Python for Beginners: Convert YAML to JSON in Python :
by:
blow post content copied from Planet Python
click here to view original post
JSON and YAML are the two most used file formats in software development. The YAML files are mainly used for configuration files whereas JSON files are used to store and transmit data. This article discusses how to convert YAML to JSON in Python.
What is YAML?
YAML is a human-readable data serialization language used for data storage and data exchange formats. It is often used for configuration files. But, we can also use it for data exchange between systems.
YAML uses indentation to denote structure and nested elements, instead of using brackets or tags as in XML or JSON. This makes YAML files more readable and easier to edit than other data serialization formats. In YAML, a list of items is denoted by a dash (-) followed by a space, and key-value pairs are separated by a colon (:) followed by a space. YAML also supports comments, which can be added using the “#” symbol.
YAML supports various data types, including strings, integers, floating-point numbers, booleans, and arrays. It also supports nested structures, allowing for the creation of complex data structures. This makes YAML suitable for a wide range of use cases, from simple configurations to more complex data structures.
Following is a sample YAML file. It contains the details of an employee.
employee:
name: John Doe
age: 35
job:
title: Software Engineer
department: IT
years_of_experience: 10
address:
street: 123 Main St.
city: San Francisco
state: CA
zip: 94102
In the above YAML file,
- The
employee
attribute has four nested attributes i.e.name
,age
,job
, andaddress
. - The
name
andage
attributes contain the name and age of the employee. - The
job
attribute has three nested attributes i.e.title
,department
, andyears_of_experience
. - Similarly, the
address
attribute has four inner attributes namelystreet
,city
,state
, andzip
.
Hence, you can observe that we can easily represent complex data with multiple levels of hierarchy using the YAML format.
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is used for exchanging data between systems. It is a text-based format that based on a subset of the JavaScript programming language. JSON is widely used for storing and exchanging data on the web, as well as for creating APIs (Application Programming Interfaces) that allow different systems to communicate with each other.
The basic structure of JSON data consists of key-value pairs, where keys are used to identify data elements, and values can be of various data types, including strings, numbers, booleans, arrays, and objects.
In JSON, data is enclosed in curly braces ({}) and separated by commas, while arrays are enclosed in square brackets ([]). JSON also supports comments, but they are not commonly used due to their limited support in various programming languages and applications.
We can represent the data shown in the previous section using JSON format as shown below.
{
"employee": {
"name": "John Doe",
"age": 35,
"job": {
"title": "Software Engineer",
"department": "IT",
"years_of_experience": 10
},
"address": {
"street": "123 Main St.",
"city": "San Francisco",
"state": "CA",
"zip": 94102
}
}
}
JSON data is lightweight and easy to parse, making it a popular choice for exchanging data on the web. It is also easily supported by many programming languages, including JavaScript, Python, Ruby, PHP, and Java, making it a versatile and universal format for exchanging data between different systems.
YAML String to JSON String in Python
To convert a YAML string to a JSON string, we will use the following steps.
- First, we will import the json and yaml modules using the import statement.
- Next, we will convert the YAML string to a python dictionary using the
load()
method defined in the yaml module. Theload()
method takes the yaml string as its first input argument and the loader type in itsLoader
argument. After execution, it returns a python dictionary. - After obtaining the dictionary, we can convert it to a json string using the
dumps()
method defined in the json module. Thedumps()
method takes the python dictionary as its input argument and returns the json string.
You can observe this in the following example.
import json
import yaml
from yaml import SafeLoader
yaml_string="""employee:
name: John Doe
age: 35
job:
title: Software Engineer
department: IT
years_of_experience: 10
address:
street: 123 Main St.
city: San Francisco
state: CA
zip: 94102
"""
print("The YAML string is:")
print(yaml_string)
python_dict=yaml.load(yaml_string, Loader=SafeLoader)
json_string=json.dumps(python_dict)
print("The JSON string is:")
print(json_string)
Output:
The YAML string is:
employee:
name: John Doe
age: 35
job:
title: Software Engineer
department: IT
years_of_experience: 10
address:
street: 123 Main St.
city: San Francisco
state: CA
zip: 94102
The JSON string is:
{"employee": {"name": "John Doe", "age": 35, "job": {"title": "Software Engineer", "department": "IT", "years_of_experience": 10}, "address": {"street": "123 Main St.", "city": "San Francisco", "state": "CA", "zip": 94102}}}
In this example, we have converted a YAML string to JSON string.
YAML string to JSON file
Instead of obtaining a json string, you can also convert a yaml string to a json file. For this, we will use the following steps.
- First, we will convert the yaml string to a python dictionary using the
load()
method defined in the yaml module. - Next, we will open a json file in write mode using the
open()
function. Theopen()
function takes the file name as its first input argument and the python literal “w
” as its second input argument. After execution, it returns the file pointer. - After opening the file, we will convert the python dictionary obtained from the yaml string into the json file. For this, we will use the
dump()
method defined in the json module. Thedump()
method takes the python dictionary as its first input argument and the file pointer returned by theopen()
function as the second input argument. - After execution of the
dump()
method, the json file will be saved on your machine. Then, we will close the json file using theclose()
method.
