Python's min() and max(): Find Smallest and Largest Values :
by:
blow post content copied from Real Python
click here to view original post
Python’s built-in functions max()
and min()
allow you to find the largest and smallest values in a dataset. You can use them with iterables, such as lists or tuples, or a series of regular arguments. They can handle numbers, strings, and even dictionaries. Plus, with the optional arguments key
and default
, you can customize their behavior to suit your needs.
By the end of this tutorial, you’ll understand that:
- Python’s
max()
andmin()
can find the largest and smallest values in a dataset. min()
andmax()
can handle string inputs by comparing their alphabetical order.- The
key
argument modifies comparison criteria by applying a function to each element before comparison. - You can use
min()
andmax()
with generator expressions for memory-efficient value comparison.
This tutorial explores the practical use cases for min()
and max()
, such as removing outliers from lists and processing strings. By the end, you’ll also know how to implement your own versions of min()
and max()
to deepen your understanding of these functions.
Free Bonus: 5 Thoughts On Python Mastery, a free course for Python developers that shows you the roadmap and the mindset you’ll need to take your Python skills to the next level.
To get the most out of this tutorial, you should have some previous knowledge of Python programming, including topics like for
loops, functions, list comprehensions, and generator expressions.
Getting Started With Python’s min()
and max()
Functions
Python includes several built-in functions that make your life more pleasant and productive because they mean you don’t need to reinvent the wheel. Two examples of these functions are min()
and max()
. They mostly apply to iterables, but you can use them with multiple regular arguments as well. What’s their job? They take care of finding the smallest and largest values in their input data.
Whether you’re using Python’s min()
or max()
, you can use the function to achieve two slightly different behaviors. The standard behavior for each is to return the minimum or maximum value through straightforward comparison of the input data as it stands. The alternative behavior is to use a single-argument function to modify the comparison criteria before finding the smallest and largest values.
To explore the standard behavior of min()
and max()
, you can start by calling each function with either a single iterable as an argument or with two or more regular arguments. That’s what you’ll do right away.
Calling min()
and max()
With a Single Iterable Argument
The built-in min()
and max()
have two different signatures that allow you to call them either with an iterable as their first argument or with two or more regular arguments. The signature that accepts a single iterable argument looks something like this:
min(iterable, *[, default, key]) -> minimum_value
max(iterable, *[, default, key]) -> maximum_value
Both functions take a required argument called iterable
and return the minimum and maximum values respectively. They also take two optional keyword-only arguments: default
and key
.
Note: In the above signatures, the asterisk (*
) means that the following arguments are keyword-only arguments, while the square brackets ([]
) denote that the enclosed content is optional.
Here’s a summary of what the arguments to min()
and max()
do:
Argument | Description | Required |
---|---|---|
iterable |
Takes an iterable object, like a list, tuple, dictionary, or string | Yes |
default |
Holds a value to return if the input iterable is empty | No |
key |
Accepts a single-argument function to customize the comparison criteria | No |
Later in this tutorial, you’ll learn more about the optional default
and key
arguments. For now, just focus on the iterable
argument, which is a required argument that leverages the standard behavior of min()
and max()
in Python:
>>> min([3, 5, 9, 1, -5])
-5
>>> min([])
Traceback (most recent call last):
...
ValueError: min() arg is an empty sequence
>>> max([3, 5, 9, 1, -5])
9
>>> max([])
Traceback (most recent call last):
...
ValueError: max() arg is an empty sequence
In these examples, you call min()
and max()
with a list of integer numbers and then with an empty list. The first call to min()
returns the smallest number in the input list, -5
. In contrast, the first call to max()
returns the largest number in the list, or 9
. If you pass an empty iterator to min()
or max()
, then you get a ValueError
because there’s nothing to do on an empty iterable.
An important detail to note about min()
and max()
is that all the values in the input iterable must be comparable. Otherwise, you get an error. For example, numeric values work okay:
>>> min([3, 5.0, 9, 1.0, -5])
-5
>>> max([3, 5.0, 9, 1.0, -5])
9
These examples combine int
and float
numbers in the calls to min()
and max()
. You get the expected result in both cases because these data types are comparable.
However, what would happen if you mixed strings and numbers? Check out the following examples:
>>> min([3, "5.0", 9, 1.0, "-5"])
Traceback (most recent call last):
...
TypeError: '<' not supported between instances of 'str' and 'int'
>>> max([3, "5.0", 9, 1.0, "-5"])
Traceback (most recent call last):
...
TypeError: '>' not supported between instances of 'str' and 'int'
Read the full article at https://realpython.com/python-min-and-max/ »
[ Improve Your Python With 🐍 Python Tricks 💌 – Get a short & sweet Python Trick delivered to your inbox every couple of days. >> Click here to learn more and see examples ]
January 18, 2025 at 07:30PM
Click here for more details...
=============================
The original post is available in Real Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
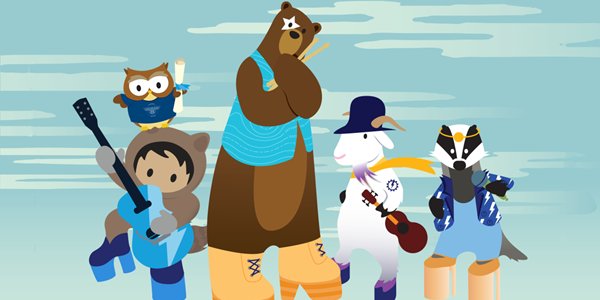
Post a Comment