Python — How Do I Import a Module from the Parent Folder? : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
The sys.path.append("..")
command in Python adds the parent directory (represented by ".."
) to the list of paths that Python checks for modules. After executing this command, you can import any module from the parent directory. The ".."
denotes one directory up from the current location, thus referring to the parent directory.
Here’s a minimal example:
import sys sys.path.append("..") import your_module
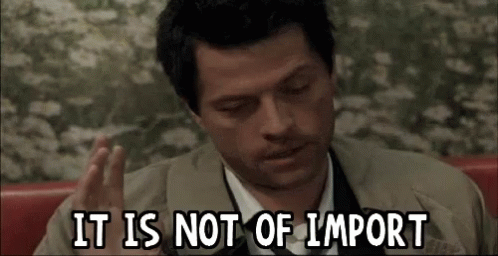
Problem Formulation
As a Python developer, you might encounter a situation where you need to import a module from the parent folder. The common folder structure you may find in a Python project looks like this:
parent_directory/
|--- module_a.py
|--- folder/
|--- module_b.py
Now, let’s assume you’re working on module_b.py
, and you need to import a function or class from module_a.py
. The default import mechanism in Python does not allow direct imports from parent folders, which might result in ModuleNotFoundError
or ImportError
.
Let’s explore a few reliable methods to solve this problem and import modules from parent folders:
Method 1: sys.path.append()
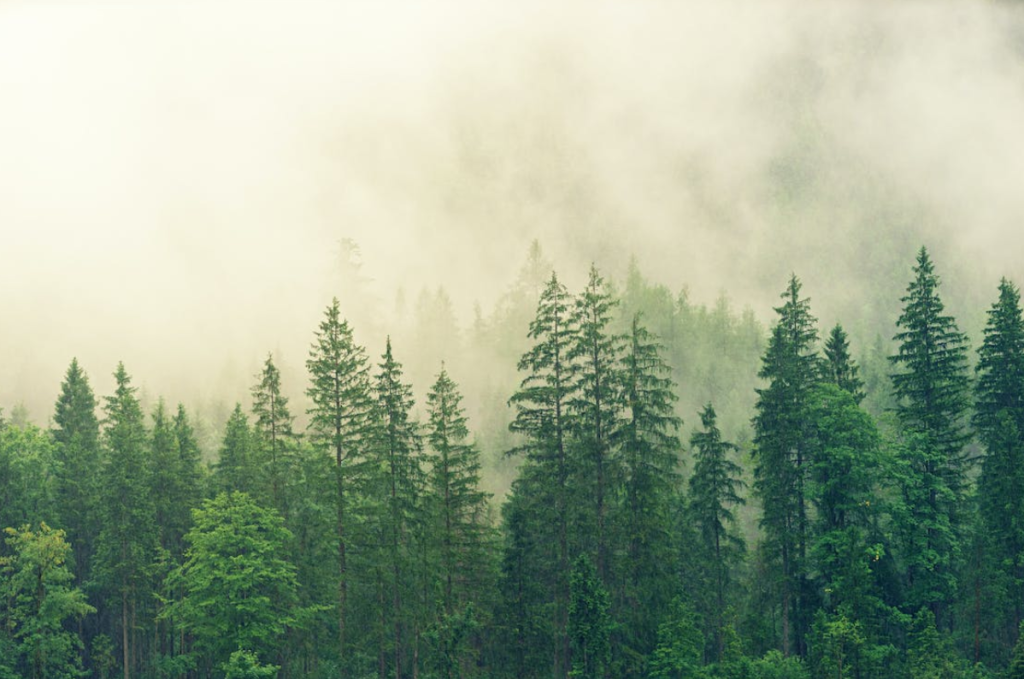
Method 1 involves using the sys.path
method to add the parent directory to the interpreter’s search path. Start by importing the sys
module and then appending the parent directory to sys.path
using the append()
method. You can add a specific path for interpreters to search.
For example, you can use the following code:
import sys sys.path.append("..") import your_module
Method 2: Declare Parent as Package
Method 2 requires declaring the parent directory as a package by adding the __init__.py
file. After declaring the directory as a package, you can use the relative package approach to import the module.
