How to Flatten a List of Lists in Python
by:
blow post content copied from Real Python
click here to view original post
Sometimes, when you’re working with data, you may have the data as a list of nested lists. A common operation is to flatten this data into a one-dimensional list in Python. Flattening a list involves converting a multidimensional list, such as a matrix, into a one-dimensional list.
To better illustrate what it means to flatten a list, say that you have the following matrix of numeric values:
>>> matrix = [
... [9, 3, 8, 3],
... [4, 5, 2, 8],
... [6, 4, 3, 1],
... [1, 0, 4, 5],
... ]
The matrix
variable holds a Python list that contains four nested lists. Each nested list represents a row in the matrix. The rows store four items or numbers each. Now say that you want to turn this matrix into the following list:
[9, 3, 8, 3, 4, 5, 2, 8, 6, 4, 3, 1, 1, 0, 4, 5]
How do you manage to flatten your matrix and get a one-dimensional list like the one above? In this tutorial, you’ll learn how to do that in Python.
Free Bonus: Click here to download the free sample code that showcases and compares several ways to flatten a list of lists in Python.
How to Flatten a List of Lists With a for
Loop
How can you flatten a list of lists in Python? In general, to flatten a list of lists, you can run the following steps either explicitly or implicitly:
- Create a new empty list to store the flattened data.
- Iterate over each nested list or sublist in the original list.
- Add every item from the current sublist to the list of flattened data.
- Return the resulting list with the flattened data.
You can follow several paths and use multiple tools to run these steps in Python. Arguably, the most natural and readable way to do this is to use a for
loop, which allows you to explicitly iterate over the sublists.
Then you need a way to add items to the new flattened list. For that, you have a couple of valid options. First, you’ll turn to the .extend()
method from the list
class itself, and then you’ll give the augmented concatenation operator (+=
) a go.
To continue with the matrix
example, here’s how you would translate these steps into Python code using a for
loop and the .extend()
method:
>>> def flatten_extend(matrix):
... flat_list = []
... for row in matrix:
... flat_list.extend(row)
... return flat_list
...
Inside flatten_extend()
, you first create a new empty list called flat_list
. You’ll use this list to store the flattened data when you extract it from matrix
. Then you start a loop to iterate over the inner, or nested, lists from matrix
. In this example, you use the name row
to represent the current nested list.
In every iteration, you use .extend()
to add the content of the current sublist to flat_list
. This method takes an iterable as an argument and appends its items to the end of the target list.
Now go ahead and run the following code to check that your function does the job:
>>> flatten_extend(matrix)
[9, 3, 8, 3, 4, 5, 2, 8, 6, 4, 3, 1, 1, 0, 4, 5]
That’s neat! You’ve flattened your first list of lists. As a result, you have a one-dimensional list containing all the numeric values from matrix
.
With .extend()
, you’ve come up with a Pythonic and readable way to flatten your lists. You can get the same result using the augmented concatenation operator (+=
) on your flat_list
object. However, this alternative approach may not be as readable:
>>> def flatten_concatenation(matrix):
... flat_list = []
... for row in matrix:
... flat_list += row
... return flat_list
...
This function is similar to flatten_extend()
. The only difference is that you’ve replaced the call to .extend()
with an augmented concatenation. Concatenations like this allow you to append a list of items to the end of an existing list.
Go ahead and call this function with matrix
as an argument:
>>> flatten_concatenation(matrix)
[9, 3, 8, 3, 4, 5, 2, 8, 6, 4, 3, 1, 1, 0, 4, 5]
This call to flatten_concatenation()
returns the same result as your previous flatten_extend()
. Both functions are equivalent and interchangeable. Their goal is to flatten a list of lists. So, it’s up to you to decide which one to use in your code. However, readability-wise, flatten_extend()
seems to be a better solution.
Read the full article at https://realpython.com/python-flatten-list/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
June 26, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
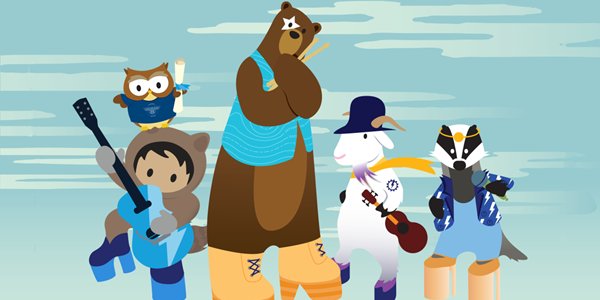
Post a Comment