3 Best Ways to Read Text File Into List Without Newline in Python : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
When reading a text file into a list using Python, one common problem is that the lines are often terminated with newline (\n
) characters. This can result in a list where each element ends with a newline character.
You may want a clean list without these newline characters at the end of each string.
Let’s explore several methods to read a text file into a list and strip out newline characters.
Method 1: Using readlines() and Stripping Newlines
One basic method involves using the readlines()
function which reads all the lines in a file and then we can use the strip()
method to remove newline characters from the end of each line. This process is usually done with a for-loop or a list comprehension.
Here’s a simple example:
with open('file.txt', 'r') as file: lines = [line.strip() for line in file.readlines()]
The with
keyword is used to open the file and ensure proper closure after its block is executed. file.readlines()
reads all the lines into a list, and line.strip()
is used to remove leading/trailing whitespace, including newline characters, from each line.
Method 2: Using read().splitlines()
Another way to accomplish this is by reading the entire file content as a single string using read()
, and then splitting it into a list of lines without newlines using splitlines()
.
Here’s a simple example:
with open('file.txt', 'r') as file: lines = file.read().splitlines()
Here, file.read()
returns the entire file contents. splitlines()
then splits the string into a list where each element is a line from the input, sans newline characters.
Method 3: Using a File Iterator with Strip
This method involves iterating over the file object directly (which is also an iterator) and stripping the newline characters from each line.
Here’s a simple example:
with open('file.txt', 'r') as file: lines = [line.strip() for line in file]
This concise approach reads lines lazily (which is memory efficient for large files) and applies strip()
to each line in a list comprehension.
Summary/Discussion
Python offers various ways to read a file and create a list of lines without including newline characters.
readlines()
combined withstrip()
is straightforward and commonly used, whileread().splitlines()
is a bit more succinct.- Iterating directly over the file is memory efficient for large files.
The choice of method may depend on your file size and whether you want to strip other whitespace characters or only newlines.
January 22, 2024 at 02:17AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
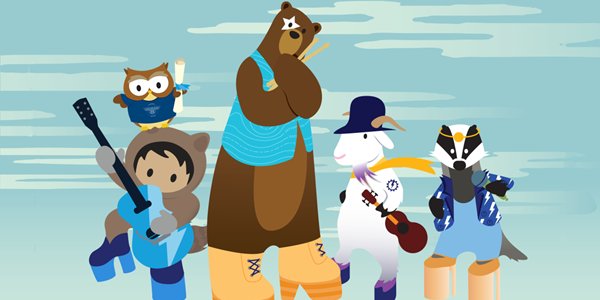
Post a Comment