For instance, you might have the following code:
from .. import your_module
Method 3: Operating System Module and Sys Path
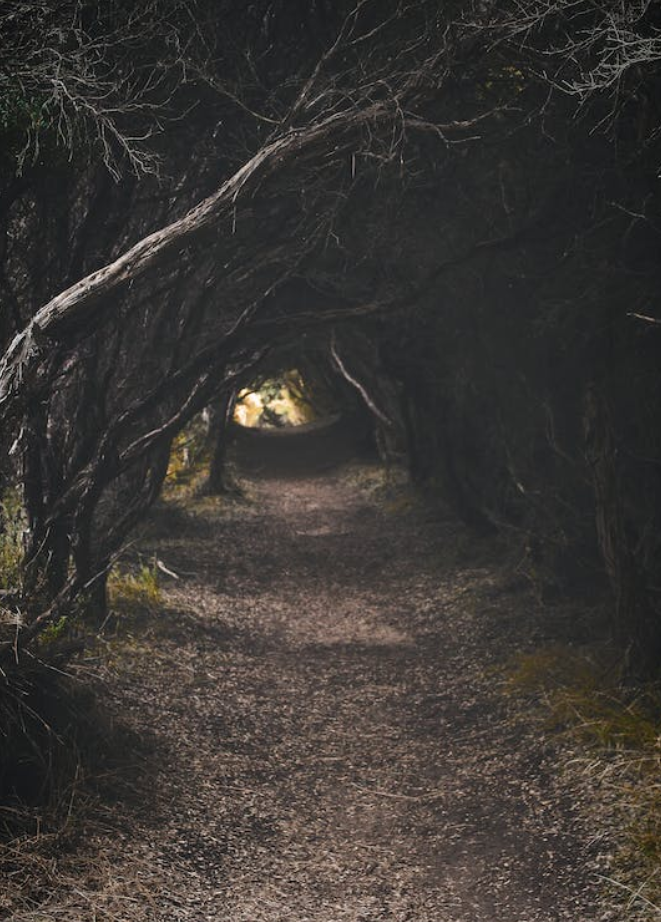
Method 3 uses the os
module in combination with sys.path
. Instead of using ..
to reference the parent directory, this method provides a more explicit approach. First, import both the os
and sys
modules, then use the following code to append the parent directory to the search path:
import os import sys sys.path.append(os.path.dirname(os.path.abspath(__file__))+"/..") import your_module
Method 4: PathLib Module
Method 4 involves the use of the pathlib
module, which is available in Python 3.4 and later versions. Similar to the previous methods, you would need to append the parent directory to the search path, but this time using the pathlib
module.
The code for this method looks like:
from pathlib import Path import sys sys.path.append(str(Path(__file__).resolve().parent.parent)) import your_module
So far, so good. Let’s get a deeper understanding of how all the package, module, and library stuff really works. In other words, let’s dive into Python Dependency Management 101.
Understanding Python Modules and Packages
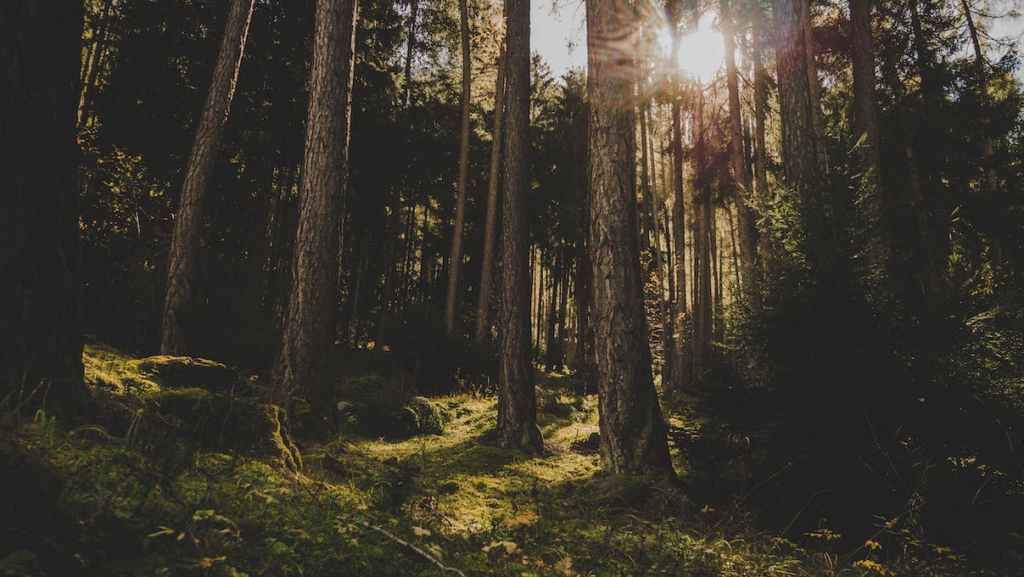
In Python, you’ll often work with both modules and packages. A module is a single file with a .py
extension, while a package is a folder containing one or more module files, typically with an __init__.py
file.
