5 Best Ways to Compress CSV Files to GZIP in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: How can we efficiently compress CSV files into GZIP format using Python? This task is common when dealing with large volumes of data that need to be stored or transferred. For instance, we may want to compress a file named
'data.csv'
into a GZIP file named 'data.csv.gz'
to save disk space or to minimize network transfer time.
Method 1: Using pandas with to_csv and compression Parameters
Pandas is a powerful data manipulation library in Python that includes methods for both reading and writing CSV files. It offers a simple way to compress a CSV file directly to GZIP by specifying the compression='gzip'
parameter in the to_csv
method. This method is concise and utilizes pandas’ robust data handling capabilities.
Here’s an example:
import pandas as pd # Create a DataFrame df = pd.DataFrame({'A': range(1, 6), 'B': range(10, 15)}) # Compress and save to 'data.csv.gz' df.to_csv('data.csv.gz', index=False, compression='gzip')
The output will be a GZIP file containing the data from the DataFrame, saved in the specified location.
This code snippet first creates a DataFrame using pandas
, then writes it to a GZIP compressed file with the to_csv
method, specifying compression='gzip'
. It’s succinct, takes advantage of the powerful pandas
ecosystem, and is ideal for those who are already processing their data using pandas
.
Method 2: Using csv and gzip Standard Libraries
The csv
and gzip
modules from Python’s standard libraries can be used together to compress CSV data into GZIP format. This method is valuable for those who prefer not to use third-party libraries such as pandas and require a more granular level of control over reading and writing the CSV files.
Here’s an example:
import csv import gzip with open('data.csv', 'rt') as csv_file: with gzip.open('data.csv.gz', 'wt') as gzip_file: writer = csv.writer(gzip_file) reader = csv.reader(csv_file) for row in reader: writer.writerow(row)
The output is the ‘data.csv’ content written into a compressed GZIP file ‘data.csv.gz’.
This example reads the CSV file line by line using the csv.reader
, and writes each row to a GZIP file using the gzip.open
method. This approach gives the user direct control over the file handling process and avoids any dependencies beyond Python’s standard library.
Method 3: Using shutil and gzip Modules
The shutil
module provides a higher-level operation interface such as file copying and removal. By partnering with the gzip
module, one can read a CSV file and write its content in a compressed format effortlessly, especially when no manipulation of data is required.
Here’s an example:
import gzip import shutil with open('data.csv', 'rb') as f_in: with gzip.open('data.csv.gz', 'wb') as f_out: shutil.copyfileobj(f_in, f_out)
The resulting output is a GZIP file ‘data.csv.gz’ that contains the compressed contents of ‘data.csv’.
This code snippet uses shutil.copyfileobj
to copy the contents of an open file object to another file object. The gzip.open
function is used to create the file object in binary write mode, resulting in writing a compressed file effortlessly.
Method 4: Using subprocess to Call External gzip Command
For systems where the UNIX gzip
utility is available, Python’s subprocess
module can be used to execute a shell command. This method is convenient when working within environments that have gzip
installed and one needs to quickly compress a file without Python-specific tools.
Here’s an example:
import subprocess # Call external gzip command subprocess.run(['gzip', 'data.csv'])
The output of this operation is that ‘data.csv’ is replaced by a compressed ‘data.csv.gz’ file in the same directory.
This snippet works by using the subprocess.run()
method to invoke the gzip
command on the CSV file. Note that running external commands can be riskier than using pure Python solutions, as it relies on the shell environment and command’s availability.
Bonus One-Liner Method 5: Streamlining Compression with Pandas and gzip
Combining the simplicity of pandas with the standard gzip
module, one can streamline the CSV compression process into a one-liner. The DataFrame is converted to CSV format and directly compressed into a GZIP stream.
Here’s an example:
import pandas as pd import gzip # Alternative one-liner using pandas and gzip pd.DataFrame({'A': range(1, 6), 'B': range(10, 15)}).to_csv(gzip.open('data.csv.gz', 'wt'), index=False)
This one-liner creates and compresses a DataFrame into ‘data.csv.gz’ without intermediate steps.
The power of this one-liner lies in its brevity and integration of pandas
with gzip
. It does the same job as Method 1, but is even more streamlined, suited for quick execution with minimal code.
Summary/Discussion
- Method 1: Pandas to_csv. Strengths: Intuitive and concise, utilizes pandas’ powerful data handling. Weaknesses: Requires pandas library, an additional dependency.
- Method 2: csv and gzip Libraries. Strengths: Uses Python’s standard library for full control over the process. Weaknesses: More verbose, requires manual handling of files.
- Method 3: shutil and gzip Modules. Strengths: Provides a high-level interface for file operations, simple and direct. Weaknesses: Not suitable for line-by-line file processing or data manipulation.
- Method 4: Subprocess gzip Command. Strengths: Utilizes system-level gzip for potentially faster compression. Weaknesses: Depends on external utilities, less portable, and riskier due to shell invocation.
- Method 5: One-Liner Pandas and gzip. Strengths: Quick and concise, ideal for simple compression tasks. Weaknesses: Still requires pandas dependency and offers no access to intermediate steps.
March 02, 2024 at 03:41AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
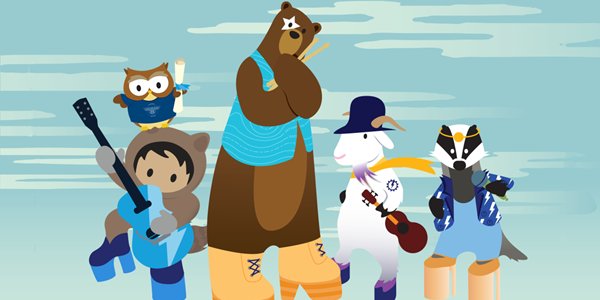
Post a Comment