How to Remove the Percentage % Sign and Convert to Number in Python : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation
Picture this: you’re extracting data from an XML file with a series of percentages. But your percentages look something like this:
8%
-10%
20%
15%
You’ve successfully extracted the data, but these percentages are tagged with the “%” symbol, making them strings rather than numbers.
Now, let’s say you want to do something like:
percentage = "12%" if percentage < 15.0: print('Yay!')
But with that “%” symbol in the way, your Python code raises the TypeError: '<' not supported between instances of 'str' and 'float'
.

So, how can you transform these string percentages into numerical data and make your code run smoothly?
Overview

- String Replacement and Conversion to Float: Removes
'%'
and converts the resulting string to float. - Using String Slicing and Float Conversion: Slices off
'%'
and converts the remaining string to float. - Regular Expression-based Conversion: Uses a regular expression to remove
'%'
and converts the result to float. - Using List Comprehension for Bulk Conversion: A bulk conversion method that utilizes list comprehension to convert all percentages in a list to float.
Method 1: String Replacement and Conversion to Float
This method involves two steps: Firstly, you’ll use Python’s replace()
function to remove the “%” symbol from our string. Then, convert the result to a float using float()
.
Code Example:
percentage = "-38%" percentage_num = float(percentage.replace('%', '')) print(percentage_num)
Output:
-38.0
In this code, replace('%', '')
removes the ‘%’ symbol from the string, leaving just the numeric part. Then, float()
converts this string to a float number, which can be used for numerical comparisons.
If you want to convert to an integer, use the built-in int()
function instead.
Method 2: Using String Slicing and Float Conversion
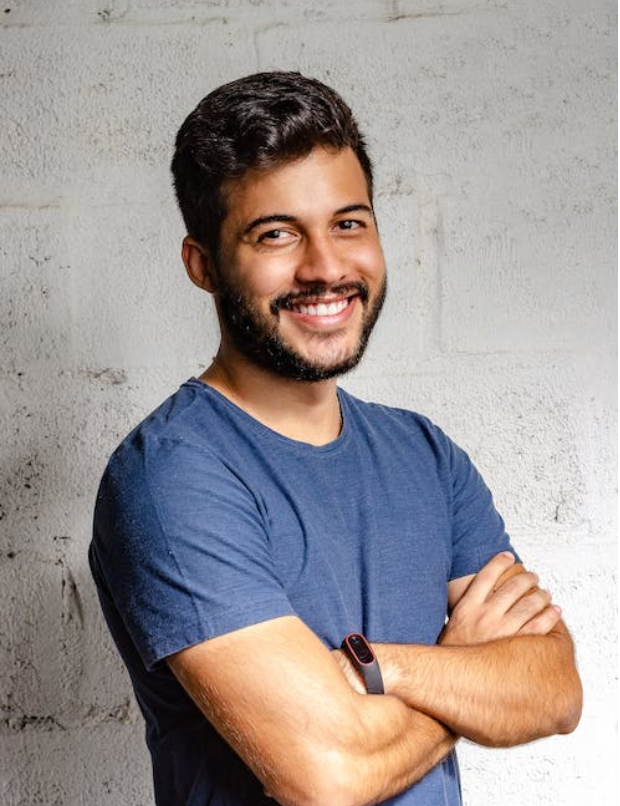
You can utilize Python’s string slicing feature to omit the last character (the “%”) and then convert the remaining part to float.
Code Example:
percentage = "25%" percentage_num = float(percentage[:-1]) print(percentage_num)
Output:
25.0
Here, percentage[:-1]
is used to slice the string, excluding the last character (“%”). The result is then converted to a float using float()
.
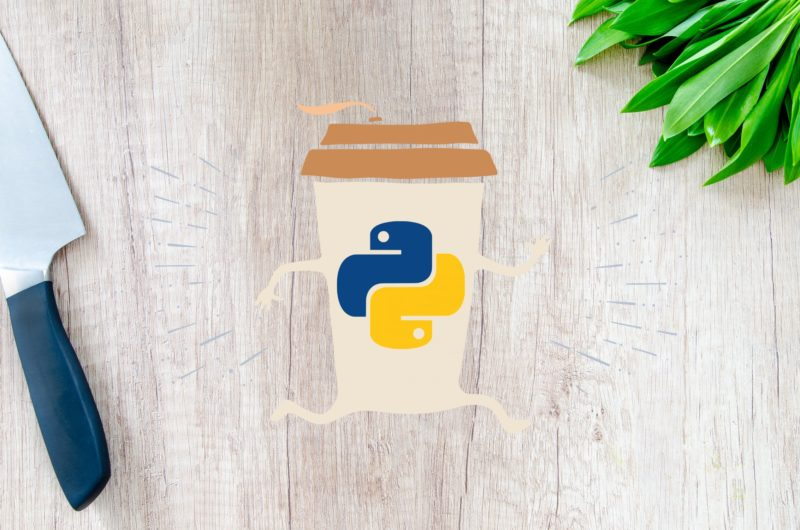
Recommended: Introduction to Slicing in Python
Method 3: Regular Expression-based Conversion
This method uses the re
module, a powerful tool for handling strings. We’ll use a regular expression to remove non-numeric characters and then convert the result to float.
