5 Best Ways to Convert Python Boolean to ‘Yes’ or ‘No’ : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: You have a Python boolean value, and you aim to represent it as a human-readable string, specifically ‘Yes’ for
True
and ‘No’ for False
. This conversion is often needed for user interfaces or reports where clarity of data representation is crucial. For example, you might have a boolean input True
and the desired output is the string ‘Yes’.
Method 1: Using the Ternary Operator
The ternary operator in Python is a concise way to perform an if-else check within a single line of code. The function’s specification is to take a boolean value and return ‘Yes’ if the value is true and ‘No’ if the value is false. It’s an inline method for simple decisions.
Here’s an example:
bool_value = True result = 'Yes' if bool_value else 'No' print(result)
Output: Yes
This code assigns the string ‘Yes’ to the variable result
if bool_value
is True
, and ‘No’ otherwise. The ternary operator provides a compact way to perform this operation.
Method 2: Using a Function
Creating a dedicated function to handle the conversion can encapsulate the logic and make the code more reusable. The function accepts a boolean argument and returns the corresponding string.
Here’s an example:
def bool_to_yes_no(value): return 'Yes' if value else 'No' print(bool_to_yes_no(False))
Output: No
This code snippet defines a function bool_to_yes_no
which uses the same logic as the ternary operator approach but improves code reusability and readability.
Method 3: Using a Dictionary
A dictionary provides a map between the boolean values and their corresponding string representations. This method is straightforward and highly readable.
Here’s an example:
conversion_dict = {True: 'Yes', False: 'No'} bool_value = False result = conversion_dict[bool_value] print(result)
Output: No
This snippet uses a dictionary to map True
and False
to their respective outputs. It looks up the value of bool_value
in the dictionary and prints the result.
Method 4: Using the str.format() Method
The str.format()
method can be utilized to format the string output based on the boolean value. This method adds readability and can be easily modified for different representations.
Here’s an example:
bool_value = True result = "{0}".format('Yes' if bool_value else 'No') print(result)
Output: Yes
This code snippet demonstrates the use of str.format()
with conditional expressions to convert a boolean to a ‘Yes’ or ‘No’ string.
Bonus One-Liner Method 5: Using List Indexing
An innovative way to convert a boolean to ‘Yes’ or ‘No’ is by using list indexing where the boolean acts as the index.
Here’s an example:
result = ['No', 'Yes'][True] print(result)
Output: Yes
This one-liner uses a list where the index 0 represents ‘No’ and index 1 represents ‘Yes’. Since True
evaluates to 1, it selects ‘Yes’ from the list.
Summary/Discussion
- Method 1: Ternary Operator. Strengths: It’s concise and explicit. Weaknesses: It’s not as readable for complex logic.
- Method 2: Using a Function. Strengths: Encapsulates the logic, promoting reuse and readability. Weaknesses: Slightly more verbose for one-off conversions.
- Method 3: Using a Dictionary. Strengths: Offers clear mapping, making the code easy to understand and extend. Weaknesses: Overhead of maintaining a dictionary.
- Method 4: Using the str.format() Method. Strengths: Provides a template that is easy to update and format. Weaknesses: Can be too elaborate for simple true/false conversions.
- Bonus Method 5: List Indexing. Strengths: Extremely concise, clever one-liner. Weaknesses: Potentially confusing and less intuitive for new programmers.
February 24, 2024 at 10:23PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
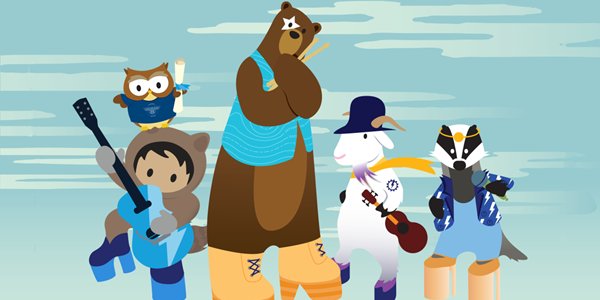
Post a Comment