You can observe this in the following example.
import json
import yaml
from yaml import SafeLoader
yaml_string="""employee:
name: John Doe
age: 35
job:
title: Software Engineer
department: IT
years_of_experience: 10
address:
street: 123 Main St.
city: San Francisco
state: CA
zip: 94102
"""
print("The YAML string is:")
print(yaml_string)
python_dict=yaml.load(yaml_string, Loader=SafeLoader)
file=open("person_details.json","w")
json.dump(python_dict,file)
file.close()
print("JSON file saved")
Output:
The YAML string is:
employee:
name: John Doe
age: 35
job:
title: Software Engineer
department: IT
years_of_experience: 10
address:
street: 123 Main St.
city: San Francisco
state: CA
zip: 94102
JSON file saved
In this example, we have converted the YAML string to a JSON file using json and yaml modules in python. The output JSON file looks as follows.
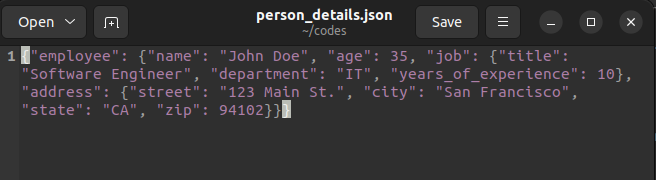
Convert YAML file to JSON string
We can also convert a yaml file to a json string. For this, we will use the following steps.
- First, we will open the yaml file in read mode using the
open()
function. Theopen()
function takes the file name of the yaml file as its first input argument and the python literal “r” as its second argument. After execution, it returns a file pointer. - Next, we will obtain the python dictionary from the yaml file using the
load()
method. Theload()
method takes the file pointer as its first input argument and the loader type in itsLoader
argument. After execution, it returns a dictionary. - After obtaining the dictionary, we will convert it to JSON string using the
dumps()
method defined in the json module. Thedumps()
method takes the python dictionary as its input argument and returns the json string.
You can observe this in the following example.
import json
import yaml
from yaml import SafeLoader
yaml_file=open("person_details.yaml","r")
python_dict=yaml.load(yaml_string, Loader=SafeLoader)
json_string=json.dumps(python_dict)
print("The JSON string is:")
print(json_string)
Output:
The JSON string is:
{"employee": {"name": "John Doe", "age": 35, "job": {"title": "Software Engineer", "department": "IT", "years_of_experience": 10}, "address": {"street": "123 Main St.", "city": "San Francisco", "state": "CA", "zip": 94102}}}
In this example, we have converted a YAML file to JSON string.
Convert YAML File to JSON File in Python
Instead of converting it into the JSON string, we can also convert the YAML file into a JSON file. For this, we will use the following steps.
- First, we will obtain the python dictionary from the yaml file using the
open()
function and theload()
method. - Then, we will open a json file in write mode using the
open()
function. Theopen()
function takes the file name as its first input argument and the literal “w” as its second input argument. After execution, it returns the file pointer. - After opening the file, we will convert the python dictionary obtained from the yaml file into the json file. For this, we will use the
dump()
method defined in the json module. Thedump()
method takes the python dictionary as its first input argument and the file pointer returned by theopen()
function as its second input argument. - After execution of the
dump()
method, the json file will be saved on your machine. Then, we will close the json file using theclose()
method.
You can observe the entire process in the following example.
import json
import yaml
from yaml import SafeLoader
yaml_file=open("person_details.yaml","r")
python_dict=yaml.load(yaml_string, Loader=SafeLoader)
file=open("person_details1.json","w")
json.dump(python_dict,file)
file.close()
print("JSON file saved")
After execution of the above code, the YAML file person_details.yaml is saved as JSON into the file person_details1.json.
Conclusion
In this article, we have discussed how to convert a yaml string or file to json format.
To learn more about python programming, you can read this article on how to convert JSON to YAML in Python. You might also like this article on custom json encoders in python.
I hope you enjoyed reading this article. Stay tuned for more informative articles.
Happy Learning!
The post Convert YAML to JSON in Python appeared first on PythonForBeginners.com.
February 08, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
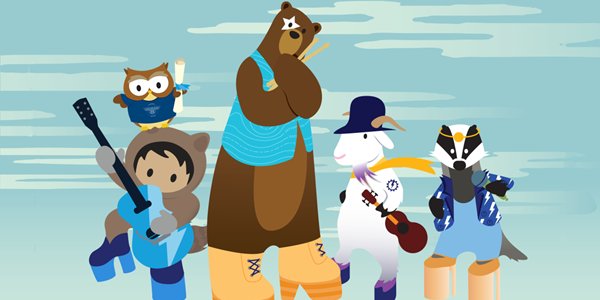
Post a Comment