Python's F-String for String Interpolation and Formatting
by:
blow post content copied from Real Python
click here to view original post
Python f-strings provide a quick way to interpolate and format strings. They’re readable, concise, and less prone to error than traditional string interpolation and formatting tools, such as the .format()
method and the modulo operator (%
). An f-string is also a bit faster than those tools!
By the end of this tutorial, you’ll know why f-strings are such a powerful tool that you should learn and master as a Python developer.
In this tutorial, you’ll learn how to:
- Interpolate values, objects, and expressions into your strings using f-strings
- Format f-strings using Python’s string formatting mini-language
- Leverage some cool features of f-strings in Python 3.12 and beyond
- Decide when to use traditional interpolation tools instead of f-strings
To get the most out of this tutorial, you should be familiar with Python’s string data type. It’s also be beneficial to have experience with other string interpolation tools like the modulo operator (%
) and the .format()
method.
Get Your Code: Click here to download the free sample code that shows you how to do string interpolation and formatting with Python’s f-strings.
Interpolating and Formatting Strings Before Python 3.6
Before Python 3.6, you had two main tools for interpolating values, variables, and expressions inside string literals:
- The string interpolation operator (
%
), or modulo operator - The
str.format()
method
You’ll get a refresher on these two string interpolation tools in the following sections. You’ll also learn about the string formatting capabilities that these tools offer in Python.
The Modulo Operator, %
The modulo operator (%
) was the first tool for string interpolation and formatting in Python and has been in the language since the beginning. Here’s what using this operator looks like in practice:
>>> name = "Jane"
>>> "Hello, %s!" % name
'Hello, Jane!'
In this quick example, you use the %
operator to interpolate the value of your name
variable into a string literal. The interpolation operator takes two operands:
- A string literal containing one or more conversion specifiers
- The object or objects that you’re interpolating into the string literal
The conversion specifiers work as replacement fields. In the above example, you use the %s
combination of characters as a conversion specifier. The %
symbol marks the start of the specifier, while the s
letter is the conversion type and tells the operator that you want to convert the input object into a string.
If you want to insert more than one object into your target string, then you can use a tuple. Note that the number of objects in the tuple must match the number of format specifiers in the string:
>>> name = "Jane"
>>> age = 25
>>> "Hello, %s! You're %s years old." % (name, age)
'Hello, Jane! You're 25 years old.'
In this example, you use a tuple of values as the right-hand operand to %
. Note that you’ve used a string and an integer. Because you use the %s
specifier, Python converts both objects to strings.
You can also use dictionaries as the right-hand operand in your interpolation expressions. To do this, you need to create conversion specifiers that enclose key names in parentheses:
>>> "Hello, %(name)s! You're %(age)s years old." % {"name": "Jane", "age": 25}
"Hello, Jane! You're 25 years old."
This syntax provides a readable approach to string interpolation with the %
operator. You can use descriptive key names instead of relying on the positional order of values.
When you use the %
operator for string interpolation, you can use conversion specifiers. They provide some string formatting capabilities that take advantage of conversion types, conversion flags, and some characters like the period (.
) and the asterisk (*
). Consider the following example:
>>> "Balance: $%.2f" % 5425.9292
'Balance: $5425.93'
>>> print("Name: %s\nAge: %5s" % ("John", 35))
Name: John
Age: 35
In the first example, you use the %.2f
conversion specifier to represent currency values. The f
letter tells the operator to convert to a floating-point number. The .2
part defines the precision to use when converting the input. In the second example, you use %5s
to align the age value five positions to the right.
Read the full article at https://realpython.com/python-f-strings/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
October 18, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
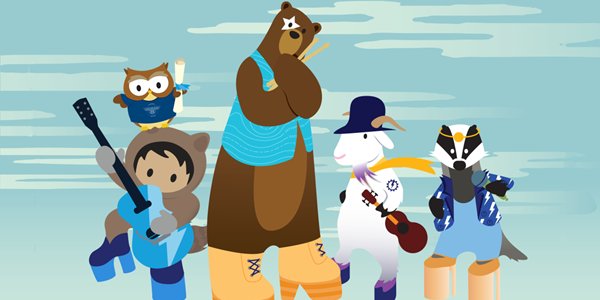
Post a Comment