Python Return Iterator From Function : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
To return an iterator from a Python function, you can use the yield
keyword. Unlike the return
statement, yield
produces a value and suspends the function’s execution. The function can be resumed later on from where it left off, allowing it to produce a series of values over time, instead of computing them all at once and sending them back in a list, for example.
Here’s an example:
def simple_generator(): for i in range(3): yield i # Create an iterator using the generator function my_iterator = simple_generator() # Iterate through the values from the generator function for value in my_iterator: print(value)
In this example, the simple_generator
function is a generator function that yields a series of values (0, 1, 2). When the simple_generator
function is called, it returns an iterator that can be iterated over to retrieve these values one at a time.
Understanding Python Iterators
Python iterators are essential tools for efficiently looping through iterables, such as lists, tuples, and dictionaries. An iterator is an object that implements the iterator protocol, which consists of two methods: __iter__()
and __next__()
.
Iterables are objects that can be looped through to access their elements.
All iterable objects, including lists, tuples, and dictionaries, have an associated iterator that allows traversal through their elements. To create an iterator for a given iterable, the iter()
function is called with the iterable as the argument1.
my_list = [1, 2, 3] my_iterator = iter(my_list)
Iterators provide a clean and efficient way of looping through elements in an iterable using a for
loop. When looping through an iterator with a for
loop, the loop accesses each value in the iterator one after another until all values are exhausted:
for element in my_iterator: print(element)
Python iterator objects are useful when you need to generate a sequence of data that can be processed one at a time to minimize memory usage, or when you want to implement a custom iterator that behaves differently from the built-in ones.
For example, suppose you want to create an iterator that generates a series of numbers following specific rules or algorithms, like the Fibonacci sequence. You can define a custom iterator class that implements the iterator protocol, using __iter__()
and __next__()
methods:
class FibonacciIterator: def __init__(self): self.a, self.b = 0, 1 def __iter__(self): return self def __next__(self): nxt = self.a self.a, self.b = self.b, self.a + self.b return nxt
By understanding Python iterators and leveraging their capabilities, you can write more efficient and flexible code for your data processing tasks.
Iterator Protocol and Special Methods
In Python, the iterator protocol is a set of rules that an object must follow to be considered an iterator. This protocol involves implementing two special methods, __iter__()
and __next__()
. These methods enable efficient, iterative operations on a collection of objects or data structures.
The __iter__()
method is implicitly called at the start of loops or when an object is used as an iterator. It should return the iterator object itself, allowing the iterator to be used in loops and other iterable contexts.
class MyIterator: def __iter__(self): # Initialization code goes here return self
The __next__()
method is called implicitly at each loop increment to retrieve the next element in the iterator sequence. It should return the next value or raise a StopIteration
exception if there are no more items available.
class MyIterator: def __iter__(self): # Initialization code goes here return self def __next__(self): # Code to retrieve the next element goes here if no_more_elements: raise StopIteration return next_element
By implementing these two methods, you adhere to the iterator protocol, and your object can be used with for
loops, comprehensions, and other iterable expressions. It is important to note that the iterator protocol does not require the object to be of a specific type or inherit from a particular class.
Here’s a simple example of an iterator that counts from 0 to a specified limit:
class Counter: def __init__(self, limit): self.limit = limit def __iter__(self): self.value = 0 return self def __next__(self): if self.value >= self.limit: raise StopIteration else: self.value += 1 return self.value - 1 for i in Counter(5): print(i) # Output: 0 1 2 3 4
In this example, we define a Counter
class that implements the iterator protocol using the __iter__()
and __next__()
methods. The iterator starts with a value of 0 and increments it at each step until it reaches the specified limit. The Counter
class can then be used with various Python constructs that expect iterators.
Python Generator Functions
Generator functions are a special type of function in Python that return a lazy iterator. These iterators can be looped over like a list, but unlike lists, they do not store their contents in memory, making them more memory-efficient when working with large datasets.
To create a generator function, you use the yield
keyword instead of return
. Whenever the yield
keyword is encountered in a function, the function’s state is stored, and execution is paused. When the function is called again, execution resumes from the point where it was paused.
Here’s a simple example of a generator function:
def simple_generator(): yield 1 yield 2 yield 3 for num in simple_generator(): print(num)
In this example, the function simple_generator
yields three values: 1, 2, and 3. When the generator function is looped over using a for
loop, these values are printed one at a time.
