Python Ternary in List Comprehension : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
List comprehensions are a powerful feature in Python, allowing you to create new lists by specifying their elements with a concise and readable syntax. One of the ways to make your list comprehensions more expressive and flexible is by incorporating ternary operators, also known as conditional expressions.
A ternary operator in a Python list comprehension allows you to include if-else expressions directly within the statement. This brings the advantage of having a more concise code, while still maintaining readability.
The use of ternary operators in list comprehensions is considered a pythonic way to achieve conditional logic within a single line of code.
Tip: As your list comprehensions grow more complex with the addition of ternary operators, they can become difficult to read and understand. To maintain the clarity of your code, it’s a good idea to only use ternary operators in list comprehensions when they significantly improve the conciseness and readability of your code, while still communicating the purpose of the list comprehension effectively.
Understanding Python Ternary in List Comprehension

Ternary Operator and its Usage
The ternary operator is a simple yet powerful concept in programming, particularly in Python. It allows you to write a concise expression for selecting one value based on a condition. The syntax for the ternary operator in Python is: value_if_true if condition else value_if_false
.
For example, you can use the ternary operator to assign a string "even"
or "odd"
depending on the remainder of a number when divided by 2:
number = 5 result = "even" if number % 2 == 0 else "odd" print(result) # Output: "odd"
List Comprehensions and their Advantages
List comprehensions are a powerful and concise way to generate lists in Python. They are used to replace traditional for loops, which can sometimes be cumbersome and harder to read, especially for operations like filtering and mapping.
List comprehensions use a compact syntax, which involves a single line of code.
You can create a list comprehension using the following format:
[expression for variable in iterable if condition]
Here is an example of a list comprehension that squares all even numbers in a given range:
even_squares = [x**2 for x in range(1, 11) if x % 2 == 0] print(even_squares) # Output: [4, 16, 36, 64, 100]
List comprehensions offer several advantages, such as improved readability, better performance, and the ability to generate numerous data structures, including lists, sets, dictionaries, and tuples concisely.
Combining the Two for Efficient Coding
By combining the ternary operator and list comprehensions, you can accomplish complex tasks in a concise and efficient manner. This technique can help you condense multiple lines of code into a single, easy-to-understand line while maintaining the same functionality.
For instance, you can create a list of strings indicating whether the numbers in a given range are even or odd using the ternary operator within a list comprehension:
even_or_odd = ["even" if i % 2 == 0 else "odd" for i in range(1, 11)] print(even_or_odd) # Output: ['odd', 'even', 'odd', 'even', 'odd', 'even', 'odd', 'even', 'odd', 'even']
Frequently Asked Questions

How does boolean work in list comprehension?
In list comprehension, you can use boolean expressions to filter or manipulate data. By incorporating a conditional statement, you can determine which elements from the original list should be included in the new list. For example, to create a list of even numbers from a given list, you can use even_numbers = [x for x in original_list if x % 2 == 0]
. The boolean expression, x % 2 == 0
, filters out odd numbers.
How to use if-else in one line list comprehension?
If-else statements can be used in list comprehension to apply different operations to elements based on a condition. The syntax is [expression_if_true if condition else expression_if_false for element in iterable]
. For instance, to create a list with “even” and “odd” strings based on numbers, use ['even' if x % 2 == 0 else 'odd' for x in original_list]
.
Can we use functions within list comprehensions?
Yes, you can use functions within list comprehensions. You can call a function to process each element of an iterable or use it as part of the condition. For example, to apply the square
function to all numbers in a list, use [square(x) for x in original_list]
. Similarly, to filter elements based on a custom condition, such as a is_prime(x)
function, use [x for x in original_list if is_prime(x)]
.
How to filter items with multiple conditions in list comprehension?
You can filter items using multiple conditions by combining boolean expressions with logical operators, such as and
or or
. For example, to create a list of all even numbers greater than 10 from an existing list, use [x for x in original_list if x % 2 == 0 and x > 10]
. To include items that meet at least one condition, use or
: [x for x in original_list if x % 2 == 0 or x > 10]
.
Can list comprehension stop at first match?
List comprehension doesn’t have a built-in way to stop at the first match. However, you can use the next()
function with a generator expression to achieve this. The syntax is next((element for element in iterable if condition), default_value)
. For example, to find the first even number in a list, use first_even = next((x for x in original_list if x % 2 == 0), None)
.
How to perform summation of elements in list comprehension?
While you can create a list of elements with list comprehension, it’s more efficient to use the sum()
function and a generator expression for summations. The syntax is total = sum(element for element in iterable if condition)
. For instance, to calculate the sum of all even numbers in a list, use sum_of_evens = sum(x for x in original_list if x % 2 == 0)
. This avoids creating an intermediary list and directly computes the total.
Recommended: Python One Line X
December 16, 2023 at 12:44AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
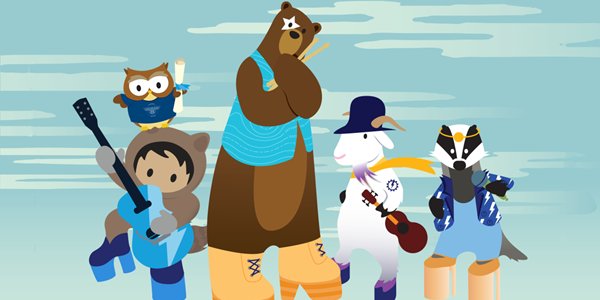
Post a Comment