Python List of Strings to One String : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
Question: How do you convert or flatten a list of strings to a single string with and without a separator?
Here are five examples:
- No Separator
- Input:
["Hello", "World"]
- Output:
"HelloWorld"
- Input:
- Space Separator
- Input:
["Hello", "World"]
- Output:
"Hello World"
- Input:
- Comma Separator
- Input:
["apple", "banana", "cherry"]
- Output:
"apple,banana,cherry"
- Input:
- Newline Separator
- Input:
["line1", "line2", "line3"]
- Output:
"line1\nline2\nline3"
- Input:
- Hyphen Separator
- Input:
["1", "2", "3", "4", "5"]
- Output:
"1-2-3-4-5"
- Input:
In each case, the .join()
method can be used in Python with the specified separator to achieve the desired output.
Method 1: Using the join() Method
The join()
method is a straightforward and efficient way to concatenate a list of strings. It allows you to specify a separator, which can be any string, including an empty string, to join the elements in the list.
fruits = ["apple", "banana", "cherry"] result = " ".join(fruits) print(result)
This code concatenates the strings in the fruits
list, separated by a space. The output will be 'apple banana cherry'
.

Python Converting List of Strings to * [Ultimate Guide]
Method 2: Using a For Loop
Iterating over the list with a for
loop and concatenating each element to a new string. This method offers more control over the concatenation process.
fruits = ["apple", "banana", "cherry"] result = "" for fruit in fruits: result += fruit + " " print(result.strip())
The code iterates through each string in fruits
, adding them to result
with a space. The strip()
function is used to remove the trailing space.
Method 3: Using List Comprehension
This method involves using list comprehension to create a new list of strings and then joining them. It’s a more concise version of the for
loop approach but really only a trivial variation of the first method. Not recommended!
fruits = ["apple", "banana", "cherry"] result = " ".join([fruit for fruit in fruits]) print(result)
The list comprehension [fruit for fruit in fruits]
creates a new list identical to fruits
, and join()
concatenates them with a space.
Method 4: Using the map() Function
The map()
function applies a given function to each item of an iterable (like a list) and returns a list of the results. When combined with join()
, it can be used for string concatenation.
fruits = ["apple", "banana", "cherry"] result = " ".join(map(str, fruits)) print(result)
The map(str, fruits)
converts each element in fruits
to a string (if not already), and join()
concatenates them.
Method 5: Using reduce() from functools
The reduce()
function, which is part of the functools
module, applies a function cumulatively to the items of a list, from left to right, so as to reduce the list to a single value.
from functools import reduce fruits = ["apple", "banana", "cherry"] result = reduce(lambda a, b: a + " " + b, fruits) print(result)
The reduce()
function applies the lambda function cumulatively to the elements of fruits
, concatenating them with a space.
Comparison and Tips
- Efficiency:
join()
is generally the most efficient method, especially for large lists. - Flexibility: Loops and list comprehension offer more control over the concatenation process.
- Simplicity:
join()
andmap()
are more readable and concise. - Use Case: Use
join()
for simple concatenations; loops or list comprehension for more complex conditions;map()
for applying a function to elements; andreduce()
for cumulative concatenations.
Related Video

What’s the most Pythonic way to join a list of objects?
January 15, 2024 at 03:31AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
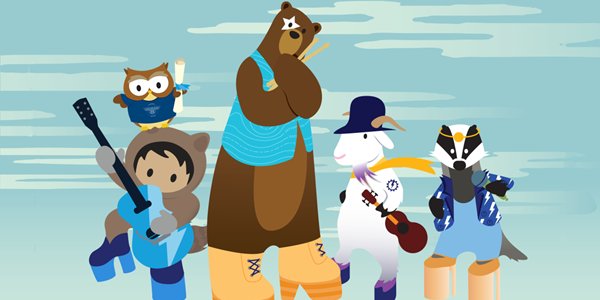
Post a Comment