Pandas Series Object – A Helpful Guide with Examples : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
If you’re working with data in Python, you might have come across the pandas library.
One of the key components of pandas is the Series object, which is a one-dimensional, labeled array capable of holding data of any type, such as integers, strings, floats, and even Python objects .
The Series object serves as a foundation for organizing and manipulating data within the pandas library.
This article will teach you more about this crucial data structure and how it can benefit your data analysis workflows. Let’s get started!
Creating a Pandas Series
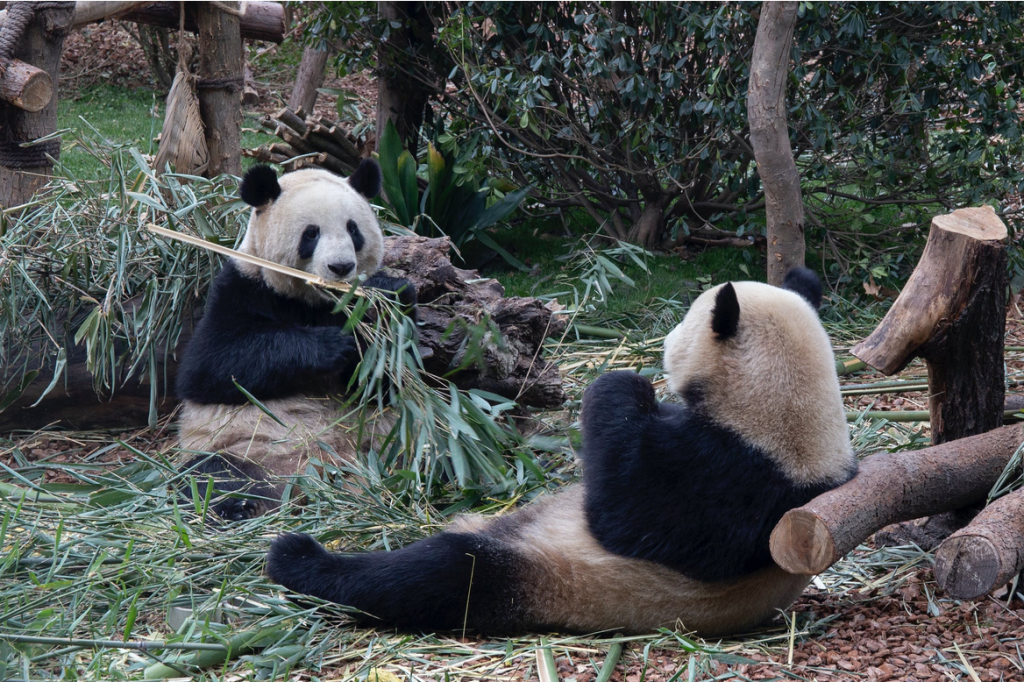
In this section, you’ll learn how to create a Pandas Series, a powerful one-dimensional labeled array capable of holding any data type.
To create a Series, you can use the Series()
constructor from the Pandas library.
Make sure you have Pandas installed and imported:
import pandas as pd
Now, you can create a Series using the pd.Series()
function, and pass in various data structures like lists, dictionaries, or even scalar values. For example:
my_list = [1, 2, 3, 4] my_series = pd.Series(my_list)
The Series()
constructor accepts various parameters that help you customize the resulting series, including:
data
: This is the input data—arrays, dicts, or scalars.index
: You can provide a custom index for your series to label the values. If you don’t supply one, Pandas will automatically create an integer index (0, 1, 2…).
Here’s an example of creating a Series with a custom index:
custom_index = ['a', 'b', 'c', 'd'] my_series = pd.Series(my_list, index=custom_index)
When you create a Series object with a dictionary, Pandas automatically takes the keys as the index and the values as the series data:
my_dict = {'a': 1, 'b': 2, 'c': 3, 'd': 4} my_series = pd.Series(my_dict)
Remember: Your Series can hold various data types, including strings, numbers, and even objects.
Pandas Series Indexing

Next, you’ll learn the best ways to index and select data from a Pandas Series, making your data analysis tasks more manageable and enjoyable.
Again, a Pandas Series is a one-dimensional labeled array, and it can hold various data types like integers, floats, and strings. The series object contains an index, which serves multiple purposes, such as metadata identification, automatic and explicit data alignment, and intuitive data retrieval and modification .
