Python to EXE with All Dependencies : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Developing a Python application can be a rewarding experience, but sharing your creation with others might seem daunting, especially if your users are not familiar with Python environments.
One solution to this dilemma is converting your Python script into an executable (.exe
) file with all its dependencies included, making it simple for others to run your application on their Windows machines without needing to install Python or manage packages.
Understanding EXE and Dependencies
When working with Python, you may want to create an executable file that can run on systems that do not have Python installed. This process involves converting your .py
file to a Windows executable, or .exe
, format. Additionally, it’s essential to include all dependencies to ensure that your program will run smoothly on any computer.
An executable file, or simply an executable, allows users to run your program without needing to worry about installing the appropriate version of Python or any other necessary libraries. A standalone executable can be especially helpful when distributing your application, as it eliminates the need for end-users to install additional components.
In Python, creating an executable with all dependencies means that the resulting file will have everything it needs for your program to function correctly.
Dependencies are external libraries or modules that your program relies on to function. For instance, if your Python script uses the requests
library to make HTTP calls, your program won’t work on a system that does not have this library installed. Including all dependencies in your executable ensures that your users won’t encounter errors due to missing components.
To convert your Python script into an executable with all dependencies, you can use tools like PyInstaller. PyInstaller bundles your Python script and its dependencies into a single file or folder, making it easier to distribute your application. Once you have generated your .exe
file, anyone with a Windows system will be able to run your program without needing to install Python or additional libraries.
Keep in mind that when creating an executable with dependencies, the resulting file might be larger than the original script. This is because all the necessary libraries are bundled directly within the .exe
. However, this is a small price to pay for the convenience of a standalone application that users can run without additional setup.
Yes, PyInstaller Works for All Operating Systems
When developing and distributing Python applications, it is critical to consider the operating system (OS) you are working on. Python runs on various OS like Windows, macOS, and Linux. Each OS handles application distribution and dependencies differently, so understanding their nuances is essential for successful Python-to-EXE conversions.
On Windows, the most popular method to package your Python application is by using PyInstaller. It helps convert your script into an executable file, bundling all the required dependencies. Users can easily run the resulting file without additional installations. This tool also provides support for other OS such as macOS and Linux.
macOS users can use the same PyInstaller mentioned above, following a similar process as they would on Windows. However, it’s crucial to note that the created executable file will be specific to macOS and not compatible with other OS without re-compilation. In other words, make sure to create separate executable files for each target OS.
For Linux systems, again, PyInstaller is an excellent choice. The usage and dependency bundling process is akin to Windows and macOS, ensuring a smooth experience for your application users. Keep in mind the Python interpreter included in the bundle will be specific to the OS and the word size.
5 Best Tools for Creating an Executable in Python
When working with Python projects, you may need to convert your scripts into executable files with all dependencies included. There are several tools available for this purpose, each with its unique features and advantages. This section will briefly introduce you to five popular tools: PyInstaller, py2exe, cx_Freeze, Nuitka, and auto-py-to-exe.
Python to Exe 1: PyInstaller is a popular choice due to its ease of use and extensive documentation. To install it, simply run pip install pyinstaller
. Once installed, you can create an executable using the command pyinstaller yourprogram.py
. For a single-file executable, you can use the --onefile
flag, as in pyinstaller --onefile yourprogram.py
.
Python to Exe 2: py2exe is another option, primarily used for Windows. It requires a slightly more involved setup, as you’ll need to create a setup.py
script to configure your project. However, it gives you more control over the final executable’s configuration. To use py2exe, first install it using pip install py2exe
, then create your setup.py
and execute it with Python to generate the EXE file.
Python to Exe 3: cx_Freeze is a cross-platform tool that works with both Python 2 and Python 3. It also utilizes a configuration script (setup.py
) and is installed with pip install cx_Freeze
. After installation, you can create an executable by executing the setup.py
file with Python.
Python to Exe 4: Nuitka is unique in that it compiles your Python code into C++ for increased performance. This can be beneficial for resource-intensive applications or when speed is critical. Installation is done via pip install Nuitka
. To create your executable, use the command nuitka --recurse-all --standalone yourprogram.py
.
Python to Exe 5: Finally, auto-py-to-exe provides a graphical user interface for PyInstaller, simplifying the process for those who prefer a more visual approach. Install it with pip install auto-py-to-exe
, then run the command auto-py-to-exe
to launch the GUI.
Package and Module Management
When working with Python, managing packages and modules is crucial to ensure your code runs smoothly and efficiently. Python provides a powerful package manager called pip, which allows you to install, update, and remove packages necessary for your project. As you build your Python application, it is essential to keep track of the dependencies and their versions, often stored in a requirements.txt
file.
To manage your dependencies more effectively, it is recommended to use a dependency manager like Pipenv, which simplifies the process for collaborative projects by automatically creating and managing a virtual environment and a Pipfile
. This tool provides an improved and higher-level workflow compared to using pip and requirements.txt
.
Python modules are files containing Python code, usually with a .py
extension. They define functions, classes, and variables that can be utilized in other Python scripts. Modules are organized in site-packages
– the directory where third-party libraries and packages are installed. A well-structured codebase will make use of these modules, organizing related functionalities and allowing for easy maintenance and updates.