Recommended: Python — How to Import Modules From Another Folder?
When working on a large project, you might need to import a module from the parent folder. Understanding module and package organization will help you do this more effectively.
To import a module located in the parent folder, modify the sys.path
variable. This variable tells Python where to look for modules and packages. Add the parent folder’s path to sys.path
before importing your desired module, as shown below:
import sys sys.path.append("..") import your_module
This code snippet adds the parent folder to the sys.path
, allowing you to import the desired module. Remember to replace your_module
with the actual name of the module you want to import.
Another useful import method is from ... import ...
. For example, if you want to import only a specific function or class from a module, you can use this syntax:
from your_module import your_function_or_class
Keep in mind that using relative imports like from .parent_folder import your_module
is typically recommended only within packages, as it can lead to confusion when used in standalone scripts.
Python Directory Structure
When working with Python, understanding the directory structure is essential to managing and importing modules effectively. The directory structure typically involves a series of folders, including the
- parent directory,
- current directory, and
- subdirectories.
The parent directory is the folder containing your current working folder or file. A subdirectory is a folder within the current directory.
To successfully import a module from the parent folder, you need to modify the Python import path. The standard approach to achieve this is by using the sys.path
method. Start by importing the sys
module, then append the parent directory to sys.path
.
For example:
import sys sys.path.append("..")
In the above code, ".."
represents the parent directory path. After appending the parent directory to sys.path
, you can now import modules from the parent folder as usual:
import module_name
Ensure to choose meaningful identifiers for your directories and modules, and keep the directory path clear and concise. This ensures better organization and readability for yourself and other developers working on the project.
Using sys.path

One efficient method to import a module from a parent folder is by using the sys.path
feature. In this brief section, you will learn how to utilize this approach using sys.path to import modules from a parent directory.
To begin, you should first understand what sys.path
is.
It’s a Python list that consists of the directories the Python interpreter will search through when attempting to import a module. By manipulating this list, you can easily direct the interpreter to the desired location.
To use sys.path
for importing a module from a parent folder, you’ll need to do the following:
- Start by importing the
sys
library, which grants access to the system-specific parameters and functions:import sys
- Next, append the parent directory to the
sys.path
list. This allows the Python interpreter to locate the necessary module. Here’s an example:sys.path.append('/path/to/parent/folder')
. - Replace
/path/to/parent/folder
with the actual path to the parent directory containing the module you want to import. - Now, with the parent folder added to your system path, you can proceed to import the desired module. For instance:
from some_module import desired_class_or_function
Remember to use sys.path
to add the appropriate paths to your interpreter’s search scope, allowing for seamless module importation.