There are two types of indexing available in a Pandas Series:
- Position-based indexing – this uses integer positions to access data. The pandas function
iloc[]
comes in handy for this purpose. - Label-based indexing – this uses index labels for data access. The pandas function
loc[]
works great for this type of indexing.

Recommended: Pandas
loc()
and iloc()
– A Simple Guide with Video
Let’s examine some examples of indexing and selection in a Pandas Series:
import pandas as pd # Sample Pandas Series data = pd.Series([10, 20, 30, 40, 50], index=['a', 'b', 'c', 'd', 'e']) # Position-based indexing (using iloc) position_index = data.iloc[2] # Retrieves the value at position 2 (output: 30) # Label-based indexing (using loc) label_index = data.loc['b'] # Retrieves the value with the label 'b' (output: 20)
Keep in mind that while working with Pandas Series, the index labels do not have to be unique but must be hashable types. This means they should be of immutable data types like strings, numbers, or tuples .
Recommended: Mutable vs. Immutable Objects in Python
Accessing Values in a Pandas Series
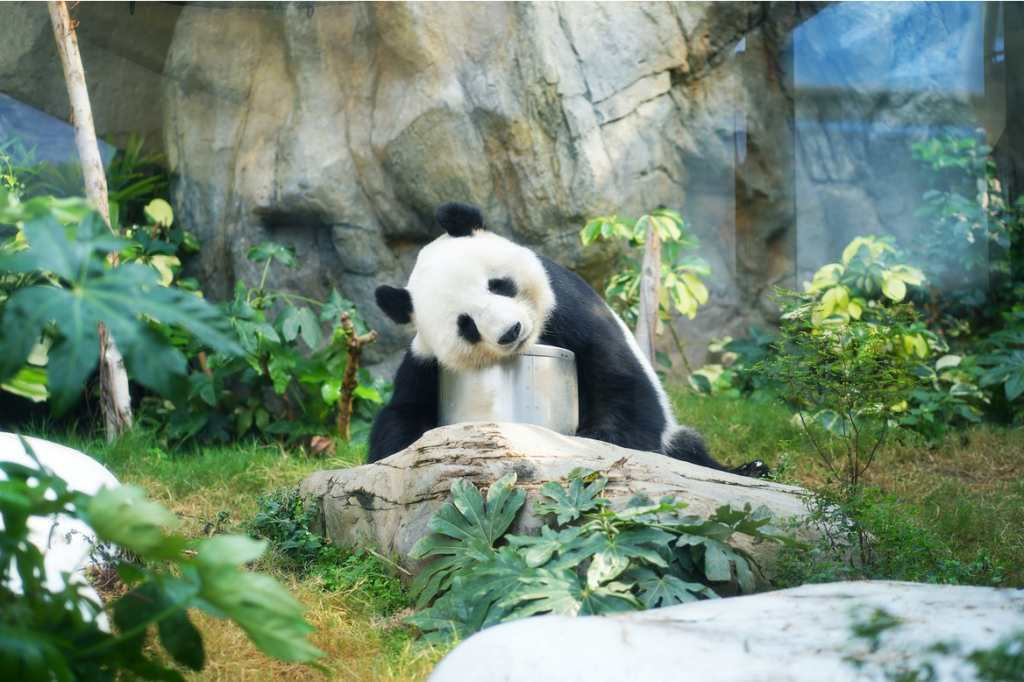
So you’re working with Pandas Series and want to access their values. I already showed you this in the previous section but let’s repeat this once again. Repetition. Repetition. Repetition!
First of all, create your Pandas Series:
import pandas as pd data = ['A', 'B', 'C', 'D', 'E'] my_series = pd.Series(data)
Now that you have your Series, let’s talk about accessing its values :
- Using index: You can access an element in a Series using its index, just like you do with lists:
third_value = my_series[2] print(third_value) # Output: C
- Using
.loc[]
: Access an element using its index label with the.loc[]
accessor, which is useful when you have custom index names:
data = ['A', 'B', 'C', 'D', 'E'] index_labels = ['one', 'two', 'three', 'four', 'five'] my_series = pd.Series(data, index=index_labels) second_value = my_series.loc['two'] print(second_value) # Output: B
- Using
.iloc[]
: Access a value based on its integer position with the.iloc[]
accessor. This is particularly helpful when you have non-integer index labels:
value_at_position_3 = my_series.iloc[2] print(value_at_position_3) # Output: C
Iterating through a Pandas Series

Although iterating over a Series is possible, it’s generally discouraged in the Pandas community due to its suboptimal performance. Instead, try using vectorization or other optimized methods, such as
apply
, transform
, or agg
.