Code Example:
import re percentage = "4%" percentage_num = float(re.sub(r'[^0-9.-]', '', percentage)) print(percentage_num)
Output:
4.0
In this code, re.sub(r'[^0-9.-]', '', percentage)
replaces non-numeric characters with nothing, effectively removing them. We then convert the remaining string to a float.
Method 4: Using List Comprehension for Bulk Conversion
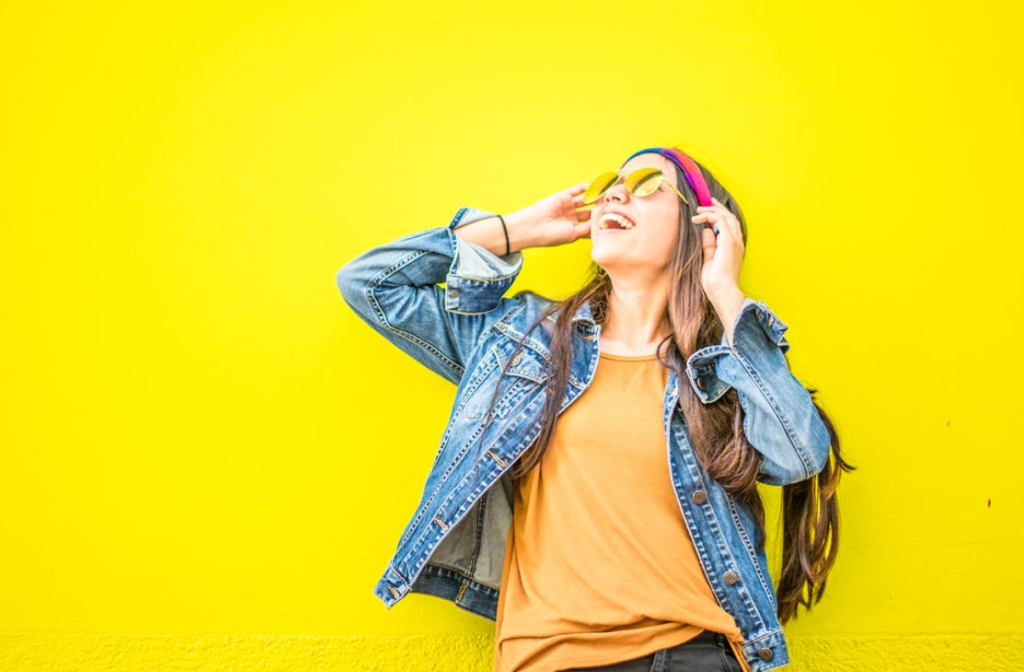
If we have a list of percentages, we can use list comprehension (combined with any of the above methods) to convert all percentages in one go.
Code Example:
percentages = ["-2%", "4%", "25%"] percentages_num = [float(p.replace('%', '')) for p in percentages] print(percentages_num)
Output:
[-2.0, 4.0, 25.0]
Here, we use list comprehension ([float(p.replace('%', '')) for p in percentages]
) to iterate over all percentages, remove the ‘%’ symbol, and convert the result to float.
Recommended: List Comprehension in Python — A Helpful Illustrated Guide
If you want to keep learning Python and tech, check out our free email academy by downloading any of our free Python cheat sheets:
July 18, 2023 at 05:14PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
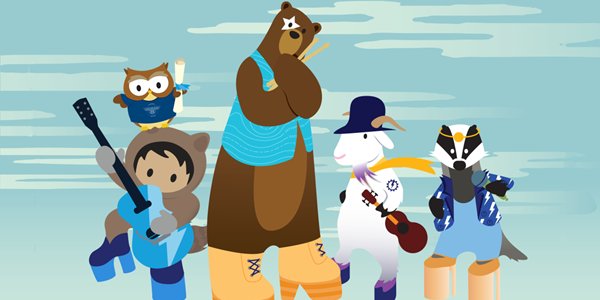
Post a Comment