Python's Array: Working With Numeric Data Efficiently :
by:
blow post content copied from Real Python
click here to view original post
When you start your programming adventure, one of the most fundamental concepts that you encounter early on is the array. If you’ve recently switched to Python from another programming language, then you might be surprised that arrays are nowhere to be found as a built-in syntactical construct in Python. Instead of arrays, you typically use lists, which are slightly different and more flexible than classic arrays.
That said, Python ships with the lesser-known array
module in its standard library, providing a specialized sequence type that can help you process binary data. Because it’s not as widely used or well documented as other sequences, there are many misconceptions surrounding the use of the array
module. After reading this tutorial, you’ll have a clear idea of when to use Python’s array
module and the corresponding data type that it provides.
In this tutorial, you’ll learn how to:
- Create homogeneous arrays of numbers in Python
- Modify numeric arrays just like any other sequence
- Convert between arrays and other data types
- Choose the right type code for Python arrays
- Emulate nonstandard types in arrays
- Pass a Python array’s pointer to a C function
Before you dive in, you may want to brush up on your knowledge of manipulating Python sequences like lists and tuples, defining custom classes and data classes, and working with files. Ideally, you should be familiar with bitwise operators and be able to handle binary data in Python.
You can download the complete source code and other resources mentioned in this tutorial by clicking the link below:
Get Your Code: Click here to download the free source code that shows you how to use Python’s array
with your numeric data.
Understanding Arrays in Programming
Some developers treat arrays and Python’s lists as synonymous. Others argue that Python doesn’t have traditional arrays, as seen in languages like C, C++, or Java. In this brief section, you’ll try to answer whether Python has arrays.
Arrays in Computer Science
To understand arrays better, it helps to zoom out a bit and look at them through the lens of theory. This will clarify some baseline terminology, including:
- Abstract data types
- Data structures
- Data types
Computer science models collections of data as abstract data types (ADTs) that support certain operations like insertion or deletion of elements. These operations must satisfy additional constraints that describe the abstract data type’s unique behaviors.
The word abstract in this context means these data types leave the implementation details up to you, only defining the expected semantics or the set of available operations that an ADT must support. As a result, you can often represent one abstract data type using a few alternative data structures, which are concrete implementations of the same conceptual approach to organizing data.
Programming languages usually provide a few data structures in the form of built-in data types as a convenience so that you don’t have to implement them yourself. This means you can focus on solving more abstract problems instead of starting from scratch every time. For example, the Python dict
data type is a hash table data structure that implements the dictionary abstract data type.
To reiterate the meaning of these terms, abstract data types define the desired semantics, data structures implement them, and data types represent data structures in programming languages as built-in syntactic constructs.
Some of the most common examples of abstract data types include these:
In some cases, you can build more specific kinds of abstract data types on top of existing ADTs by incorporating additional constraints. For instance, you can build a stack by modifying the queue or the other way around.
As you can see, the list of ADTs doesn’t include arrays. That’s because the array is a specific data structure representing the list abstract data type. The list ADT dictates what operations the array must support and which behaviors it should exhibit. If you’ve worked with the Python list
, then you should already have a pretty good idea of what the list in computer science is all about.
Note: Don’t confuse the list abstract data type in computer science with the list
data type in Python, which represents the former. Similarly, it’s easy to mistake the theoretical array data structure for a specific array data type, which many programming languages provide as a convenient primitive type built into their syntax.
The list abstract data type is a linear collection of values forming an ordered sequence of elements. These elements follow a specific arrangement, meaning that each element has a position relative to the others, identified by a numeric index that usually starts at zero. The list has a variable but finite length. It may or may not contain values of different types, as well as duplicates.
The interface of the list abstract data type resembles Python’s list
, typically including the following operations:
List ADT | Python’s list |
---|---|
Get an element by an index | fruits[0] |
Set an element at a given index | fruits[0] = "banana" |
Insert an element at a given index | fruits.insert(0, "banana") |
Delete an element by an index | fruits.pop(0) , del fruits[0] |
Delete an element by a value | fruits.remove("banana") |
Delete all elements | fruits.clear() |
Find the index of a given element | fruits.index("banana") |
Append an element at the right end | fruits.append("banana") |
Merge with another list | fruits.extend(veggies) , fruits + veggies |
Sort elements | fruits.sort() |
Get the number of elements | len(fruits) |
Iterate over the elements | iter(fruits) |
Check if an element is present | "banana" in fruits |
Now that you understand where the array data structure fits into the bigger picture, it’s time to take a closer look at it.
Read the full article at https://realpython.com/python-array/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
January 01, 2024 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
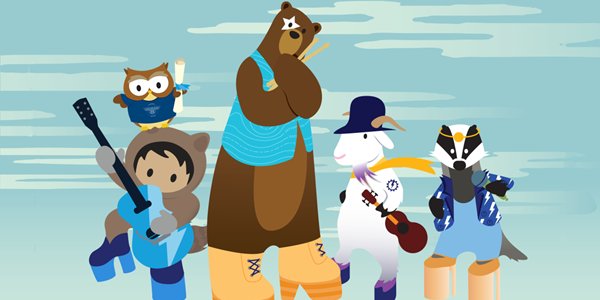
Post a Comment