5 Best Ways to Check If Number Is Even in Python : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Determining the parity of an integer in Python involves checking whether it is divisible by 2 without any remainder. Typically, you might have an integer
n
and you want to get back a Boolean value, True
if n
is even, and False
if it is not. For example, given n = 4
, you want to end up with True
, as 4
is an even number.
Method 1: Using The Modulus Operator
The modulus operator (%
) returns the remainder of a division operation. If a number is divisible by 2 with no remainder, it is even. This method uses the modulus operator to check whether the remainder of the division of a number by 2 is zero.
Here’s an example:
def is_even(num): return num % 2 == 0 print(is_even(10)) # Is 10 an even number? print(is_even(11)) # How about 11?
Output:
True False
In this code snippet, we define a function is_even
that takes a single parameter num
. We return True
if the remainder of num
divided by 2 is 0, which means the number is even. The print statements demonstrate the function in action, returning True
for 10 and False
for 11.
Method 2: Using Bitwise AND Operator
The bitwise AND operator (&
) compares each bit of the number to the corresponding bit of another number. If both bits are 1, it gives 1. Even numbers have their least significant bit as 0. Therefore, performing a bitwise AND operation with 1 can determine if a number is even or odd.
Here’s an example:
def is_even(num): return (num & 1) == 0 print(is_even(42)) # Let's check the answer to life! print(is_even(77)) # What about a lucky number 77?
Output:
True False
The function is_even
here checks if the least significant bit of num
is 0. Since even numbers always have 0 as the least significant bit, num & 1
will be 0 for them. For the number 42, which is even, it returns True
and False
for the odd number 77.
Method 3: Using Division and Multiplication
Another way to check for an even number is to divide the number by 2, multiply the quotient by 2, and then compare it with the original number. If they are the same, the number is even.
Here’s an example:
def is_even(num): return (num // 2) * 2 == num print(is_even(2022)) # A recent even year. print(is_even(1989)) # The year Taylor Swift was born.
Output:
True False
In this code, the is_even
function checks if the result of integer division of num
by 2, when multiplied back by 2, equals num
. This works because integer division by 2 drops the decimal place, effectively ignoring the remainder if it was an odd number. For 2022, which is even, it returns true. It returns false for 1989, which is odd.
Method 4: Using The Float Division
Float division can also be used to determine evenness. By dividing the number by 2 and checking if the result is a whole number, one can infer whether the original number is even. For whole numbers, the mantissa of the floating-point result will be .0
.
Here’s an example:
def is_even(num): return num / 2.0 % 1 == 0 print(is_even(64)) # Can this power of two confirm? print(is_even(81)) # Maybe a square of an odd number?
Output:
True False
By dividing the number by 2.0 (to enforce float division), the is_even
function then checks if there’s any remainder when dividing the result by 1. If there’s none (remainder is 0
), then the number must be even, since it’s fully divisible by 2. This test validates that 64 is even and 81 is not.
Bonus One-Liner Method 5: Using Lambda
For those who appreciate brevity, here’s a one-liner lambda function that performs the check using the modulus operator.
Here’s an example:
is_even = lambda num: num % 2 == 0 print(is_even(2)) # The smallest even prime. print(is_even(3)) # And its odd counterpart.
Output:
True False
This compact version utilizes a lambda function to perform the same operation as in Method 1. It remains concise and to the point, ideal for quick inline use without defining a full function.
Summary/Discussion
- Method 1: Modulus Operator. Strengths: Intuitive and straightforward to understand. Weaknesses: Possibly less efficient than bit manipulation.
- Method 2: Bitwise AND. Strengths: Fast operation at the bit level. Weaknesses: Less readable for those who are not familiar with bitwise operations.
- Method 3: Division and Multiplication. Strengths: Avoids the use of modulus, potentially offering performance benefits. Weaknesses: More arithmetic operations which may not be as efficient as other methods.
- Method 4: Float Division. Strengths: Offers a different approach that is clear in intent. Weaknesses: Involves floating-point arithmetic, which may be slower and could introduce floating-point precision issues.
- Method 5: Lambda One-Liner. Strengths: Very concise, ideal for quick functional programming usage. Weaknesses: Lambda functions can be less accessible to those less experienced with Python, potentially impacting readability.
11 Ways to Create a List of Even Numbers in Python
February 13, 2024 at 05:36PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
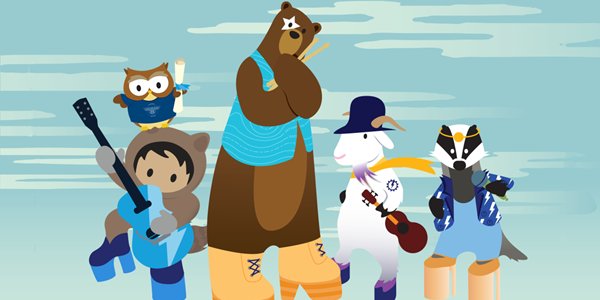
Post a Comment