Yahoo-Fin: Fetching Historical Stock Data with Python’s Yahoo Finance API : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
This guide provides an easy-to-understand foundation for beginners and intermediate users to leverage the Yahoo Finance API with Python for financial data analysis.
Yahoo Finance API offers a wealth of financial data, from stock prices and market trends to currency exchange rates. This guide will introduce you to using the Yahoo Finance API yahoo_fin
with Python, a powerful combination for financial data analysis.
Quick Info: The Python library
yahoo_fin
is not well maintained but works for quick, dirty, and simple access without requiring you to create an API key. It provides free access to a wide range of financial data.
Setting Up Your Environment
Before diving into the API, ensure Python is installed on your system. Python 3.x is recommended for its latest features and support.
Recommended: How to Install Python?
You’ll primarily need yahoo_fin
, a Python library that simplifies accessing Yahoo Finance data. Install it using pip:
pip install yahoo_fin
Understanding the Yahoo_fin Library
yahoo_fin
offers two main modules:
stock_info
: For stock data.options
: For options data.
These modules provide functions to access various data types like historical prices, stock information, etc.
Fetching Historical Data with Yahoo_fin
The get_data()
function from stock_info
module is used to fetch historical price data for a given stock. Here’s how you use it:
from yahoo_fin.stock_info import get_data # Fetch historical data for Apple apple_data = get_data('AAPL') print(apple_data)
Output:

For real-time data, use the get_live_price()
function:
from yahoo_fin.stock_info import get_live_price # Get the current price of Tesla stock tesla_live_price = get_live_price('TSLA') print(tesla_live_price) # 234.3000030517578
Example: Analyzing Stock Performance
Python’s data analysis libraries, like Pandas, can be used in conjunction with yahoo_fin
to perform more complex analyses.
import pandas as pd from yahoo_fin.stock_info import get_data # Fetch historical data for a stock stock_data = get_data('AAPL', start_date='01/01/2020', end_date='01/01/2021') # Convert to a Pandas DataFrame for analysis df = pd.DataFrame(stock_data) print(df)
Output:

Visualizing Data
With libraries like Matplotlib or Seaborn, you can visualize financial data:
import matplotlib.pyplot as plt # Plotting the closing price of Apple stock plt.figure(figsize=(10, 5)) plt.plot(df['close'], label='Apple Close Price') plt.title('Apple Stock Close Price (2020)') plt.xlabel('Date') plt.ylabel('Price (USD)') plt.legend() plt.show()
This is how this looks at the time of writing (using the df
variable defined in the previous step):
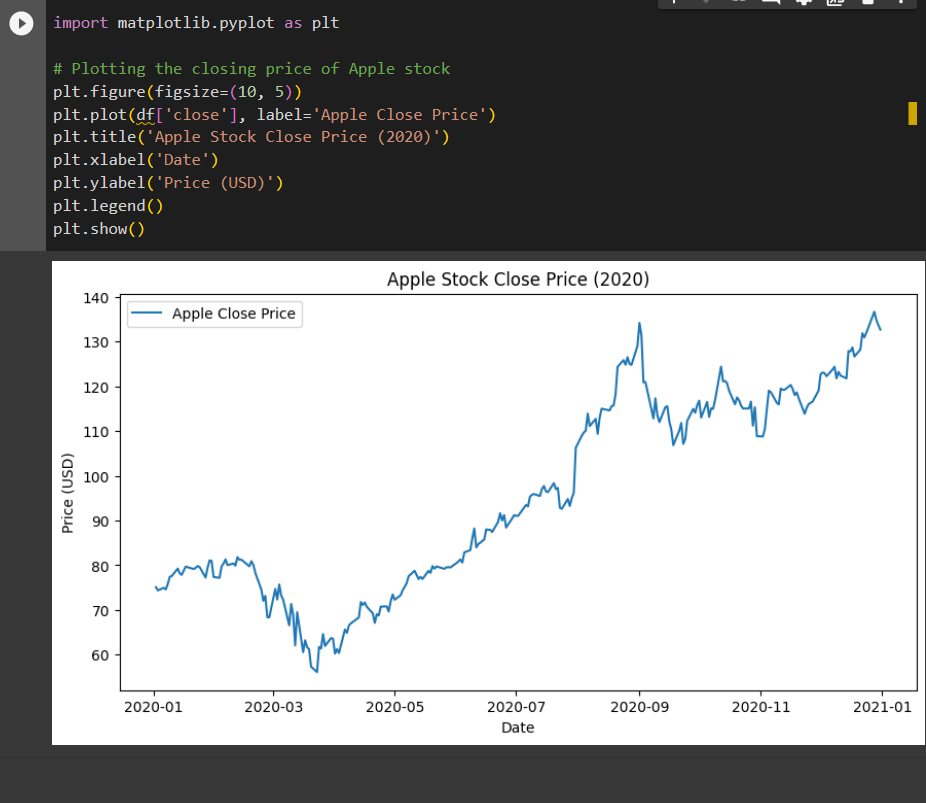
Check out our email newsletter with free cheat sheets to keep learning and improving your skills:
November 18, 2023 at 04:23PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
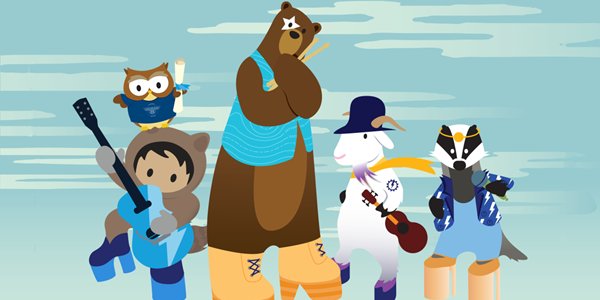
Post a Comment