Python for Beginners: Convert JSON to YAML in Python :
by:
blow post content copied from Planet Python
click here to view original post
Different software uses different file formats for storing and transmitting data. Hence, we often need to convert data from one format to another. This article will discuss how we can convert JSON to YAML in python.
What is JSON Format?
JSON (JavaScript Object Notation) is a lightweight data interchange format. It is easy for humans to read and write and for machines to parse and generate. The JSON format is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition – December 1999.
In JSON, attributes and their values are represented as key-value pairs just like a python dictionary. The keys and values are separated by a colon and enclosed in curly braces. Nested attributes are represented as nested key-value pairs. The data structure is easy to read and understand, making JSON a popular format for data exchange and storage.
A simple JSON file with nested attributes looks as follows.
{
"employee": {
"name": "John Doe",
"age": 35,
"job": {
"title": "Software Engineer",
"department": "IT",
"years_of_experience": 10
},
"address": {
"street": "123 Main St.",
"city": "San Francisco",
"state": "CA",
"zip": 94102
}
}
}
In this example, the employee attribute has several nested attributes, such as name, age, job, and address. The job attribute has further nested attributes, including title, department, and years_of_experience.
What is YAML Format?
YAML (Yet Another Markup Language) is a human-readable data serialization language used to store and transmit data. It is commonly used for configuration files, data exchange between languages and platforms, and software development.
We can represent the JSON data shown in the previous example using YAML as shown below.
employee:
name: John Doe
age: 35
job:
title: Software Engineer
department: IT
years_of_experience: 10
address:
street: 123 Main St.
city: San Francisco
state: CA
zip: 94102
The YAML syntax uses indentation to show the relationship between the attributes, making it easy to understand the structure of the data.
Convert JSON string to YAML string in Python
To convert a JSON string to a YAML string, we will first convert the json string to a python dictionary using the loads()
method defined in the json module. The loads()
method takes a json string as its input argument and returns a python dictionary after execution.
Once we get the dictionary, we can convert it to a YAML string using the dump()
method defined in the yaml module. The dump()
method takes the python dictionary as its input argument and returns the YAML string as shown in the following example.
import json
import yaml
json_string='{"name": {"first": "John", "last": "Doe"}, "address": {"street": "123 Main St", "city": "Anytown", "state": "CA", "zipcode": "12345"}, "email": "[email protected]", "age": 32}'
print("The JSON string is:")
print(json_string)
python_dict=json.loads(json_string)
ymal_string=yaml.dump(python_dict)
print("The YAML string is:")
print(ymal_string)
Output:
The JSON string is:
{"name": {"first": "John", "last": "Doe"}, "address": {"street": "123 Main St", "city": "Anytown", "state": "CA", "zipcode": "12345"}, "email": "[email protected]", "age": 32}
The YAML string is:
address:
city: Anytown
state: CA
street: 123 Main St
zipcode: '12345'
age: 32
email: [email protected]
name:
first: John
last: Doe
You can also convert a JSON string to a YAML file. For this, you can use the dump()
method defined in the yaml module as shown below.
import json
import yaml
json_string='{"name": {"first": "John", "last": "Doe"}, "address": {"street": "123 Main St", "city": "Anytown", "state": "CA", "zipcode": "12345"}, "email": "[email protected]", "age": 32}'
print("The JSON string is:")
print(json_string)
python_dict=json.loads(json_string)
file=open("person_data.yaml","w")
yaml.dump(python_dict,file)
file.close()
print("YAML file saved.")
Output:
The JSON string is:
{"name": {"first": "John", "last": "Doe"}, "address": {"street": "123 Main St", "city": "Anytown", "state": "CA", "zipcode": "12345"}, "email": "[email protected]", "age": 32}
YAML file saved.
In this example, we first opened a YAML file in write mode. Then, we passed the file pointer to the dump()
method as its second input argument. Hence, the dump()
method saves the data into the files instead of returning a string.
After execution of the above code, the output file looks as follows.
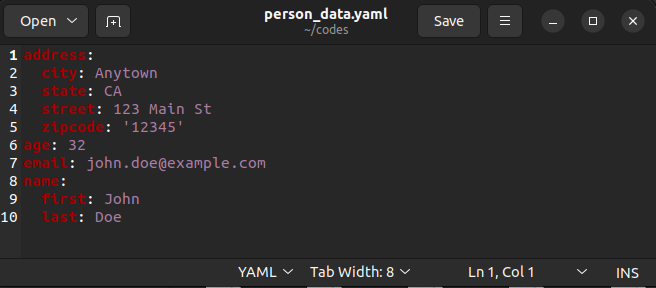
Convert JSON file to the YAML format
To convert a JSON file to YAML, we will use the following steps.
- First, we will open the JSON file in read mode using the
open()
function. Theopen()
function takes the file name of the json file as its input argument and returns a file pointer. - Next, we will load the json file to a python dictionary using the
load()
method defined in the json module. Theload()
method takes the file pointer of the json file as its input argument and returns a python dictionary. - Once we get the dictionary, we can convert it to a YAML string using the
dump()
method defined in the yaml module. Thedump()
method takes the python dictionary as its input argument and returns the YAML string.
You can observe this in the following example.
import json
import yaml
file=open("person_data.json","r")
python_dict=json.load(file)
yaml_string=yaml.dump(python_dict)
print("The YAML file is:")
print(yaml_string)
Output:
The YAML file is:
address:
city: Anytown
state: CA
street: 123 Main St
zipcode: '12345'
age: 32
email: [email protected]
name:
first: John
last: Doe
To convert a JSON file to a YAML file, you can use the dump()
method as shown below.
import json
import yaml
file=open("person_data.json","r")
python_dict=json.load(file)
file1=open("person_data1.yaml","w")
yaml.dump(python_dict,file1)
file1.close()
print("YAML file saved.")
Output:
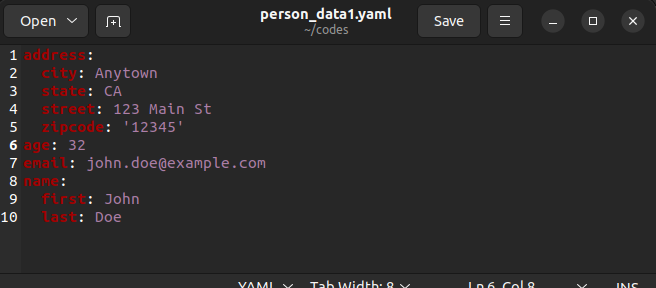
JSON to YAML Online
To convert JSON to YAML online, you can use jsontoyaml.com.
Conclusion
In this article, we have discussed different ways to convert a JSON file or string to YAML in python. To learn more about python programming, you can read this article on how to convert a dictionary to json in Python. You might also like this article on working with JSON files in Python.
I hope you enjoyed reading this article. Stay tuned for more informative articles.
Happy Learning!
The post Convert JSON to YAML in Python appeared first on PythonForBeginners.com.
February 03, 2023 at 07:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
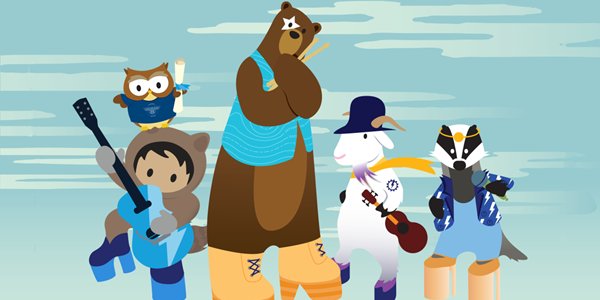
Post a Comment