Python for Beginners: Convert CSV to HTML Table in Python :
by:
blow post content copied from Planet Python
click here to view original post
CSV files contain comma-separated values that usually contain tabular. Sometimes, we might need to render a csv file into an HTML page. In this article, we will discuss how we can convert a csv file to an HTML table in python.
Convert CSV to HTML Table Using the pandas Module
The pandas module provides us with different tools to handle csv files. To convert a csv file to an HTML table, we will first open the file using the read_csv()
method. The read_csv()
method takes the file name of the csv file as an input argument and returns a dataframe containing the data from the csv file.
After obtaining the data from the csv file into the dataframe, we can convert the dataframe into an HTML string using the to_html()
method. The to_html()
method, when invoked on a dataframe, converts the dataframe into an HTML table and returns the HTML text in the form of a string. You can observe this in the following example.
import pandas as pd
df1 = pd.read_csv('student_details.csv')
print("The dataframe is:")
print(df1)
html_string = df1.to_html()
print("The html string is:")
print(html_string)
Output:
The dataframe is:
Name Roll Number Subject
0 Aditya 12 Python
1 Sam 23 Java
2 Chris 11 C++
3 Joel 10 JavaScript
4 Mayank 5 Typescript
The html string is:
<table border="1" class="dataframe">
<thead>
<tr style="text-align: right;">
<th></th>
<th>Name</th>
<th>Roll Number</th>
<th>Subject</th>
</tr>
</thead>
<tbody>
<tr>
<th>0</th>
<td>Aditya</td>
<td>12</td>
<td>Python</td>
</tr>
<tr>
<th>1</th>
<td>Sam</td>
<td>23</td>
<td>Java</td>
</tr>
<tr>
<th>2</th>
<td>Chris</td>
<td>11</td>
<td>C++</td>
</tr>
<tr>
<th>3</th>
<td>Joel</td>
<td>10</td>
<td>JavaScript</td>
</tr>
<tr>
<th>4</th>
<td>Mayank</td>
<td>5</td>
<td>Typescript</td>
</tr>
</tbody>
</table>
You can also save the data directly into an HTML file. For this, you have to pass the filename as an input argument to the to_html()
method. The to_html()
method, when invoked on a dataframe, takes the file name of the HTML file as an input argument and saves it in the current working directory. After execution, the to_html()
method returns None in this case. You can observe this in the following example.
import pandas as pd
df1 = pd.read_csv('student_details.csv')
print("The dataframe is:")
print(df1)
df1.to_html("html_output.html")
print("CSV file saved into html file.")
Output:
The dataframe is:
Name Roll Number Subject
0 Aditya 12 Python
1 Sam 23 Java
2 Chris 11 C++
3 Joel 10 JavaScript
4 Mayank 5 Typescript
CSV file saved into html file.
Following is the snapshot of the html table created using the above program.
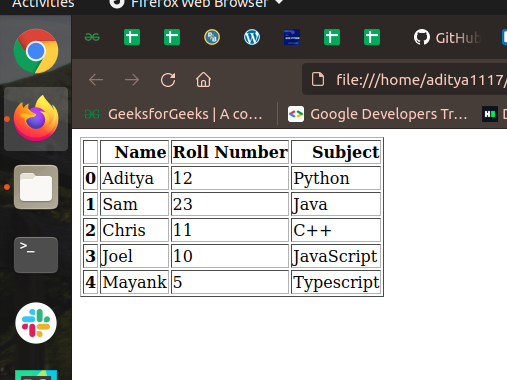
Convert CSV to HTML Table Using the PrettyTable Module
We can also convert a csv file into an HTML file using the PrettyTable()
method. For this, we will first open the csv file using the open()
method in the read mode. The open()
method takes the filename as its first input argument and the literal ‘r’ as its second input argument. After execution, it returns a file object containing the file content.
After opening the file, we will read the file content using the readlines()
method. The readlines()
method, when invoked on a file object, returns the contents of the file as a list of strings where each element of the list contains a line from the input file.
Now, the header of the csv file will be present at the index 0 in the list returned by the readlines()
method. We will extract the name of the columns of the csv files using the string split operation on the first element of the list returned by the readlines()
method.
After obtaining the column names in a list, we will create a pretty table using the PrettyTable
() method. The PrettyTable()
method takes a list containing the column names as its input argument and returns a pretty table. After creating the table, we will add the data to the table using the add_row()
method. The add_row()
method takes a list containing the values in a row and adds it to the pretty table.
After creating the table, we will obtain the HTML string of the table using the get_html_string()
method. The get_html_string()
method, when invoked on a pretty table object, returns the HTML text of the table in the form of a string.
You can observe this entire process in the following example.
import prettytable
csv_file = open('student_details.csv', 'r')
data = csv_file.readlines()
column_names = data[0].split(',')
table = prettytable.PrettyTable()
table.add_row(column_names)
for i in range(1, len(data)):
row = data[i].split(",")
table.add_row(row)
html_string = table.get_html_string()
print("The html string obtained from the csv file is:")
print(html_string)
Output:
The html string obtained from the csv file is:
<table>
<thead>
<tr>
<th>Field 1</th>
<th>Field 2</th>
<th>Field 3</th>
</tr>
</thead>
<tbody>
<tr>
<td>Name</td>
<td>Roll Number</td>
<td> Subject<br></td>
</tr>
<tr>
<td>Aditya</td>
<td> 12</td>
<td> Python<br></td>
</tr>
<tr>
<td>Sam</td>
<td> 23</td>
<td> Java<br></td>
</tr>
<tr>
<td>Chris</td>
<td> 11</td>
<td> C++<br></td>
</tr>
<tr>
<td>Joel</td>
<td> 10</td>
<td> JavaScript<br></td>
</tr>
<tr>
<td>Mayank</td>
<td> 5</td>
<td> Typescript</td>
</tr>
</tbody>
</table>
Conclusion
In this article, we have discussed how to convert a csv file to an HTML file in python. To know more about python programming, you can read this article on list comprehension in python. You might also like this article on dictionary comprehension in python.
The post Convert CSV to HTML Table in Python appeared first on PythonForBeginners.com.
July 15, 2022 at 06:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
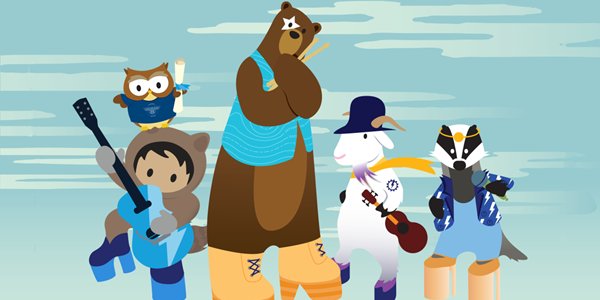
Post a Comment