5 Best Ways to Create a NumPy Array of Zeros in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post

Method 1: Using numpy.zeros()
The numpy.zeros()
function is the most straightforward approach for creating an array filled with zeros. It accepts the shape of the array as a tuple and an optional dtype parameter to specify the data type. The method is efficient and the go-to choice for most applications that require zero initialization.
Here’s an example:
import numpy as np # Creating a 3x4 array of zeros zero_array = np.zeros((3, 4)) print(zero_array)
Output:
[[0. 0. 0. 0.] [0. 0. 0. 0.] [0. 0. 0. 0.]]
In the above snippet, the np.zeros()
function is used to create a 3×4 array of zeros. The print
function then outputs this array to the console.
Method 2: Using numpy.zeros_like()
The numpy.zeros_like()
function creates an array of zeros with the same shape and type as an existing array. It’s particularly useful when you want your zero array to match the specifications of another array you’re working with.
Here’s an example:
import numpy as np # Suppose we have an existing array existing_array = np.array([[1, 2], [3, 4]]) # Now we create an array of zeros with the same shape and type zero_like_array = np.zeros_like(existing_array) print(zero_like_array)
Output:
[[0 0] [0 0]]
The code first defines an existing array existing_array
, and then uses the np.zeros_like()
function to create a new array of the same shape but filled with zeros.
Method 3: Using numpy.full()
Through numpy.full()
, users can create an array filled with any specified value, including zero. This method requires the shape and the fill value, offering a great balance between flexibility and simplicity.
Here’s an example:
import numpy as np # Creating a 2x3 array filled with zeros zero_full_array = np.full((2, 3), 0) print(zero_full_array)
Output:
[[0 0 0] [0 0 0]]
The np.full()
function creates a 2×3 array and fills it with the specified value of zero, efficiently generating the desired zero-filled array.
Method 4: Using numpy.empty() and numpy.fill()
Another technique involves creating an empty array with numpy.empty()
and then filling it with zeros using the fill()
method. This may be more verbose but useful in scenarios where multiple fill values are used over time.
Here’s an example:
import numpy as np # Creating an empty array of a given shape empty_array = np.empty((3, 2)) # Filling the array with zeros empty_array.fill(0) print(empty_array)
Output:
[[0. 0.] [0. 0.] [0. 0.]]
The code first creates an uninitialized array with np.empty()
, and then the fill()
method is called on this array to populate it with zeros.
Bonus One-Liner Method 5: Using List Comprehension and numpy.array()
For the lovers of Python’s list comprehensions, an array of zeros can also be created by combining list comprehension with the numpy.array()
function. This is more of a Pythonic way and might appeal to those familiar with list operations.
Here’s an example:
import numpy as np # Creating a 2D array (3x4) of zeros using list comprehension zero_array_list_comp = np.array([[0 for _ in range(4)] for _ in range(3)]) print(zero_array_list_comp)
Output:
[[0 0 0 0] [0 0 0 0] [0 0 0 0]]
The provided code demonstrates creating a 3×4 array of zeros using list comprehensions to generate the necessary lists before converting them to a NumPy array.
Summary/Discussion
- Method 1: numpy.zeros(). Efficient and straightforward for most use cases. It might not be as flexible when the array needs to match another array’s properties.
- Method 2: numpy.zeros_like(). Best used when an array needs to match the shape and data type of an existing array. It’s a more specific use case compared to method 1.
- Method 3: numpy.full(). Offers great flexibility as it can fill an array with any specified value, not just zeros. It is slightly more verbose for creating zero arrays but pays off when filling with various values is needed.
- Method 4: numpy.empty() and fill(). This method may have a more niche application since it involves two steps, but it’s useful when you need to re-use the allocated array for different fill values.
- Method 5: List Comprehension and numpy.array(). A Pythonic approach that is familiar to those comfortable with list comprehensions. It might not be the most performant for large arrays but is readable and intuitive.
February 20, 2024 at 06:50PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
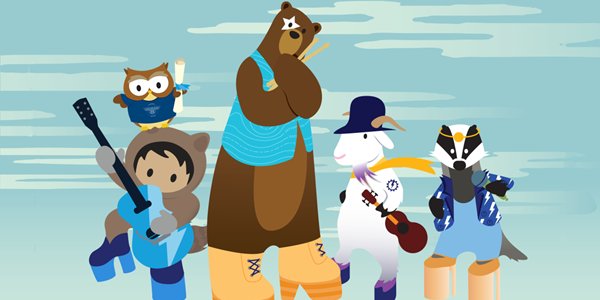
Post a Comment