This section will discuss Series iteration methods, but always remember to consider potential alternatives first!
When you absolutely need to iterate through a Series, you can use the iteritems()
function, which returns an iterator of index-value pairs. Here’s an example:
for idx, val in your_series.iteritems(): # Do something with idx and val
Another method to iterate over a Pandas Series is by converting it into a list using the tolist()
function, like this:
for val in your_series.tolist(): # Do something with val
However, keep in mind that these approaches are suboptimal and should be avoided whenever possible. Instead, try one of the following efficient techniques:
- Vectorized operations: Apply arithmetic or comparison operations directly on the Series.
- Use
apply()
: Apply a custom function element-wise. - Use
agg()
: Aggregate multiple operations to be applied. - Use
transform()
: Apply a function and return a similarly-sized Series.
Sorting a Pandas Series 
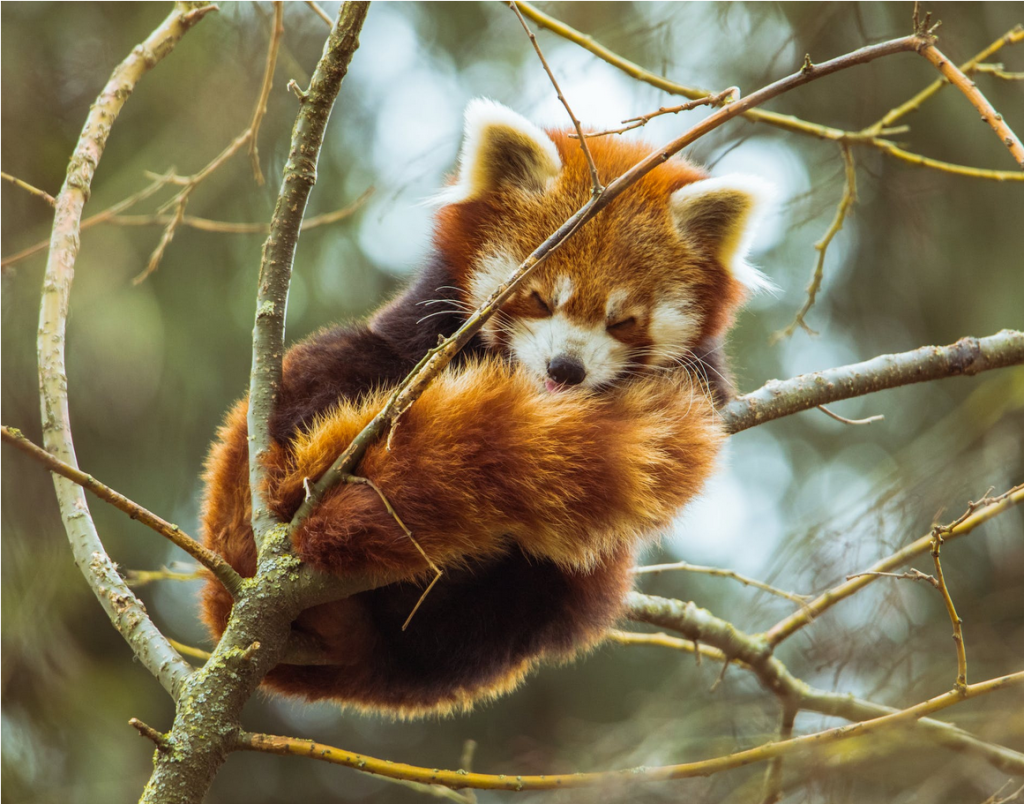
Sorting a Pandas Series is pretty straightforward. With the sort_values()
function, you can easily reorder your series, either in ascending or descending order.
First, you must import the Pandas library and create a Pandas Series:
import pandas as pd s = pd.Series([100, 200, 54.67, 300.12, 400])
To sort the values in the series, just use the sort_values()
function like this:
sorted_series = s.sort_values()
By default, the values will be sorted in ascending order. If you want to sort them in descending order, just set the ascending
parameter to False
:
sorted_series = s.sort_values(ascending=False)
You can also control the sorting method using the kind
parameter. Supported options are 'quicksort'
, 'mergesort'
, and 'heapsort'
. For example:
sorted_series = s.sort_values(kind='mergesort')
When dealing with missing values (NaN
) in your series, you can use the na_position
parameter to specify their position in the sorted series. The default value is 'last'
, which places missing values at the end.