Another useful feature of generator functions is their ability to receive values while iterating. This can be done using the .send()
method of a generator.
Here’s an example:
def receive_value_generator(): received_value = yield print('Received:', received_value) generator_instance = receive_value_generator() next(generator_instance) # Start the generator generator_instance.send('Hello, world!') # Send a value to the generator
In this example, the generator function receive_value_generator
yields its first value, waiting to receive a value via the send()
method. After starting the generator with the next()
function, a value is sent using the send()
method, and the received value is printed.
Generator Expressions and List Comprehensions
Generator expressions and list comprehensions are commonly used to create and transform iterables such as lists, sets, and dictionaries.
A generator expression (or genexp) is similar to a list comprehension, but it returns an iterator instead of a list. This makes it more memory-efficient for working with large data sets, as it only computes the values as needed. A genexp is created using parentheses instead of square brackets and has a very similar syntax to list comprehension.
For example, the following code creates a generator expression that generates a sequence of squared numbers:
squared_genexp = (x**2 for x in range(10))
You can then use the next()
function or a for
loop to iterate through the generated values. Note that the iterator created by a generator expression can only be iterated once.
List comprehensions provide a concise way to create lists using a single line of code. The syntax is very similar to generator expressions but uses square brackets. List comprehensions are ideal for small to medium-sized data sets, where readability and creation speed are more important than memory efficiency.
Here’s an example of a list comprehension that creates a list of squared numbers:
squared_list = [x**2 for x in range(10)]
Both generator expressions and list comprehensions support filtering through an optional if
statement.
For example, you can create a list of even numbers using the following list comprehension:
even_list = [x for x in range(10) if x % 2 == 0]
Similarly, you can create a generator expression that generates even numbers:
even_genexp = (x for x in range(10) if x % 2 == 0)
Creating Custom Iterator Classes
In Python, iterators are essential components of object-oriented programming, allowing you to create efficient and memory-friendly loops, among other things. In this section, we’ll look at how to create a custom iterator class by implementing the __iter__()
and __next__()
methods in a class.
A custom iterator class allows you to define how your objects will be iterated over. The first method you need to implement is __iter__()
. This method returns the iterator object itself, which can be initialized if necessary.
For instance, consider a custom iterator class that iterates through a given range of numbers:
class RangeIterator: def __init__(self, start, end): self.start = start self.end = end def __iter__(self): return self
The second essential method is __next__()
. This method must return the next item in the sequence. If there are no more items to return, it should raise the StopIteration
exception.
Here’s how you’d implement the __next__()
method within the RangeIterator
class:
class RangeIterator: # ... (previous code) def __next__(self): if self.start >= self.end: raise StopIteration current = self.start self.start += 1 return current
With the custom iterator class complete, you can create an object of this class and use it in a loop:
my_range = RangeIterator(0, 5) for i in my_range: print(i)
This code will output the numbers from 0 to 4, as the custom RangeIterator
class defines how the iteration proceeds through the given range.
Iterating Through Built-In Collections
In Python, there are several built-in collections like lists, tuples, dictionaries, sets, and the collections
module which provide different types of containers. Iterating through these collections can be done using loops and Python’s iterator protocol.
Lists and Tuples
Lists and tuples are ordered collections of elements. You can iterate through them using a for
loop. Here’s an example of iterating through a list and a tuple:
my_list = [1, 2, 3, 4, 5] my_tuple = (6, 7, 8, 9, 10) for item in my_list: print(item) for item in my_tuple: print(item)
Dictionaries
Dictionaries are key-value pairs. When iterating through a dictionary, you can loop through either its keys, values, or both. Here’s an example for each case:
my_dict = {'a': 1, 'b': 2, 'c': 3} # Iterating through keys for key in my_dict: print(key) # Iterating through values for value in my_dict.values(): print(value) # Iterating through key-value pairs for key, value in my_dict.items(): print(key, value)
Sets
Sets are unordered collections of unique elements. Similar to lists and tuples, you can iterate through sets using a for
loop:
my_set = {1, 2, 3, 4, 5} for item in my_set: print(item)
Collections Module
The collections
module provides specialized container data types like Counter
, deque
, defaultdict
, and OrderedDict
, among others. You can iterate through these data structures using a for
loop or other iterator methods.
