside the join()
function, creating an efficient means of concatenating the list items with a comma.
Summary/Discussion
- Method 1:
join()
Function. This is the standard and most efficient way to join list items with a comma. However, it cannot be used directly if the list contains non-string elements. - Method 2: For Loop. Gives the programmer granular control over the concatenation process and is good for additional processing. It’s less Pythonic and efficient than using
join()
. - Method 3: List Comprehension. Offers a Pythonic way to join strings and can be condensely written, but doesn’t offer any performance benefits over Method 1.
- Method 4:
map()
Function. Useful when elements need to be processed or converted to strings, but it’s an overkill for simple string lists. - Bonus Method 5: Generator Expression. Memory efficient for large datasets and still as compact as list comprehension, but might be less intuitive to Python beginners.
February 18, 2024 at 07:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
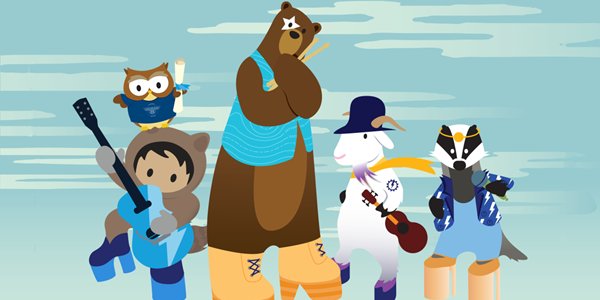
Post a Comment