5 Best Ways to Initialize a List of Dictionaries in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When working with data in Python, it’s common to organize complex information using lists of dictionaries. This approach enables efficient storage of related data with key-value associations within each dictionary in the list. For instance, if you want to store user data such as usernames and email addresses, you would need to initialize a list of dictionaries where each dictionary represents a user:
[{"username": "example", "email": "[email protected]"}, ...]
. The goal is to find the most efficient and readable ways to initialize this data structure.
Method 1: Using a For Loop
Creating a list of dictionaries through a for loop is a straightforward and intuitive way to initialize this data structure. It’s especially handy when the dictionaries are populated based on another sequence or range of values.
Here’s an example:
users = [] for i in range(5): users.append({"id": i, "username": f"user{i}", "email": f"user{i}@example.com"})
Output:
[ {'id': 0, 'username': 'user0', 'email': '[email protected]'}, {'id': 1, 'username': 'user1', 'email': '[email protected]'}, ... ]
The above snippet uses a for loop to append each dictionary to the list users
. We’re using a range of numbers to create unique values for the ‘id’, ‘username’, and ’email’ keys. This method is very clear and easy to modify, perfect for beginners or for cases where the dictionaries might vary greatly in content.
Method 2: List Comprehensions
List comprehensions offer a concise way to create lists. When it comes to initializing a list of dictionaries, a list comprehension can be used to streamline the process and condense the code that would otherwise be written as a for loop.
Here’s an example:
users = [{"id": i, "username": f"user{i}", "email": f"user{i}@example.com"} for i in range(5)]
Output:
[ {'id': 0, 'username': 'user0', 'email': '[email protected]'}, {'id': 1, 'username': 'user1', 'email': '[email protected]'}, ... ]
This snippet achieves the same result as Method 1 but in a more compact form using list comprehension. Each iteration of the loop inside the list comprehension generates a dictionary, which is then automatically appended to the resulting list users
. This method is more Pythonic and is great for lines of code reduction and readability, but it can be less clear to Python newcomers.
Method 3: Using the *
Operator
Python’s *
operator can be used to repeat items in a list. To initialize a list of identical dictionaries without manually copying them, the *
operator can be quite efficient; however, it creates shallow copies, so care should be taken when the dictionaries will be modified.
Here’s an example:
user_template = {"id": None, "username": "", "email": ""} users = [user_template.copy() for _ in range(5)]
Output:
[ {'id': None, 'username': '', 'email': ''}, {'id': None, 'username': '', 'email': ''}, ... ]
Here, instead of individually creating each dictionary, we define a template dictionary and use list comprehension with the .copy()
method to create individual copies for each item in the list users
. This approach is useful when starting with a default structure, but it requires further individual updates if each dictionary is to differ from the template.
Method 4: Using the dict()
Constructor
The dict()
constructor can be used to convert sequences containing key-value pairs into dictionaries. This method is particularly useful for creating dictionaries with a common set of keys.
Here’s an example:
keys = ["id", "username", "email"] values = [(i, f"user{i}", f"user{i}@example.com") for i in range(5)] users = [dict(zip(keys, v)) for v in values]
Output:
[ {'id': 0, 'username': 'user0', 'email': '[email protected]'}, {'id': 1, 'username': 'user1', 'email': '[email protected]'}, ... ]
This code first creates a list of tuples with the values for each dictionary. Then, it uses the dict()
constructor along with zip()
to combine these values with the common set of keys inside a list comprehension. The result is a list of dictionaries with the same keys but different values. It’s a neat method for ensuring consistency of keys across dictionaries.
Bonus One-Liner Method 5: Dictionary Comprehensions
Dictionary comprehensions, similar to list comprehensions, allow for the direct creation of dictionaries. A list of dictionary comprehensions can also be used to create a list of dictionaries in a concise one-liner.
Here’s an example:
users = [{k: f"{k}{i}" for k in ["id", "username", "email"]} for i in range(5)]
Output:
[ {'id': 'id0', 'username': 'username0', 'email': 'email0'}, {'id': 'id1', 'username': 'username1', 'email': 'email1'}, ... ]
This snippet uses a dictionary comprehension within a list comprehension to generate individual dictionaries. It dynamically constructs keys and values based on the iteration variable. This method is slick and Pythonic, but the complexity might be a bit high if the code needs to be understood by novices.
Summary/Discussion
- Method 1: For Loop. Easy to understand. Best for variable dictionary content. Can be verbose.
- Method 2: List Comprehension. Compact. Pythonic. Might be less clear for beginners.
- Method 3: Using
*
Operator with.copy()
. Good for initializing default structures. Requires additional steps for unique elements. - Method 4:
dict()
Constructor. Ensures consistency of keys. Might be less straightforward for complex structures. - Method 5: Dictionary Comprehension. Very concise. Can handle complex constructions. Not as beginner-friendly.
February 22, 2024 at 07:25PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
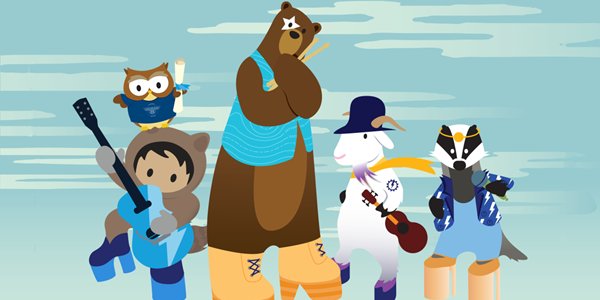
Post a Comment