5 Best Ways to Append to a CSV Column in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: When working with CSV files in Python, you may encounter scenarios where you need to append data to a specific column without altering the rest of the file. This can be useful for logging new information, updating records, or simply expanding your dataset. Supposing you have an input CSV with columns “Name,” “Age,” and “Occupation,” and you would like to append a list of email addresses to a new “Email” column; this article will guide you through multiple methods to achieve this.
Method 1: Using the csv module to rewrite the file
The csv module in Python is a robust tool for reading and writing CSV files. This method involves reading the original CSV file into memory, appending the new column data, and writing the updated data back into the CSV. It is direct and uses the built-in capabilities of Python without the need for additional libraries.
Here’s an example:
import csv emails = ['[email protected]', '[email protected]', '[email protected]'] with open('people.csv', 'r') as infile, open('updated_people.csv', 'w', newline='') as outfile: reader = csv.DictReader(infile) fieldnames = reader.fieldnames + ['Email'] writer = csv.DictWriter(outfile, fieldnames=fieldnames) writer.writeheader() for row, email in zip(reader, emails): row['Email'] = email writer.writerow(row)
Output:
Name,Age,Occupation,Email Alice,30,Engineer,[email protected] Bob,24,Designer,[email protected] Carol,29,Manager,[email protected]
This code snippet creates a new CSV `updated_people.csv` with the appended “Email” column. The `csv.DictReader` and `csv.DictWriter` are utilized for reading and writing CSV files respectively. For each row read by the reader, a new entry for the email is added before the row is written to the `outfile`.
Method 2: Using pandas for simplicity
pandas is a powerful data manipulation library that simplifies operations on datasets. This method leverages pandas to load the CSV into a DataFrame, append the new column, and save the updated DataFrame back to a CSV file. It shines in its simplicity and is particularly useful for large datasets with complex operations.
Here’s an example:
import pandas as pd emails = ['[email protected]', '[email protected]', '[email protected]'] df = pd.read_csv('people.csv') df['Email'] = emails df.to_csv('updated_people.csv', index=False)
Output:
Name,Age,Occupation,Email Alice,30,Engineer,[email protected] Bob,24,Designer,[email protected] Carol,29,Manager,[email protected]
This snippet quickly loads a CSV file into a pandas DataFrame, appends an ‘Email’ column, and writes the DataFrame back to a new CSV. The `index=False` parameter ensures that the DataFrame index is not written as a separate column in the new CSV file.
Method 3: Appending with open file handles
This method involves working with file handles directly using Python’s built-in open function. Line by line, data is processed, the new column is appended, and the result is written to a new file. It is memory-efficient but can be less intuitive and slower for very large files.
Here’s an example:
emails = ['[email protected]', '[email protected]', '[email protected]'] with open('people.csv', 'r') as infile, open('updated_people.csv', 'w') as outfile: outfile.write(infile.readline().strip() + ',Email\n') # Write header for line, email in zip(infile, emails): outfile.write(line.strip() + ',' + email + '\n')
Output:
Name,Age,Occupation,Email Alice,30,Engineer,[email protected] Bob,24,Designer,[email protected] Carol,29,Manager,[email protected]
This code block demonstrates manually reading from one file and writing to another while adding a new column. Note that this approach requires manual handling of newlines and can become complex if the CSV involves special cases such as quoted fields with commas.
Method 4: Using csv module with DictReader and writerow
The csv module can also be used with the writerow method for more control over the writing process. This method provides a lower-level approach that can be advantageous for nuanced CSV handling but requires more boilerplate code compared to DictWriter.
Here’s an example:
import csv emails = ['[email protected]', '[email protected]', '[email protected]'] with open('people.csv', 'r') as infile, open('updated_people.csv', 'w', newline='') as outfile: reader = csv.reader(infile) writer = csv.writer(outfile) headers = next(reader) + ['Email'] writer.writerow(headers) for row, email in zip(reader, emails): writer.writerow(row + [email])
Output:
Name,Age,Occupation,Email Alice,30,Engineer,[email protected] Bob,24,Designer,[email protected] Carol,29,Manager,[email protected]
This code leverages the csv.reader and csv.writer for straightforward reading and writing. Each row from the original CSV is extended with the new email column before being written to the new file.
Bonus One-Liner Method 5: List Comprehension with File IO
A Python one-liner can achieve appending a column using list comprehension and file IO. This method is concise and Pythonic but potentially less readable and not advisable for very large files due to memory consumption.
Here’s an example:
emails = ['[email protected]', '[email protected]', '[email protected]'] with open('people.csv', 'r') as infile, open('updated_people.csv', 'w') as outfile: lines = infile.readlines() lines = [line.strip() + ',' + email + '\n' for line, email in zip(lines, ['Email'] + emails)] outfile.writelines(lines)
Output:
Name,Age,Occupation,Email Alice,30,Engineer,[email protected] Bob,24,Designer,[email protected] Carol,29,Manager,[email protected]
In this one-liner, file lines are read and with the new column data appended using list comprehension. The modified lines are then written back out. It’s a minimalistic approach that does the job with very little code.
Summary/Discussion
- Method 1: csv module with DictReader/DictWriter. Offers good control and readability. However, requires writing to a new file.
- Method 2: pandas. Simplifies complex data manipulations. It’s the most powerful for large datasets but introduces an external dependency.
- Method 3: Direct file handle manipulation. Memory efficient, yet can be error-prone with more complex CSV data structures.
- Method 4: csv module with reader/writer. More control over the file output but involves more code compared to using DictWriter.
- Method 5: One-liner with list comprehension. Quick and elegant for small files but less readable and can consume more memory for larger files.
March 02, 2024 at 03:41AM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
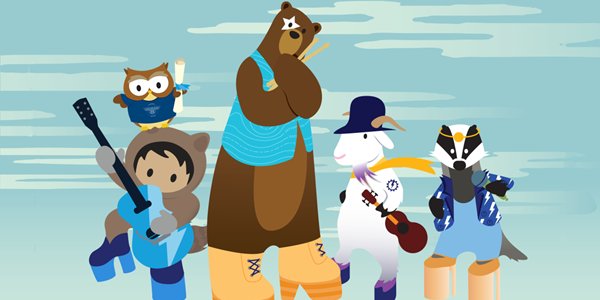
Post a Comment