To put them at the beginning of the sorted series, just set the na_position
parameter to 'first'
:
sorted_series = s.sort_values(na_position='first')
Applying Functions to a Pandas Series
You might come across situations where you want to apply a custom function to your Pandas Series. Let’s dive into how you can do that using the apply()
method.

To begin with, the apply()
method is quite flexible and allows you to apply a wide range of functions on your Series. These functions could be NumPy’s universal functions (ufuncs
), built-in Python functions, or user-defined functions. Regardless of the type, apply()
will work like magic.
For instance, let’s say you have a Pandas Series containing square numbers, and you want to find the square root of these numbers:
import pandas as pd square_numbers = pd.Series([4, 9, 16, 25, 36])
Now, you can use the apply()
method along with the built-in Python function sqrt()
to calculate the square root:
import math square_roots = square_numbers.apply(math.sqrt) print(square_roots)
You’ll get the following output:
0 2.0
1 3.0
2 4.0
3 5.0
4 6.0
dtype: float64
Great job! Now, let’s consider you want to create your own function to check if the numbers in a Series are even. Here’s how you can achieve that:
def is_even(number): return number % 2 == 0 even_numbers = square_numbers.apply(is_even) print(even_numbers)
And the output would look like this:
0 True
1 False
2 True
3 False
4 True
dtype: bool
Congratulations! You’ve successfully used the
apply()
method with a custom function.
Replacing Values in a Pandas Series
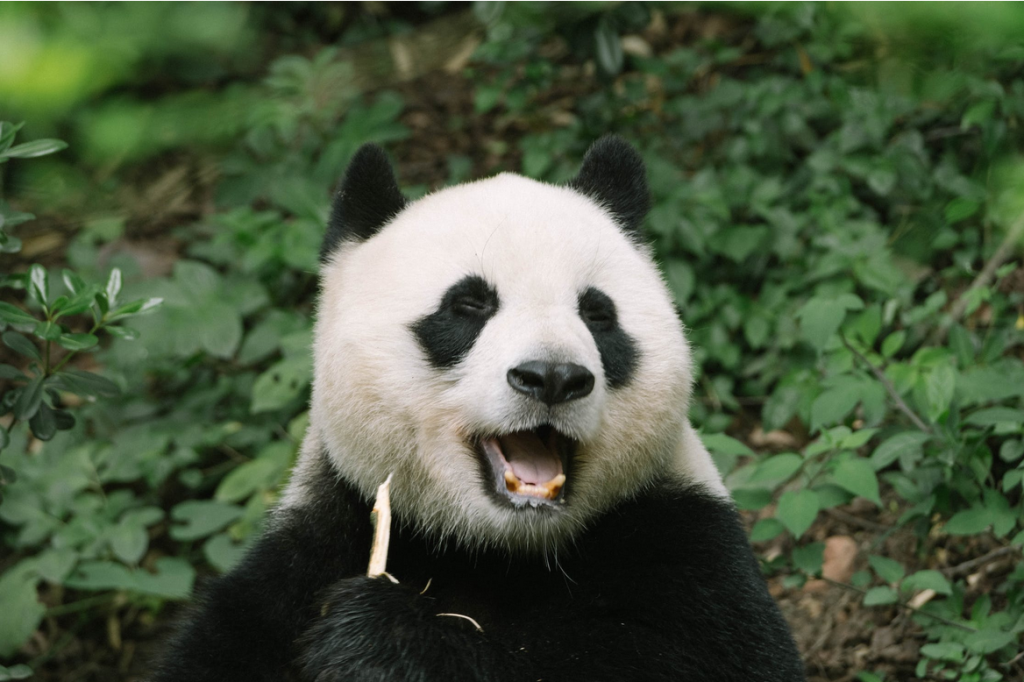
You might want to replace specific values within a Pandas Series to clean up your data or transform it into a more meaningful format. The replace()
function is here to help you do that!
How to use replace()
To use the replace()
function, simply call it on your Series object like this: your_series.replace(to_replace, value)
. to_replace
is the value you want to replace, and value
is the new value you want to insert instead. You can also use regex for more advanced replacements.
