Python Read Text File into List of Strings : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
Challenge: Reading text files into lists is a common task in Python programming, especially when processing or manipulating each line of text in a file. This article demonstrates various methods to efficiently read a text file into a list of strings, where each element of the list corresponds to a line in the file.
Method 1: Using readlines()
The readlines()
method is a straightforward approach to reading a text file into a list of strings in Python. It reads until EOF using readline()
and returns a list containing the lines.
with open('example.txt', 'r') as file: lines = file.readlines()
The with
statement handles the opening and closing of the file. The readlines()
method reads all the lines from the file and stores them as a list in the variable lines
, where each line ends with a newline character.
Method 2: Using List Comprehension with readline()
List comprehension combined with readline()
provides a more explicit control over reading lines and can be used to modify the lines as they are read.
with open('example.txt', 'r') as file: lines = [line.strip() for line in file]
We utilize list comprehension to iterate over the file object, which yields lines of the file.
The strip()
method is called on each line to remove the newline characters at the end of each line before storing them in the lines
list. This method affords an opportunity to process the strings during file reading.
Method 3: Using read().splitlines()
Another approach is to read the entire content of the file using read()
and then split it into a list of strings using splitlines()
.
with open('example.txt', 'r') as file: lines = file.read().splitlines()
This method opens the file, reads its entire content into a single string, and then uses the splitlines()
method to split the string into a list, where each element is a line from the text file, excluding the newline characters.
Method 4: Using read().split()
For files that contain a list of words or strings separated by whitespace rather than newline characters, you can use read()
followed by split()
to create your list.
with open('example.txt', 'r') as file: words = file.read().split()
In this snippet, the read()
method reads the entire content of the file into a single string, and then split()
is used to divide the string into a list, based on whitespace. Each element of the list is a word from the file.
Summary/Discussion
These methods provide different ways to read a text file into a list of strings in Python. Choosing the right method depends on whether you need to remove newline characters or process lines as they are read.
- The
readlines()
method is the simplest, but list comprehension gives you more control over the input and output. read().splitlines()
is suitable when you want to avoid newline characters in the result, andread().split()
is best for reading words or delimited strings.
It’s important to handle files carefully and ensure they are properly closed after reading to avoid any resource leaks, which the with
statement helps manage effectively.
January 22, 2024 at 02:25AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
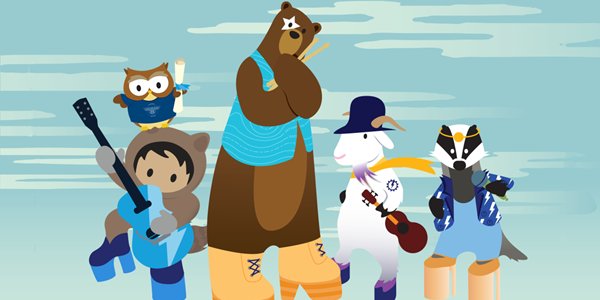
Post a Comment