Manipulating PYTHONPATH Environment Variable
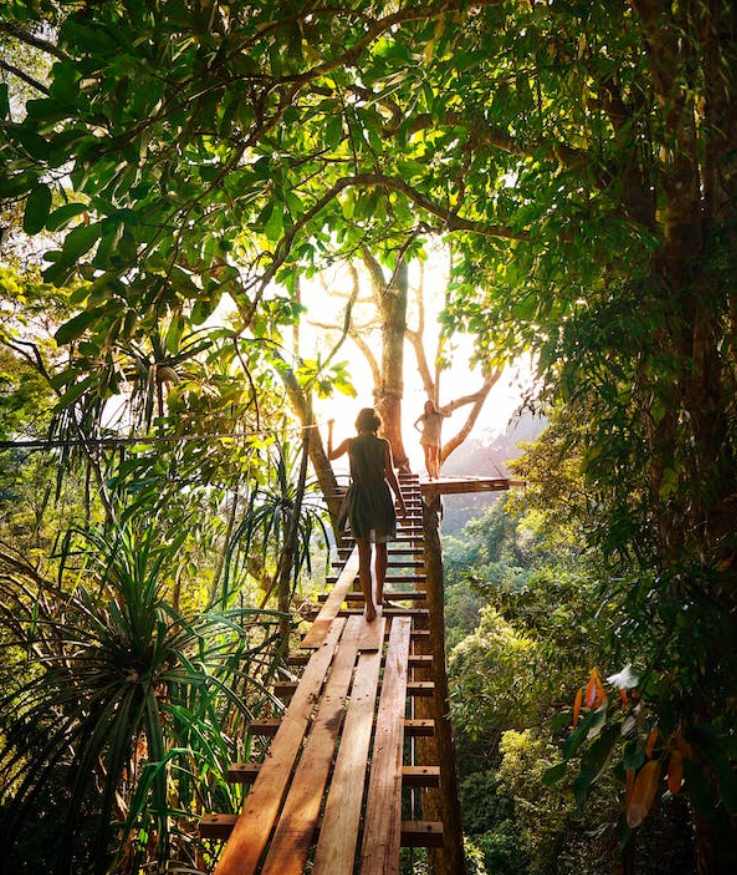
Adjusting the PYTHONPATH
environment variable is one way to import a module from the parent folder in Python. This variable tells Python interpreter where to look for modules when importing them. By specifying the parent folder in the PYTHONPATH
, you make it easier to import modules from it.
On Windows operating systems, you can set the PYTHONPATH
environment variable using the following steps:
- Open the Start menu and search for “Environment Variables.”
- Select “Edit the system environment variables” from the search results.
- In the “System Properties” window, click on the “Environment Variables” button.
- Under “System variables,” click on “New” if there is no
PYTHONPATH
variable present, or find the existingPYTHONPATH
variable and click “Edit.” - In the “Variable name” field, type
PYTHONPATH
(if it’s not already there), and in the “Variable value” field, enter the path to the parent folder where your desired module is located. Separate multiple paths with a semicolon (;
).
For example, if your module is located in C:\Projects\MyProject\
, the “Variable value” should be C:\Projects\MyProject\
.
Once you’ve set your PYTHONPATH
, you’ll need to restart any active Python processes for the changes to take effect. After that, you should be able to import the desired module from the parent folder using the standard Python import statement.
Keep in mind that manipulating the PYTHONPATH
can sometimes lead to inconsistencies or conflicts when working with different projects.
So I’d advise you not to mingle with it.
Relative Imports and init.py
In Python, relative imports are commonly used to access modules and functions within a package. They provide a simple and organized way to manage your code, especially when working with complex package structures. To fully understand relative imports, it’s essential to be familiar with the concept of __init__.py
files.
The __init__.py
file is a crucial component of a Python package. It serves as an initialization script that is executed whenever the package is imported. The presence of __init__.py
in a directory defines it as a Python package, allowing you to perform relative imports.
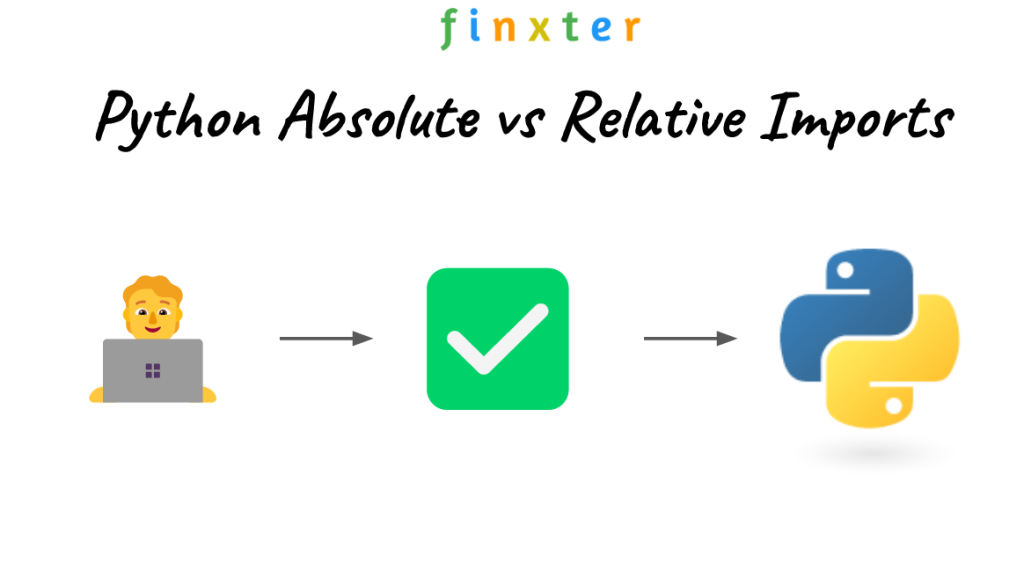
Recommended: Python Absolute vs Relative Imports
To import a module from the parent folder using relative imports, you need to ensure that your package hierarchy meets the following criteria:
- Your top-level package is located within the Python path.