Let’s see an example:
import pandas as pd data = pd.Series([1, 2, 3, 4]) data = data.replace(2, "Two") print(data)
This code will replace the value 2
with the string "Two"
in your Series.
Multiple replacements
You can replace multiple values simultaneously by passing a dictionary or two lists to the function. For example:
data = pd.Series([1, 2, 3, 4]) data = data.replace({1: 'One', 4: 'Four'}) print(data)
In this case, 1
will be replaced with 'One'
and 4
with 'Four'
.
Limiting replacements
You can limit the number of replacements by providing the limit
parameter. For example, if you set limit=1
, only the first occurrence of the value will be replaced.
data = pd.Series([2, 2, 2, 2]) data = data.replace(2, "Two", limit=1) print(data)
This code will replace only the first occurrence of 2
with "Two"
in the Series.
Appending and Concatenating Pandas Series
You might want to combine your pandas Series while working with your data. Worry not! Pandas provides easy and convenient ways to append and concatenate your Series.
Appending Series
Appending Series can be done using the append()
method. It allows you to concatenate two or more Series objects. To use it, simply call the method on one series and pass the other series as the argument.
For example:
import pandas as pd series1 = pd.Series([1, 2, 3]) series2 = pd.Series([4, 5, 6]) result = series1.append(series2) print(result)
Output:
0 1
1 2
2 3
0 4
1 5
2 6
dtype: int64
However, appending Series iteratively may become computationally expensive. In such cases, consider using concat()
instead.
Concatenating Series
The concat()
function is more efficient when you need to combine multiple Series vertically. Simply provide a list of Series you want to concatenate as its argument, like so:
import pandas as pd series_list = [ pd.Series(range(1, 6), index=list('abcde')), pd.Series(range(1, 6), index=list('fghij')), pd.Series(range(1, 6), index=list('klmno')) ] combined_series = pd.concat(series_list) print(combined_series)
Output:
a 1
b 2
c 3
d 4
e 5
f 1
g 2
h 3
i 4
j 5
k 1
l 2
m 3
n 4
o 5
dtype: int64
There you have it! You’ve combined your Pandas Series using
append()
and concat()
.
Renaming a Pandas Series

Renaming a Pandas Series is a simple yet useful operation you may need in your data analysis process.
To start, the rename()
method in Pandas can be used to alter the index labels or name of a given Series object. But, if you just want to change the name of the Series, you can set the name
attribute directly. For instance, if you have a Series object called my_series
, you can rename it to "New_Name"
like this:
my_series.name = "New_Name"
Now, let’s say you want to rename the index labels of your Series. You can do this using the rename()
method. Here’s an example:
renamed_series = my_series.rename(index={"old_label1": "new_label1", "old_label2": "new_label2"})
The rename()
method also accepts functions for more complex transformations. For example, if you want to capitalize all index labels, you can do it like this:
capitalized_series = my_series.rename(index=lambda x: x.capitalize())
Keep in mind that the rename()
method creates a new Series by default and doesn’t modify the original one. If you want to change the original Series in-place, just set the inplace
argument to True
:
my_series.rename(index={"old_label1": "new_label1", "old_label2": "new_label2"}, inplace=True)
Unique Values in a Pandas Series
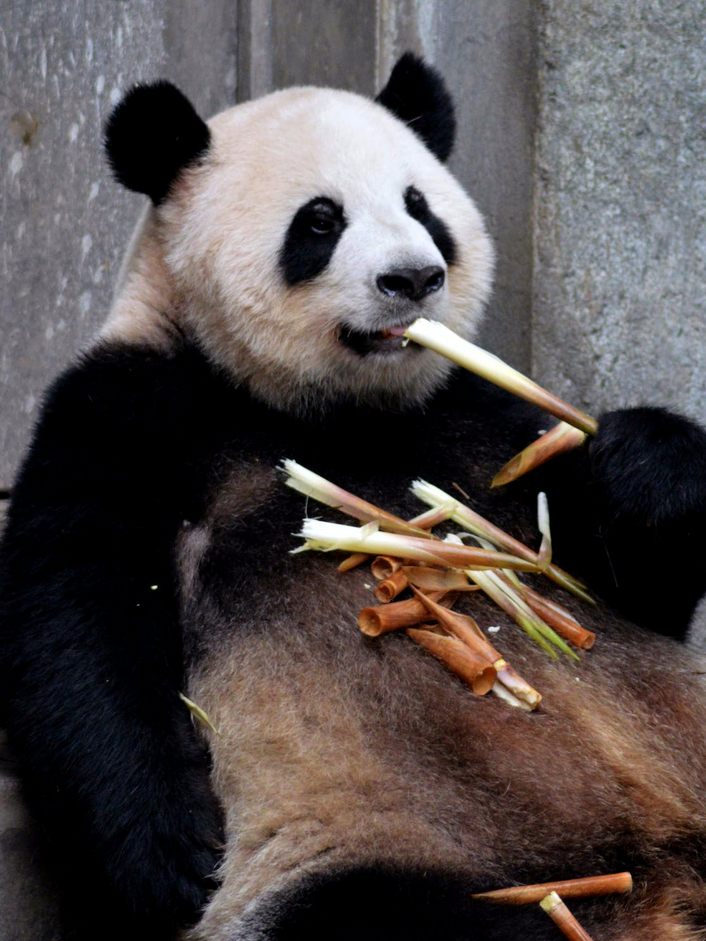
To find unique values in a Pandas Series, you can use the unique()
method. This method returns the unique values in the series without sorting them, maintaining the order of appearance.
