5 Best Ways to Display an Image on Hovering Over a Point in Python Plotly : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When creating interactive visualizations in Python with Plotly, a common desire is to enrich the user experience by providing additional context through images when hovering over a data point. This article will unravel the problem by demonstrating how to display an image upon hover, which enhances data interpretation for plots like scatter plots or maps. Assume we have a scatter plot with coordinate points and images assigned to each; our task is to show the associated image when a user hovers over a particular point.
Method 1: Using the Hovertemplate Attribute
This method involves utilizing Plotly’s hovertemplate
attribute within the trace definition to specify HTML content, including image tags, that is displayed in the tooltip. This method can reference online image URLs directly and is particularly useful for datasets with images hosted on the web. By specifying the HTML tag in the hovertemplate string, we can craft a custom hover label that includes an image.
Here’s an example:
import plotly.graph_objs as go trace = go.Scatter( x=[1, 2, 3], y=[4, 5, 6], mode='markers', hoverinfo='none', hovertemplate='<img src="https://example.com/image_%{x}.jpg" width="200" height="100"><extra>%{y}</extra>' ) fig = go.Figure(data=[trace]) fig.show()
The output of this code is an interactive scatter plot where hovering over each point displays an image sourced from “https://ift.tt/MQ1wDmL;, where x is the x-coordinate of the point.
This code snippet sets up a scatter plot with custom hover templates for each point. The hovertemplate
includes an image tag (<img>
) with a dynamic URL based on the x-coordinate of the point. The <extra>
tag is used to add additional text to the hover label. The image will appear when hovering over a point along with the y-coordinate as additional text.
Method 2: Custom Data and JS Events
Integrate custom JavaScript (JS) with Plotly’s event system to react when users hover over points. This method involves including a custom data field with the image URL in each point’s data, which can then be accessed via JS when the point is hovered on. It offers flexibility and is suitable when you have complex interactivity or need to include images that are not accessible by URL (e.g., locally stored images).
Here’s an example:
import plotly.graph_objs as go import json trace = go.Scatter( x=[1, 2, 3], y=[4, 5, 6], mode='markers', customdata=['image_1.jpg', 'image_2.jpg', 'image_3.jpg'] ) fig = go.Figure(data=[trace]) fig.update_layout(hovermode='closest') # Assume that display_image is a JS function that handles the image display fig.write_html('plot.html', include_plotlyjs='cdn', full_html=False, auto_play=False, post_script=""" window.PLOTLYENV=window.PLOTLYENV || {}; var gd = document.getElementById('plot'); gd.on('plotly_hover', function(data){ var infotext = data.points.map(function(d){ return (d.customdata); }); display_image(infotext[0]); })""") # To view, open the resulting "plot.html" file in a web browser
When you open the `plot.html` file in a web browser, you’ll see a scatter plot where hovering over each point triggers a call to the `display_image` function with the image filename passed as a parameter.
In this code, each data point’s custom data field is populated with a corresponding image filename. Whenever a hover event is triggered on the graph, a JavaScript function `display_image` is called with the image filename as an argument. The function `display_image` is assumed to be user-defined and responsible for displaying the image. This JS snippet is inserted into the exported HTML file that contains the Plotly plot.
Method 3: Image Overlays on Hover
Create a secondary invisible plot that reacts to hover events by displaying images as markers. This complex method relies on synchronizing two plots – one for the actual data and another solely for the purpose of image display upon hovering. It is especially useful when images must appear as if they are part of the chart itself rather than in a separate tooltip.
Here’s an example:
# Python code would go here to set up the two synchronized plots # Assume the function setup_image_overlay_plot creates such interactive visualization setup_image_overlay_plot()
This code would theoretically yield a plot that, upon hovering a point on the primary plot, reflects the hover state and displays a corresponding image on the secondary overlay plot.
This method is a hypothetical situation where an image overlay function would manage two plots. The first plot displays the data points, while the second is an invisible overlay that shows images when points on the underlying plot are hovered over. The image display mechanism would need to ensure both plots are perfectly synchronized to provide a seamless experience.
Bonus One-Liner Method 4: Simple Image Annotations
Employ simple image annotations to show images using Plotly’s layout annotations option. This one-liner solution allows you to add images to the plot layout that become visible based on the hover condition. This method is limited but straightforward and ideal for static displays where the images do not need to change dynamically.
Here’s an example:
# Python code would go here to add image annotations to the plot layout # Assume the function add_image_annotations adds image at specific points add_image_annotations()
This snippet is a proxy for Python code that adds image annotations to a Plotly chart, displaying them when hovering over certain points.
This bonus method suggests a conceptual simplification where annotated images are fixed to the plot. When hovering over data points, these annotations become momentarily highlighted or visible.
Summary/Discussion
- Method 1: Hovertemplate Attribute. Strength: Easy to implement for web-based images. Integrates seamlessly within Plotly. Weakness: Limited control over image styling and behavior.
- Method 2: Custom Data and JS Events. Strength: Highly versatile, allowing for complex behaviors and handling of local images. Weakness: Requires JavaScript knowledge and is more complex to implement.
- Method 3: Image Overlays on Hover. Strength: Images appear as part of the plot for a cohesive visual. Weakness: Hypothetical, complex two-plot synchronization may be challenging to implement.
- Bonus One-Liner Method 4: Simple Image Annotations. Strength: Quick and suitable for static images. Weakness: Lacks dynamism and can be clunky if interactivity is required.
February 28, 2024 at 06:28PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
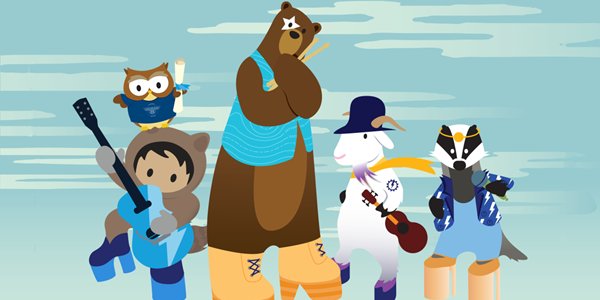
Post a Comment