- Each folder within the package contains a valid
__init__.py
file.
With these requirements in place, you can perform a relative import using a series of dots (.
). Each dot represents a level in your package hierarchy, starting from the current folder.
For example, to import a module from the parent folder, prepend ..
to the module name as follows:
from ..module_name import function_name_or_class_name
Importing with Importlib
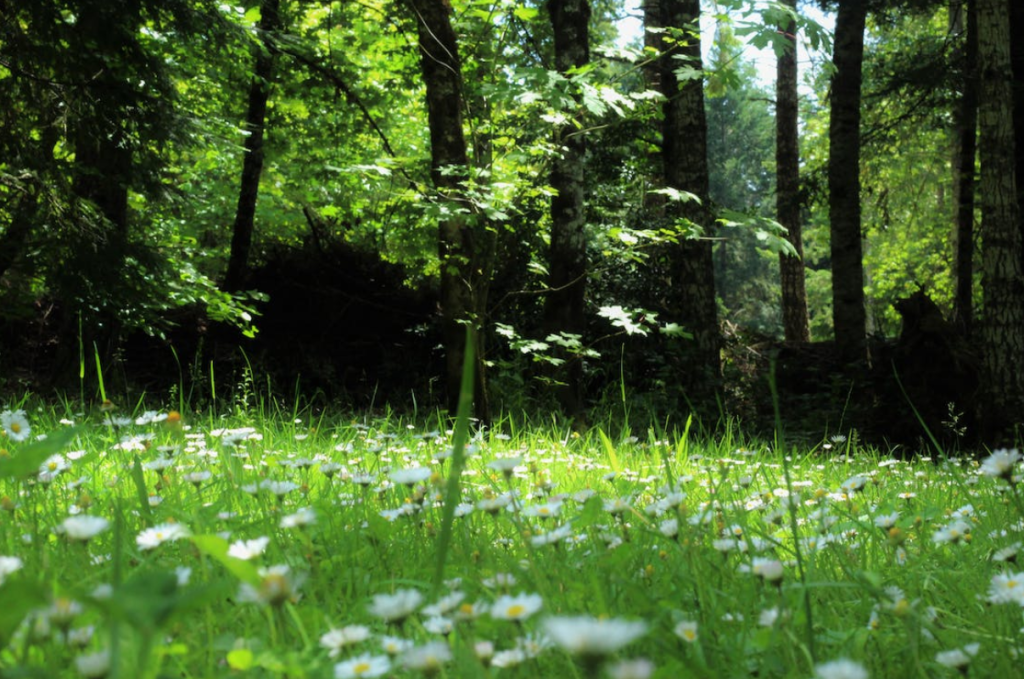
Importing a module from a parent folder in Python can be achieved using the importlib
library. This library is specifically designed to handle the dynamic loading of modules, making the process easier and more efficient.
First, you need to ensure that the importlib
library is installed in your Python environment. It is a built-in library since Python 3.1, so installing it separately should be unnecessary. You can use the function to import a module from the parent folder. This function requires the name of the module you want to import and the package’s location.