Here’s a quick example:
import pandas as pd data = {'A': [1, 2, 1, 4, 5, 4]} series = pd.Series(data['A']) unique_values = series.unique() print(unique_values)
The output will be: [1, 2, 4, 5]
When working with missing values, keep in mind that the unique()
method includes NaN values if they exist in the series. This behavior ensures you are aware of missing data in your dataset .
If you need to find unique values in multiple columns, the unique()
method might not be the best choice, as it only works with Series objects, not DataFrames. Instead, use the .drop_duplicates()
method to get unique combinations of multiple columns.
Recommended: The Ultimate Guide to Data Cleaning in Python and Pandas
To summarize, when finding unique values in a Pandas Series:
- Use the
unique()
method for a single column - Remember that
NaN
values will be included as unique values when present - Use the
.drop_duplicates()
method for multiple columns when needed
With these tips, you’re ready to efficiently handle unique values in your Pandas data analysis!
Converting Pandas Series to Different Data Types
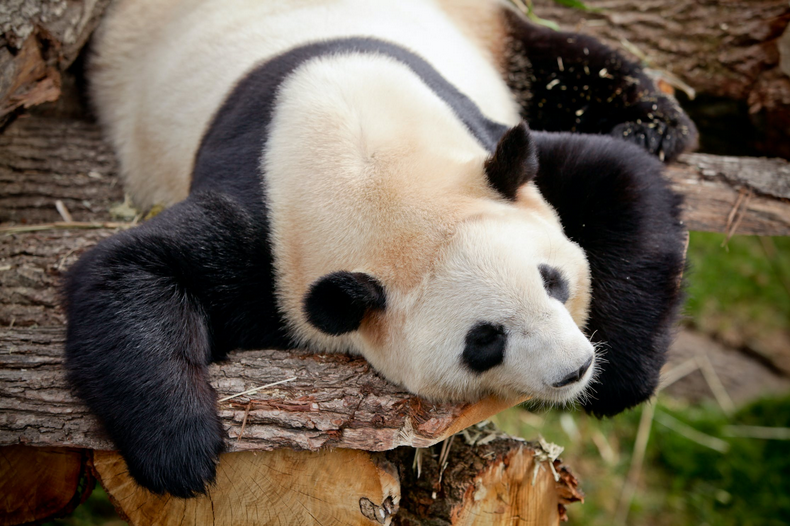
You can convert a Pandas Series to different data types to modify your data and simplify your work. In this section, you’ll learn how to transform a Series into a DataFrame, List, Dictionary, Array, String, and Numpy Array. Let’s dive in!
Series to DataFrame
To convert a Series to a DataFrame, use the to_frame()
method. Here’s how:
import pandas as pd data = pd.Series([1, 2, 3, 4]) df = data.to_frame() print(df)
This code will output:
0
0 1
1 2
2 3
3 4
Series to List
For transforming a Series to a List, simply call the tolist()
method, like this:
data_list = data.tolist() print(data_list)
Output:
[1, 2, 3, 4]
Series to Dictionary
To convert your Series into a Dictionary, use the to_dict()
method:
data_dict = data.to_dict() print(data_dict)
This results in:
{0: 1, 1: 2, 2: 3, 3: 4}
The keys are now indexes, and the values are the original Series data.
