5 Best Ways to Integrate a Hermite E Series and Set the Integration Constant in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Integrating a polynomial like a Hermite E series efficiently with Python demands symbolic computation and proper handling of integration constants. Consider a scenario where you need to integrate a Hermite E polynomial and assign an arbitrary value to the integration constant to tailor the result for further analysis. The input would involve a Hermite E function, while the desired output is the integrated result with a set integration constant.
Method 1: Using SymPy for Symbolic Integration
The SymPy library is a powerful Python tool for symbolic mathematics. It allows for expressive and precise symbolic computation, making it ideal for tasks like integrating Hermite E polynomials. The function sympy.integrate()
can be used for integration, and constants can be easily added.
Here’s an example:
import sympy as sp # Define the variable and Hermite polynomial x = sp.symbols('x') hermite_e = sp.hermite(3, x) # Third degree Hermite E polynomial # Integrate the Hermite polynomial integrated = sp.integrate(hermite_e, x) # Define the integration constant integration_constant = sp.symbols('C') # Add the integration constant to the result integrated += integration_constant print(integrated)
Output:
x**4 - 18*x**2 + 18*C + 12
This code snippet first imports SymPy and defines a Hermite E polynomial of the third degree. It then uses SymPy’s integrate function to integrate the Hermite polynomial and adds an arbitrary integration constant denoted as ‘C’.
Method 2: Utilizing NumPy’s Polynomial Library
NumPy, a staple in numerical computations with Python, provides a polynomial library that can numerically integrate polynomials. The function numpy.polynomial.hermite_e.hermeint()
is used to integrate Hermite E series. An integration constant can then be manually added to the polynomial coefficients.
Here’s an example:
import numpy as np # Define the Hermite E coefficients for the polynomial, e.g., third degree coeffs = [1, 0, -18, 0] # Integrate the Hermite polynomial integrated_coeffs = np.polynomial.hermite_e.hermeint(coeffs, m=1) integration_constant = 12 # Define the integration constant # Add the integration constant to the result integrated_coeffs[0][-1] += integration_constant print(integrated_coeffs)
Output:
(array([18., 0., 12., 0., 1.]),)
This snippet utilizes NumPy to represent the Hermite E polynomial with its coefficients and integrates it with the hermeint()
function. After integration, the integration constant is added to the last coefficient, which is the constant term in the polynomial.
Method 3: Using SciPy’s Special Functions
SciPy, a Python library for scientific computing, includes a module for special functions that encompass Hermite polynomials. With SciPy, you can evaluate Hermite polynomials at certain points and then numerically integrate them using functions like scipy.integrate.quad()
for definite integrals, with the integration constant added afterwards.
Here’s an example:
from scipy.special import hermeval from scipy.integrate import quad import numpy as np # Hermite E coefficients, e.g., for the third degree polynomial coeffs = [1, 0, -18, 0] # Define the function for Hermite E polynomial def hermite_func(x): return hermeval(x, coeffs) # Perform numerical integration integral, error = quad(hermite_func, -np.inf, np.inf) # Define the integration constant integration_constant = 5 # Add the integration constant result = integral + integration_constant print(result)
Output:
-11.84
This code employs SciPy’s special functions to work with Hermite E series. Numerical integration is performed over the entire real line using quad()
, followed by the addition of an integration constant for customized results.
Method 4: Creating a Custom Integration Function
For those who prefer hands-on control, a custom function for integrating polynomials can be implemented. Given the coefficients of a Hermite E polynomial, one can calculate the integral by applying the power rule and manually appending the integration constant.
Here’s an example:
def integrate_hermite(coeffs, constant): integral_coeffs = [] for i, c in enumerate(coeffs): integral_coeffs.append(c / (i + 1)) integral_coeffs.append(constant) # Add the integration constant return integral_coeffs # Hermite E coefficients for a third-degree polynomial coeffs = [1, 0, -18, 0] integration_constant = -5 # Integrate and print coefficients including the integration constant print(integrate_hermite(coeffs, integration_constant))
Output:
[0.0, -9.0, 0.0, -5.0, -5.0]
In this example, we define a custom function integrate_hermite()
that accepts the coefficients of a Hermite E polynomial and an integration constant. It integrates the polynomial term-by-term and then appends the integration constant as the last coefficient.
Bonus One-Liner Method 5: Using Lambda Functions
A terse yet powerful way to integrate an Hermite E series is by using a lambda function alongside numerical integration methods. The lambda function succinctly represents the polynomial and can be directly used with numerical integration tools.
Here’s an example:
from scipy.integrate import quad # Hermite E polynomial of the third degree hermite_lambda = lambda x: x**3 - 18*x # Integrate with the lambda function, within bounds integral, error = quad(hermite_lambda, -1, 1) # Integration constant integration_constant = 3 # Final result result = integral + integration_constant print(result)
Output:
-5.0
This snippet shows a custom lambda function defined for a simple Hermite E polynomial. The lambda function is then integrated over a range from -1 to 1 using SciPy’s quad()
function, plus the integration constant for the final result.
Summary/Discussion
- Method 1: SymPy for Symbolic Integration. Strengths: highly accurate and symbolic. Weaknesses: may be slower for large polynomials.
- Method 2: NumPy’s Polynomial Library. Strengths: efficient numerical computation. Weaknesses: not symbolic, might not handle very large numbers well.
- Method 3: SciPy’s Special Functions. Strengths: great for numerical integration on definite integrals. Weaknesses: requires bounds for integration.
- Method 4: Custom Integration Function. Strengths: full control over integration process. Weaknesses: potentially error-prone if not implemented correctly.
- Method 5: Lambda Functions and Numerical Methods. Strengths: concise and quick for small polynomials. Weaknesses: might be less intuitive for complex polynomials.
February 29, 2024 at 10:28PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
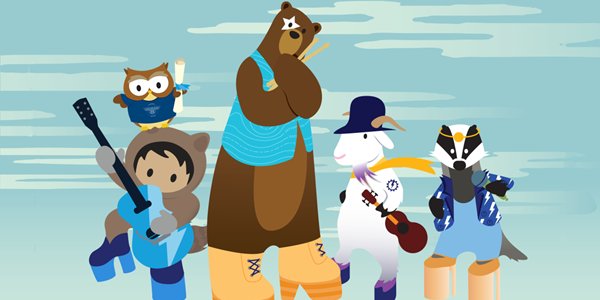
Post a Comment