Assuming you have a directory structure like this:
parent_directory/
main.py
sub_directory/
module_to_import.py
Here is an example of how to import module_to_import.py
in main.py
using importlib
:
import sys import importlib.util sys.path.append("../") spec = importlib.util.find_spec("sub_directory.module_to_import") module = importlib.util.module_from_spec(spec) spec.loader.exec_module(module)
Keep in mind that the sys.path.append("../")
line is essential for adding the parent directory to the system’s path so that Python can locate the module you want to import.
If the module is found, the find_spec()
function will return a specification that describes how to load it.
The module_from_spec()
function will then create a new module object based on this information, and finally, the exec_module()
function will execute the module.
Attention: When using
importlib
, it is important to handle exceptions properly, such as ValueError
and ModuleNotFoundError
. These exceptions may occur when the specified module could not be found, or another issue occurred during the import, such as an incorrect filename
. Proper exception handling will allow you to provide useful feedback to the user or log the error for debugging purposes.
Using Setup.py and Editable Install
To import a module from a parent folder in Python, one method is to create a setup.py
script and use the editable install feature provided by pip
. This approach allows you to make changes to your code without needing to reinstall the package every time.
As a German dev, I love efficiency!
First, create a setup.py
file in the root of your project folder. In this file, you will need to use setuptools.setup()
to provide the necessary information for installing your package.
An example setup.py
file may look like this:
from setuptools import setup, find_packages setup( name='your-package-name', version='0.1', packages=find_packages(), )
Once you have created the setup.py
file, you can install your package in an editable state using the pip install
command.
Just navigate to your project folder in the terminal and run:
pip install -e .
The -e
flag tells pip
to install the package in editable mode, linking it directly to your project folder. This allows you to make changes to your code and have them reflected immediately in your package without reinstalling.
Now that your package is installed, you can import modules from the parent folder using the standard import syntax:
from your_package_name.parent_module_name import your_function_or_class
By following this approach, you can confidently import modules from parent folders and make your Python development process more efficient and streamlined. Remember to use the setup.py
file, the pip install -e
command, and be aware of the editable mode features to optimize your experience.
Handling Import Errors

You might encounter an ImportError if you’re trying to import a module from a parent folder.
Firstly, it’s essential to add the parent folder to the Python system path. This can be done using the os
and sys
modules. You will need to import these modules in your script.
Here’s a code snippet demonstrating how to achieve this:
import os import sys current_dir = os.path.dirname(os.path.abspath(__file__)) parent_dir = os.path.dirname(current_dir) sys.path.append(parent_dir)
In this example, the os.path
module helps in finding the path of the current and parent folders. Then, the parent folder is added to the system path using sys.path.append(parent_dir)
. This allows you to import a module from the parent folder without worrying about import errors related to the file location.
Now that you have added the parent folder to the system path, you can easily import a module from the parent folder using the following syntax:
from parent_module import your_desired_function
Keep in mind that if you have an __init__.py
file in your parent folder and subfolders, it will signal to Python that they are packages, making it much easier to import modules across directories.
Frequently Asked Questions
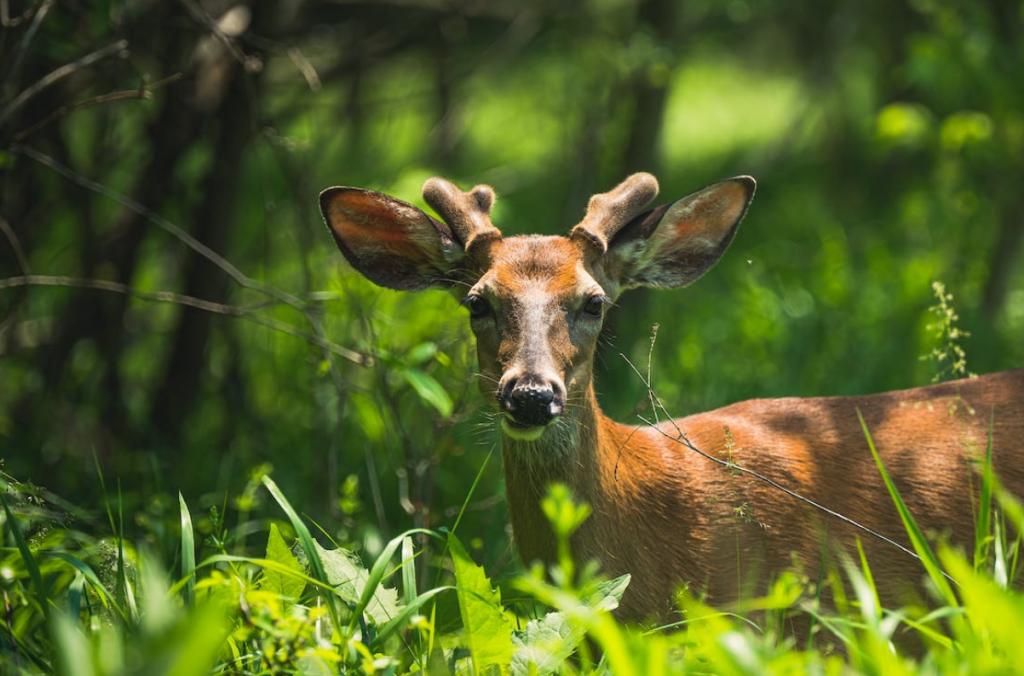
How to access a module in the parent directory in Python?