Series to Array
Convert your Series to an Array by accessing its .array
attribute:
data_array = data.array print(data_array)
Output:
<PandasArray> [1, 2, 3, 4]
Series to String
To join all elements of a Series into a single String, use the join()
function from the str
library:
data_str = ''.join(map(str, data)) print(data_str)
This will result in:
1234
Series to Numpy Array
For converting a Series into a Numpy Array, call the to_numpy()
method:
import numpy as np data_numpy = data.to_numpy() print(data_numpy)
Output:
array([1, 2, 3, 4], dtype=int64)
Now you’re all set to manipulate your Pandas Series objects and adapt them to different data types!
Python Pandas Series in Practice 

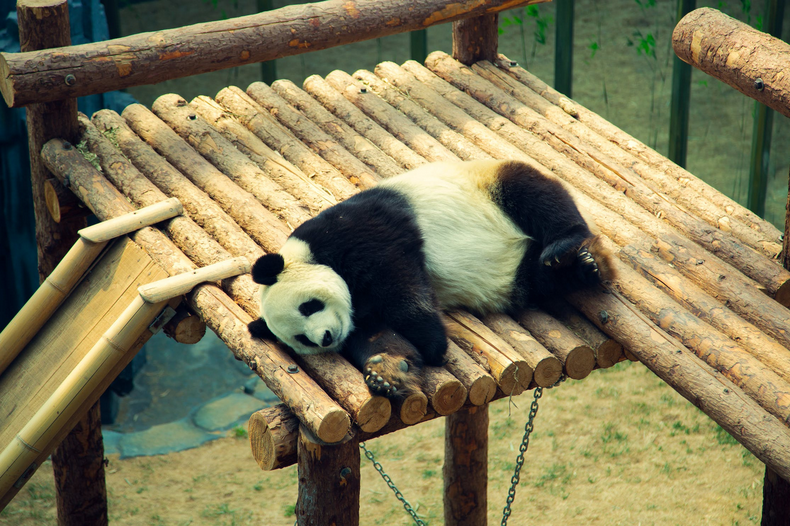
A Pandas Series is a one-dimensional array-like object that’s capable of holding any data type. It’s one of the essential data structures in the Pandas library, along with the DataFrame. Series is an easy way to organize and manipulate your data, especially when dealing with labeled data, such as SQL databases or dictionary keys.
To begin, import the Pandas library, which is usually done with the alias ‘pd
‘:
import pandas as pd
Creating a Pandas Series 

To create a Series, simply pass a list, ndarray, or dictionary to the pd.Series()
function. For example, you can create a Series with integers:
integer_series = pd.Series([1, 2, 3, 4, 5])
Or with strings:
string_series = pd.Series(['apple', 'banana', 'cherry'])
In case you want your Series to have an explicit index, you can specify the index
parameter:
indexed_series = pd.Series(['apple', 'banana', 'cherry'], index=['a', 'b', 'c'])
Accessing and Manipulating Series Data 

Now that you have your Series, here’s how you can access and manipulate the data:
- Accessing data by index (using both implicit and explicit index):
- First item:
integer_series[0]
orindexed_series['a']
- Slicing:
integer_series[1:3]
- First item:
- Adding new data:
- Append:
string_series.append(pd.Series(['date']))
- Add with a label:
indexed_series['d'] = 'date'
- Append:
- Common Series methods:
These are just a few examples of interacting with a Pandas Series. There are many other functionalities you can explore!
Practice makes perfect, so feel free to join our free email academy where I’ll show you practical coding projects, data science, exponential technologies in AI and blockchain engineering, Python, and much more. How can you join? Simply download your free cheat sheets by entering your name here:
Let your creativity run wild and happy coding!
May 01, 2023 at 12:03AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
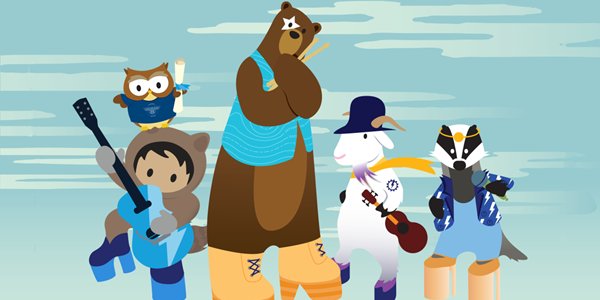
Post a Comment