When you need to package your Python project into a standalone executable, tools like PyInstaller can make the process easier. This application packages Python programs into stand-alone executables, compatible with Windows, macOS, Linux, and other platforms. It includes all dependencies and required files within the executable, ensuring your program can be distributed and run on machines without Python installed.
To provide a smooth experience for users, it is crucial to properly manage hidden imports. Hidden imports are modules that PyInstaller may not automatically detect when bundling your application. To ensure these modules are included, you can modify the PyInstaller command using the --hidden-import
option or list the hidden imports in your PyInstaller spec file.
By mastering package and module management in your Python projects, you’ll ensure that your applications run efficiently and are easily maintainable. Utilizing the proper tools and following best practices will enable you to manage dependencies seamlessly and create robust, standalone executables.
Setting Up the Environment
Before diving into converting your Python code into an executable with all dependencies, it’s important to set up a proper development environment. This allows for a smooth workflow and ensures that your application runs correctly.
First, you should have a solid text editor or integrated development environment (IDE) for writing your Python code. There are many popular text editors such as Visual Studio Code, Sublime Text, or PyCharm that can help you with this.
Next, you will need to install Python on your machine. Visit the official Python website and download the appropriate version for your operating system. Make sure to add Python to your system PATH
during the installation process, so it is accessible from the command prompt or terminal.
Once Python is installed, it’s a good idea to create a virtual environment for your project. This isolates your project’s dependencies from other Python projects on your system. To create a virtual environment, you’ll need to install the virtualenv
package with the command:
pip install virtualenv
Then, navigate to your project folder and run the following command to create a new virtual environment:
python -m venv <virtual-environment-name>
Replace <virtual-environment-name>
with an appropriate name for your environment, typically something like env
. To activate your virtual environment, use one of the following commands, depending on your operating system:
- For Windows:
env\Scripts\activate.bat
- For macOS/Linux:
source env/bin/activate
With your virtual environment activated, you can now install the necessary dependencies for your project. Create a setup.py
file in your project folder and define the required packages and their versions. Then, to install the dependencies, simply run:
pip install -r setup.py
All your dependencies should now be installed within your virtual environment and ready to use in your Python code.
Finally, when you’re ready to convert your Python code into an executable, you will generate the executable in a dist
folder. This folder should contain your .exe
file alongside any necessary dependencies, ensuring your application runs smoothly on the target system.
Python to EXE Conversion Process
Converting your Python code to an executable file with all its dependencies can be a simple and straightforward process. When you want to create a standalone executable from your Python application, you can use a tool like PyInstaller that packages your code and its dependencies into a single EXE file.
First, you need to install PyInstaller by running the following command in your command prompt or terminal:
pip install pyinstaller
After installing PyInstaller, navigate to your Python script directory using the cd
command. Then use the following command to create an executable:
pyinstaller yourprogram.py
This command compiles your Python code and bundles the required dependencies into the executable. The result will be a folder named dist
in the same directory as your Python script, containing the EXE file alongside the necessary libraries.
To customize the output executable, such as specifying an icon or including additional data files, you can create a spec
file. A spec file contains configuration options that dictate how PyInstaller packages your Python application. To create a spec file, use the following command:
pyinstaller --onefile --specpath your_spec_directory -i your_icon.ico yourprogram.py
This command generates a spec file in the specified directory, with the provided icon for the executable. You can then edit the spec file to include additional settings, such as the NSIS installer options or custom hooks for including third-party libraries.
Once you’ve configured your spec file, you can use it to create the final executable by running:
pyinstaller yourprogram.spec
Potential Errors and Testing
When converting Python scripts to executable files along with all their dependencies, you may encounter various errors during the process. To help you prevent, identify, and resolve these issues, here are some pointers on potential errors and testing strategies.
One common error you might face is missing dependencies when converting your .py
file to a .exe
file. To avoid this, ensure that all required packages and libraries are installed and properly functioning before initiating the conversion process. Use tools like pyinstaller to help package your script with necessary dependencies.
Runtime errors are another category of issues that might emerge after creating your executable. Since the executable will often be run on machines without Python, you need to verify that the generated binary works correctly. Test your newly created .exe
file on various systems to catch and resolve any compatibility issues. Remember to cover different operating systems, system architectures, and hardware configurations in your testing process.
Note: As you work with large dependencies such as OpenCV, BeautifulSoup4, and Selenium, bear in mind that the size of the final executable might increase significantly. This might lead to longer load times for your application, possible memory issues, and challenges when distributing the executable to users. Optimize your code and dependencies where possible, and consider using compression tools to reduce the file size.
Finally, it’s important to conduct thorough testing to ensure that your executable works as expected. Carry out end-to-end testing on different systems and evaluate every aspect of the application’s functionality.
Additionally, perform performance testing to measure the application’s responsiveness and resource usage, allowing you to pinpoint any bottlenecks and optimization opportunities.
If your Python EXE doesn’t work, everybody will hate your app.