For example, iterating through a Counter
object:
from collections import Counter count_items = Counter(['a', 'b', 'b', 'c', 'c', 'c']) for item, count in count_items.items(): print(item, count)
Python’s Iterator Functions
In Python, iterators are objects that can be iterated upon. They define a specific protocol consisting of the __iter__()
and __next__()
methods, which allow you to loop through the object and retrieve its elements. The built-in functions iter()
and next()
make the process of implementing and iterating through iterators simple and efficient.
The __iter__()
method should return the iterator object itself, while the __next__()
method should return the next value from the iterator. When there are no more items left, the __next__()
method should raise the StopIteration
exception.
Here’s an example of a simple iterator:
class MyNumbers: def __iter__(self): self.a = 1 return self def __next__(self): x = self.a self.a += 1 return x my_numbers = MyNumbers() iterator = iter(my_numbers) print(next(iterator)) print(next(iterator))
In this example, the MyNumbers
class has implemented the iterator protocol. The iter()
function is used to obtain the iterator object, while the next()
function is used to retrieve the next value in the sequence.
You can also create an iterator in Python using generator functions, which use the yield
keyword instead of explicitly defining the __iter__()
and __next__()
methods. Here’s an example using a generator function:
def my_numbers(): a = 1 while True: yield a a += 1 iterator = iter(my_numbers()) print(next(iterator)) print(next(iterator))
This example demonstrates a similar functionality as the previous example, but with less code. The generator function my_numbers()
is defined with the yield
keyword, and the iterator object is created using the iter()
function. The next()
function once again retrieves the next value in the sequence.
Infinite Iterators and itertools Module
In Python, the itertools
module provides various functions to create and manipulate iterators, including infinite iterators. Infinite iterators can generate an endless sequence of values, which can be helpful for certain applications or algorithms. Among the functions provided by the itertools
module are count()
, which produces an infinite sequence of evenly spaced values.
For example, the count()
function can be used to generate an infinite sequence of numbers, starting at a specified value and incrementing by a step value:
import itertools # Generate an infinite iterator that starts at 5 and increments by 2: numbers = itertools.count(5, 2) # Print the first 10 numbers from the iterator: for _ in range(10): print(next(numbers))
This code would output the sequence 5, 7, 9, 11, 13, 15, 17, 19, 21, 23, demonstrating the ability of count()
to generate an infinite iterator.
There are other infinite iterators in the itertools module, such as cycle()
and repeat()
. However, it is crucial to use such iterators wisely to avoid infinite loops in your code. To control the length of an infinite iterator, you can combine it with other itertools functions, like islice()
, which can take a slice of a given iterator.
Additional Python Modules for Iteration
In this section, we will discuss some additional Python modules that can be helpful for efficiently working with iterators. These include the operator
, itertools
, and functools
modules.
The operator
module provides a set of convenient functions that correspond to built-in Python operators. These functions are particularly useful when working with iterators since they can be passed as arguments to functions such as map()
or reduce()
. For example, using operator.add
with Python’s built-in reduce()
function can efficiently calculate the sum of a sequence:
import operator from functools import reduce numbers = [1, 2, 3, 4, 5] result = reduce(operator.add, numbers) print(result) # Output: 15
The itertools
module is another valuable resource for handling iterators. Among its many functions, a couple of notable ones are accumulate()
and groupby()
. The accumulate()
function generates an iterator that returns accumulated results for each element in an iterable:
import itertools numbers = [1, 2, 3, 4, 5] result = list(itertools.accumulate(numbers)) print(result) # Output: [1, 3, 6, 10, 15]
The groupby()
function creates an iterator that groups consecutive elements in an input iterable based on a key function. This can be particularly helpful when processing sorted data:
import itertools data = [('apple', 3), ('banana', 7), ('apple', 2), ('orange', 4)] sorted_data = sorted(data, key=lambda x: x[0]) for key, group in itertools.groupby(sorted_data, key=lambda x: x[0]): print(key, list(group)) # Output: # apple [('apple', 3), ('apple', 2)] # banana [('banana', 7)] # orange [('orange', 4)]
Finally, the functools
module provides higher-order functions that can help manipulate functions or callable objects. One important function in this module is reduce()
, which we already used in a previous example. Another example of a useful function in functools
is partial()
, which allows you to fix a certain number of arguments for a function and generate a new function:
import functools import operator add_five = functools.partial(operator.add, 5) result = add_five(3) print(result) # Output: 8
As you can see, these additional Python modules for iteration provide efficient and handy tools for working with iterators and iterables. Utilizing these modules can greatly enhance the readability and performance of your Python code.