To access a module in the parent directory in Python, you can use the sys.path
method. First, import the sys
module and append the parent directory to sys.path
. Afterward, you can import the module as you normally would. Here’s a code example:
import sys sys.path.append("..") import module_from_parent_directory
What is the method to import a module from the parent folder in Python 3?
In Python 3, the recommended method for importing a module from the parent folder is to use explicit relative imports with the from ... import
statement. For example, if your module is named my_module
in the parent directory:
from .. import my_module
How do I handle ImportError: attempted relative import with no known parent package?
To resolve the “attempted relative import with no known parent package” error in Python, make sure your script is part of a package by including an __init__.py
file in each directory within the package’s hierarchy. After ensuring your package is correctly structured, you can use explicit relative imports as mentioned earlier.
What is the proper way to import a module from a different directory in Python?
When importing a module from a different directory in Python, use either explicit relative imports or manipulate sys.path
to include the directory you want to import from. For example, to add the directory /path/to/module
and import module_from_different_directory
from there:
import sys sys.path.append("/path/to/module") import module_from_different_directory
How can I import a module from the parent folder in a Jupyter Notebook?
In Jupyter Notebook, to import a module from the parent folder, first, you need to modify the sys.path
to include the parent directory. Here’s an example of how to import a module named my_module
from the parent folder:
import sys sys.path.insert(1, "..") import my_module
How do I fix ‘No module named’ error when importing from parent directory in Python?
To fix the ‘No module named’ error when importing from the parent directory in Python, ensure that you have correctly added the parent directory to sys.path
. If that’s the case but the error still persists, verify the module’s name and ensure you have the right module in the parent directory. Also, check if your package structure has an __init__.py
file in each relevant directory.
Related Articles:
- [Collection] 11 Python Cheat Sheets Every Python Coder Must Own
- [Python OOP Cheat Sheet] A Simple Overview of Object-Oriented Programming
- [Collection] 15 Mind-Blowing Machine Learning Cheat Sheets to Pin to Your Toilet Wall
- Your 8+ Free Python Cheat Sheet [Course]
- Python Beginner Cheat Sheet: 19 Keywords Every Coder Must Know
- Python Functions and Tricks Cheat Sheet
- Python Cheat Sheet: 14 Interview Questions
- Beautiful Pandas Cheat Sheets
- 10 Best NumPy Cheat Sheets
- Python List Methods Cheat Sheet [Instant PDF Download]
- [Cheat Sheet] 6 Pillar Machine Learning Algorithms
July 18, 2023 at 06:58PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
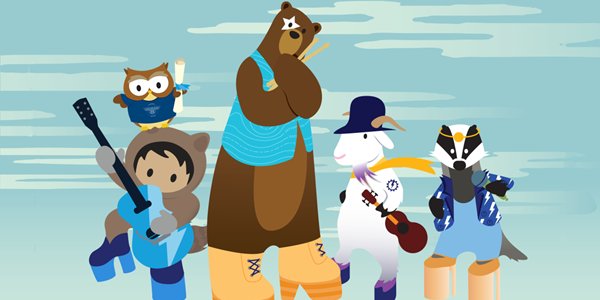
Post a Comment