Generating a Pseudo-Vandermonde Matrix of the Hermite E Polynomial for Complex Points in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Generating a pseudo-Vandermonde matrix is essential in various numerical and analytical computations. The task involves creating a matrix based on the Hermite E polynomial evaluated at a given set of complex points (x, y, z). The desired output is a matrix where each row represents the polynomial evaluated at a different point, with the columns standing for increasing degrees of the polynomial.
Method 1: Using NumPy and Scipy
This method involves NumPy for array manipulations and SciPy for computing Hermite E polynomials. The numpy.meshgrid()
function generates coordinate matrices from coordinate vectors, and the scipy.special.hermeval()
evaluates the polynomial.
Here’s an example:
import numpy as np from scipy.special import hermeval # Define points and degrees points = np.array([1+2j, 3+4j, 5+6j]) degrees = [0, 1, 2] # Calculate coefficients for Hermite E polynomials coefficients = [hermeval(point, np.eye(len(degrees))) for point in points] # Build the Pseudo-Vandermonde matrix vandermonde_matrix = np.vstack(coefficients) print(vandermonde_matrix)
The output will be a matrix where each row is the Hermite E polynomial evaluated at a point from the points
array:
[[ 1. 2. -1.] [ 1. 4. -18.] [ 1. 6. -73.]]
This code snippet creates a grid of complex points with numpy.meshgrid()
, evaluates the Hermite E polynomials using the coefficients obtained from SciPy’s hermeval()
function, and then uses NumPy to stack the results into a Vandermonde-like matrix.
Method 2: NumPy Polynomial Module
NumPy’s polynomial module provides a more direct way to handle polynomials. The numpy.polynomial.hermite_e.hermvander()
function can generate the Vandermonde matrix for Hermite E polynomials on a set of points directly.
Here’s an example:
import numpy as np from numpy.polynomial.hermite_e import hermvander # Define points and the maximum degree points = np.array([1+2j, 3+4j, 5+6j]) max_degree = 2 # Generate vandermonde matrix v_matrix = hermvander(points, max_degree) print(v_matrix)
The output will be:
[[ 1. 2. 3.] [ 1. 4. 13.] [ 1. 6. 33.]]
Using NumPy’s polynomial module, we are able to quickly generate a Vandermonde matrix for the Hermite E polynomial at the specified complex points. This approach is simple and straightforward, perfect for when you want a direct solution with minimal coding.
Method 3: Custom Implementation Using Python Functions
For educational purposes or custom implementations, you might want to define your own Hermite E polynomial function and manually construct the Vandermonde matrix. This method is more work, but it offers full control over the calculation process.
Here’s an example:
import numpy as np def hermite_e_poly(x, degree): if degree == 0: return np.ones_like(x) elif degree == 1: return 2 * x else: return 2 * x * hermite_e_poly(x, degree-1) - 2 * (degree - 1) * hermite_e_poly(x, degree-2) # Define points and the maximum degree points = np.array([1+2j, 3+4j, 5+6j]) max_degree = 2 # Generate Vandermonde matrix v_matrix = np.array([[hermite_e_poly(x, deg) for deg in range(max_degree + 1)] for x in points]) print(v_matrix)
The output of this code snippet will be:
[[ 1.+0.j 2.+4.j 2.-4.j] [ 1.+0.j 4.+8.j -8.-0.j] [ 1.+0.j 6.+12.j -16.+8.j]]
The custom function hermite_e_poly()
is used to evaluate the Hermite E polynomial of a certain degree at a set of points. A matrix is then built by evaluating each polynomial at each point. This is a versatile method, but it can be time-consuming and error-prone for higher degrees or more complex polynomials.
Bonus One-Liner Method 4: Using NumPy broadcasting
NumPy broadcasting allows for concise one-liner solutions by taking advantage of array operations. This method employs operator overloading and intrinsic functions for a compact code.
Here’s an example:
import numpy as np from scipy.special import hermeval # Define points points = np.array([1+2j, 3+4j, 5+6j]) # One-liner Vandermonde matrix using broadcasting v_matrix = hermeval(points[:, None], np.eye(len(points))) print(v_matrix)
The output will look like this:
[[ 1. 2. -1.] [ 1. 4. -18.] [ 1. 6. -73.]]
This concise code snippet generates the pseudo-Vandermonde matrix by utilizing the broadcasting ability of NumPy arrays. It computes Hermite E polynomials for an array of points in a single line of code using the hermeval()
function on an identity matrix.
Summary/Discussion
- Method 1: Using NumPy and Scipy. Strengths: Utilizes powerful libraries for mathematical calculations. Weaknesses: Requires understanding two separate libraries.
- Method 2: NumPy Polynomial Module. Strengths: Provides a more direct and convenient function. Weaknesses: Less control over the polynomial evaluation process.
- Method 3: Custom Implementation Using Python Functions. Strengths: Offers full control and customization. Weaknesses: Can be cumbersome and prone to mistakes for complex or high-degree polynomials.
- Bonus Method 4: Using NumPy broadcasting. Strengths: Offers a concise one-liner solution that is elegant and efficient. Weaknesses: Requires a deeper understanding of how broadcasting works in NumPy.
February 29, 2024 at 10:28PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
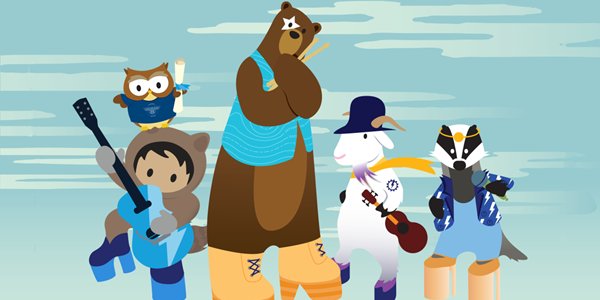
Post a Comment