How I Get YouTube Thumbnails Without API Keys or Libraries (e.g. Python) : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
You can get the YouTube thumbnail if you don’t want to create and use an API key from Google with this simple trick:
YouTube uses a consistent URL pattern for their video thumbnails, which is:
https://img.youtube.com/vi/<video_id>/maxresdefault.jpg
The <video_id>
is the part after "watch?v="
in the YouTube video link.
For example, consider the video at https://www.youtube.com/watch?v=A5I55aOgX2o with the video identifier A5I55aOgX2o.
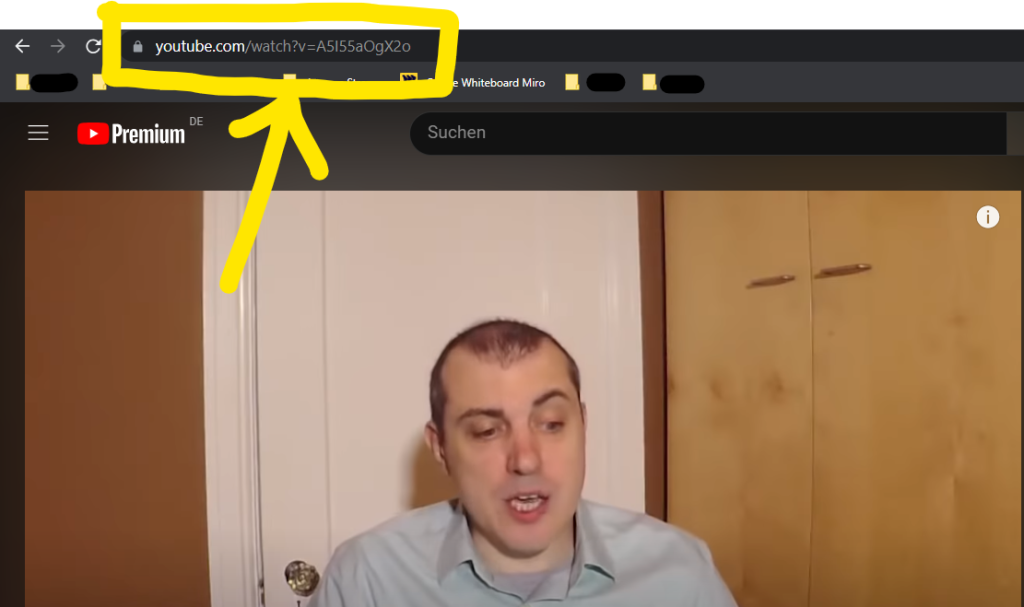
Here’s a simple Python function that will build this URL for you:
def get_thumbnail_url(video_id): return f"https://img.youtube.com/vi/{video_id}/maxresdefault.jpg" video_id = "VIDEO_ID" # Replace with your YouTube video ID thumbnail_url = get_thumbnail_url(video_id) print(thumbnail_url)
This will print out the URL of the thumbnail image. However, please note that this method might not always provide the highest-resolution thumbnail, primarily if the uploaded video doesn’t support high resolution.
Here’s the thumbnail in the above video example: https://img.youtube.com/vi/A5I55aOgX2o/maxresdefault.jpg with the video identifier A5I55aOgX2o.

If you want to download the image, you can use the urllib
and shutil
libraries:
import shutil import urllib.request def download_thumbnail(video_id): thumbnail_url = get_thumbnail_url(video_id) with urllib.request.urlopen(thumbnail_url) as response, open('thumbnail.jpg', 'wb') as out_file: shutil.copyfileobj(response, out_file) download_thumbnail(video_id)
This will save the thumbnail image as 'thumbnail.jpg'
in the current directory.
July 08, 2023 at 11:24PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
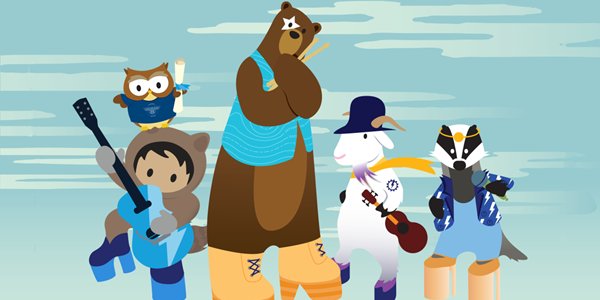
Post a Comment