Distribution and Documentation
When it comes to distributing your Python application as an executable with all its dependencies, using tools like PyInstaller can greatly simplify the process. This tool helps you create a standalone executable file without the need for end-users to install Python or any other dependencies.
To get started with PyInstaller, you will want to ensure your project is well-organized and conveniently use Python libraries. If you haven’t already, create a virtual environment for your project and install all the necessary dependencies using pip install
. This will make it easier for the tool to package everything your application needs.
Documentation plays a crucial role in making your application easy to use and understand. Be sure to provide clear and concise instructions on using your executable, including any available command-line options or configuration settings. Remember to address any potential issues or common troubleshooting steps that users might encounter.
As you’re writing your documentation, keep in mind that your users may not be experts in Python. Avoid using jargon, and opt for simple, straightforward language to explain any technical aspects of your application. Where possible, provide examples and illustrations to help users visualize the processes.
In addition to written instructions, it’s a good idea to create a repository for your project, including readme files and guides that demonstrate how to set up, run, and modify your program. Platforms like GitHub or GitLab are excellent choices because they allow you to store, manage, and share your project files and documentation easily.
Advanced Python to EXE Techniques
One common issue faced when converting Python applications to EXE files is handling large libraries such as NumPy and Pandas. Using a tool like pyinstaller can help package these dependencies along with your application. Install and use pyinstaller by running:
pip install pyinstaller pyinstaller yourprogram.py
This will generate an executable file with all dependencies in the “dist” folder.
For Python projects that involve a graphical user interface (GUI) application, If using a library like Tkinter or PyQt, ensure that you properly configure the main()
function within your script. This will allow the application to launch properly after being converted into an EXE. Also, consider using a dedicated tool such as auto-py-to-exe that provides a visual interface for packaging your GUI application.
If your Python project relies on C extensions, you might want to look into Cython. It’s a superset of the Python programming language that compiles Python scripts into C, which then can be linked into an executable. Cython can help improve performance and also provide better protection for your source code. Cython can be a suitable option in case you’re considering bundling your application with C or C++ libraries.
When converting Python applications that interact with other programming languages, such as Java, it is important to include the required dependencies and interfaces. Tools like Jython and JPype can be employed for Java integration, but ensure you properly package these dependencies during the conversion process.
Frequently Asked Questions
How can I create an exe file from a Python script with all dependencies included?
To create an exe file from a Python script with all dependencies included, you can use a tool such as PyInstaller. PyInstaller packages your Python script and its dependencies into a single executable, making it easy to share and run on systems without Python installed. Simply install PyInstaller using pip, and then run the command pyinstaller --onefile your_script.py
.
What is the best method to convert a Python project to a standalone executable in Windows?
The best method to convert a Python project to a standalone executable in Windows is using tools like PyInstaller or cx_Freeze. Both tools are capable of generating standalone executables, and they offer different options and customizations depending on your needs. Make sure to read their respective documentations to choose the one that suits your project best.
How do I use PyInstaller effectively to create an exe from a Python file?
To use PyInstaller effectively, first install it by running pip install pyinstaller
. Then, you can create an exe by running pyinstaller --onefile your_script.py
in the command line. For more advanced usage, like hiding the console window, use options like --noconsole
. You can also create a configuration file for PyInstaller, known as a .spec
file, to apply more customization options like icon files or additional data. Read the PyInstaller documentation for more details about these options.
Is there a way to make a Python file executable and auto-install required packages?
Although making a Python file executable and auto-installing required packages isn’t directly possible, you can use PyInstaller to bundle your script and its dependencies into a single executable. Alternatively, you can use pipenv or conda to create a virtual environment that includes all dependencies, making it easier for others to run your script.
How does one convert a multi-file Python project to a single executable?
Converting a multi-file Python project to a single executable is similar to converting a single script. Tools like PyInstaller and cx_Freeze automatically detect and include imports from other files in your project. Run the command pyinstaller --onefile your_main_script.py
or follow the cx_Freeze documentation to create a standalone executable that includes all files in your project.
Are there alternative tools to PyInstaller for creating standalone Python executables?
Yes, there are alternative tools to PyInstaller for creating standalone Python executables. Some popular alternatives include cx_Freeze, Nuitka, and py2exe. Each tool has its own features, options, and limitations. Consider the specific requirements of your project when choosing the right tool for you.
Recommended: Top 20 Profitable Ways to Make Six Figures Online as a Developer (2023)
The post Python to EXE with All Dependencies appeared first on Be on the Right Side of Change.
September 10, 2023 at 06:20PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
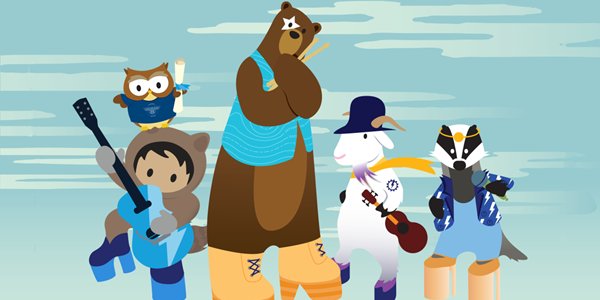
Post a Comment