Practical Examples
In this section, we will explore some practical examples of using Python iterators from functions. We will cover concepts such as yield statement, input values, sequencing, and generator functions while keeping the tone confident, knowledgeable, neutral, and clear.
Example 1: Simple generator function
A generator function is a type of iterator that is defined using a function with the yield
statement. Let’s create a simple generator that yields numbers from 0 to n
.
def simple_generator(n): i = 0 while i <= n: yield i i += 1 for number in simple_generator(5): print(number)
In this example, the yield
statement is responsible for producing a sequence of numbers from 0 to n
. When the generator function is called, it returns an iterator that can be consumed using a for loop or the next()
function.
Example 2: Filtering input values with a generator function
Let’s create a generator function that filters out even numbers from a given list:
def filter_even_numbers(numbers): for number in numbers: if number % 2 != 0: yield number input_values = [1, 2, 3, 4, 5, 6, 7, 8] filtered_values = filter_even_numbers(input_values) for value in filtered_values: print(value)
Here, the generator function filter_even_numbers
filters out even numbers by only yielding odd numbers from the input list. This example demonstrates how we can process input values using a generator function.
Example 3: Generating a Fibonacci sequence with a generator function
A Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones, usually starting with 0 and 1. Let’s implement a generator function to generate the first n
numbers of the Fibonacci sequence:
def fibonacci(n): current, next_value = 0, 1 for _ in range(n): yield current current, next_value = next_value, current + next_value for number in fibonacci(10): print(number)
This generator function uses the yield
statement to produce the Fibonacci sequence up to the n
numbers. Implementing iterators with generator functions provides a concise and memory-efficient way to generate sequences.
Frequently Asked Questions
How do I create a custom iterator in Python?
To create a custom iterator in Python, you need to define a class that implements the __iter__()
and __next__()
methods. Here’s an example:
class Counter: def __init__(self, limit): self.limit = limit self.count = 0 def __iter__(self): return self def __next__(self): if self.count < self.limit: result = self.count self.count += 1 return result else: raise StopIteration counter = Counter(3) for number in counter: print(number)
What is the difference between iterators and generators in Python?
Iterators are objects that implement the iterator protocol with __iter__()
and __next__()
methods, whereas generators are functions that use the yield
keyword to return a sequence of values. Generators are more memory-efficient as they only produce one value at a time upon request.
How can I use the ‘next’ method with Python iterators?
The next()
function is used to retrieve the next item from an iterator. Here’s an example:
iterable = [1, 2, 3] iterator = iter(iterable) print(next(iterator)) # Output: 1 print(next(iterator)) # Output: 2
How to convert a Python list into an iterator?
To convert a Python list to an iterator, use the iter()
function:
my_list = [1, 2, 3] iterator = iter(my_list)
What is the difference between an iterable and an iterator in Python?
An iterable is any object that can be used in a for
loop, such as lists, tuples, and strings. An iterator is an object that iterates over an iterable using the __iter__()
and __next__()
methods. All iterators are iterables, but not all iterables are iterators.
How can I check if a Python iterator has more elements?
You can use next(iterator, default)
to check if an iterator has more elements, without causing a StopIteration
exception. The default
can be any value, which will be returned if the iterator is exhausted:
iterable = [1, 2, 3] iterator = iter(iterable) print(next(iterator, None)) # Output: 1 print(next(iterator, None)) # Output: 2 print(next(iterator, None)) # Output: 3 print(next(iterator, None)) # Output: None
July 29, 2023 at 04:40PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
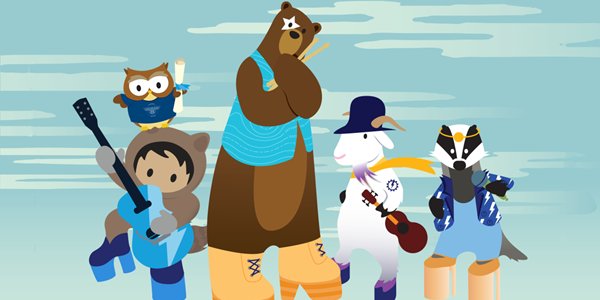
Post a Comment