Python Async Function : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
As a Python developer, you might have encountered the terms async
and await
, wondering what they are and how to use them in your projects.
Async functions enable you to write concurrent code using the async
/await
syntax. This powerful duo allows you to perform multiple tasks simultaneously without blocking the execution of your code. Think of it as having multiple browser tabs open; while one page loads, you can continue browsing other tabs. This capability means your Python applications can become faster, more efficient, and capable of handling many I/O operations.
To get started with async
functions, you’ll need to get acquainted with Python’s asyncio
library, which serves as a foundation for numerous asynchronous frameworks such as high-performance network and web servers, database connection libraries, and distributed task queues.
Mastering async functions can truly elevate your Python programming skills and help you build powerful and responsive applications.
Python Async Function Basics

First, you’ll learn the basics of Python async
functions, which can help improve the performance of your asynchronous programming.
We’ll cover
- async functions without
await
, - an async function example, async function return, and
- async function call.
Async Function Without Await
You might wonder if it’s possible to create an async function without using the await
keyword. Well, it is!
However, without await
, the async function becomes somewhat less useful, since you won’t be able to pause its execution and yield control back to the event loop.
This means your async code will not be able to achieve cooperative concurrency, and other coroutines might be stuck waiting for their turn to execute. It’s generally a good idea to use await
when working with async functions for more efficient asynchronous programming.
Async Function Example
Let’s dive into a simple example of using an async function in Python with asyncio
:
import asyncio async def greet(name: str): print(f"Hello, {name}!") await asyncio.sleep(1) print(f"Nice to meet you, {name}!") async def main(): task1 = asyncio.create_task(greet("Alice")) task2 = asyncio.create_task(greet("Bob")) await task1 await task2 asyncio.run(main())

In this example, an async function greet
is declared, which prints a greeting message, waits for 1 second using asyncio.sleep
, and then prints another message.
The main
asynchronous function creates two tasks to call greet
with different names, running them concurrently.
Async Function Return
When you want to return a value from an async function, just use the return
statement as you would in regular functions. However, keep in mind that the returned value will be wrapped in an asyncio.Future
object, not the actual value.
You’ll need to use await
to get the value when calling this async function.
For example:
async def calculate_result(): await asyncio.sleep(1) return "Result!" async def main(): result = await calculate_result() print(result) asyncio.run(main())
Here, calculate_result
is an async function that returns a value after asynchronously waiting for 1 second. In the main()
function, you can use await
to get the actual value and print it.
Async Function Call
To call an async function, you can’t simply use the normal function call syntax, because doing so would just return a coroutine object, not the actual result of the function. Instead, you have to use the await
keyword to call the async function, or use asyncio.create_task
or similar functions to run it concurrently:
# Using `await` to call async function result = await async_function() # Using `asyncio.create_task` to run concurrently task = asyncio.create_task(async_function())
Remember to always use the appropriate method to call your async functions in order to achieve efficient asynchronous programming with Python’s powerful async/await
syntax.
Advanced Async Function Concepts

Next, you’ll explore advanced async function concepts to give you a better understanding of how they work in Python. Ready? Let’s dive in!
Async Function Decorator
To create an async function, you’ll use the async def
syntax. This means you don’t have to use a decorator, but you can still decorate asynchronous functions with the @some_decorator
syntax for better modularity in your programs.
For instance, consider using @asyncio.coroutine
with a yield from
syntax if you’re working with Python 3.4 or earlier. Or simply upgrade to newer versions!
Async Function Type Hint
Type hints help improve the readability of your async code. Specify the input and output types of your async function using the typing
module’s Coroutine and asyncio
‘s Future objects.
Here’s an example:
from typing import Coroutine import asyncio async def some_async_function() -> Coroutine[str]: await asyncio.sleep(1) return "done"
Async Function Returns Coroutine
An async function, also known as a coroutine, returns a coroutine object when called. You can use it as a direct call or pass it to an event loop to run the async function using asyncio.run()
or loop.run_until_complete()
.
Keep in mind coroutine objects aren’t executed until you explicitly use an event loop or an await
expression.
Async Function Await
When writing async functions, the await
keyword is crucial. It allows you to pause the execution of a coroutine and wait for a result without blocking other coroutines.
You’ll often use await
with I/O-bound operations, like reading from files, interacting with network services, or retrieving resources, which can take a significant amount of time.
Recommended: Python
__await()__
Magic Method
Async Function Type
An async function’s type is coroutine
. So when defining your async function, you’re essentially creating a non-blocking function that allows other functions to run while it waits for results.
To check if an object in Python is a coroutine, you can use inspect.iscoroutine(obj)
or inspect.iscoroutinefunction(obj)
.
Async Function Return Type
Async functions return a coroutine
object, but you can also specify the type of the eventual returned value. For instance, if your async function performs some networking tasks and returns JSON data, you can specify the return type as Dict[str, Any]
.
Here’s how you do that:
from typing import Dict, Any, Coroutine import asyncio async def fetch_json_data() -> Coroutine[Dict[str, Any]]: # some networking tasks here await asyncio.sleep(2) return {"key": "value"}
Async Function in Different Contexts

In this section, we will explore using async functions in different circumstances, such as within classes, threads, and converting async functions to sync. We will also discuss the concepts of async function sleep and handling functions that were never awaited.
Async Function in Class
When working with classes in Python, you might want to include asynchronous methods. To achieve this, just define your class method with async def
. Remember to await
your async methods when calling them to ensure proper execution.
Here’s an example:
class MyClass: async def my_async_method(self): await asyncio.sleep(1) async def main(): my_obj = MyClass() await my_obj.my_async_method()
Async Function in Thread
Running async functions in a thread can be tricky due to event loop requirements. Use asyncio.to_thread()
for running async functions in threads. This will ensure your async function is executed within the correct thread’s event loop.
For example:
async def my_async_function(): await asyncio.sleep(1) print("Hello from async function!") async def main(): result = await asyncio.to_thread(my_async_function)
Async Function to Sync
If you need to call an async function from synchronous code, you can use asyncio.run()
or create an event loop that runs a given coroutine.
Here’s an example of how to run an async function from sync code:
def sync_function(): asyncio.run(my_async_function())
Async Function Sleep
Sometimes, you might want to introduce a delay in your coroutine using asyncio.sleep
. This allows other coroutines to run while waiting for IO operations or other events.
Example:
async def delayed_hello(): await asyncio.sleep(1) print("Hello after 1 second!")
Recommended: Time Delay in Python
Async Function Was Never Awaited
In some cases, you may forget to await
an async function, which leads to warnings such as "coroutine 'my_async_function' was never awaited."
To prevent these issues, always ensure you’re using await
when calling async functions:
async def my_async_function(): await asyncio.sleep(1) async def main(): # Missing 'await' would lead to a warning await my_async_function()
If you got something out of this article, I’m sure you’ll learn something of this one:
Recommended: Python Async With Statement — Simplifying Asynchronous Code
I promise it has more beautiful pics.
May 07, 2023 at 05:09PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
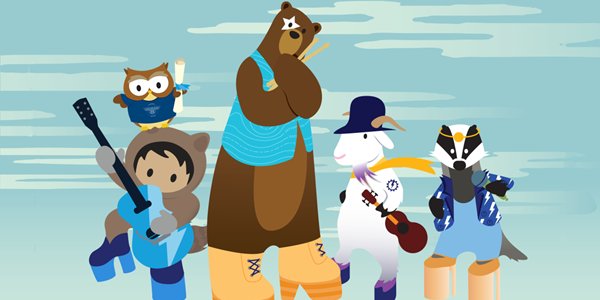
Post a Comment