5 Reliable Methods to Check if a Python String Contains a Substring : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: In Python programming, there are various scenarios where it becomes necessary to check if a string contains a specific substring. It’s a common task in text processing, data validation, and parsing tasks.
For instance, given the input string "The quick brown fox jumps over the lazy dog"
and the substring "brown"
, the aim is to figure out a method that confirms the presence of "brown"
within the input string.
Method 1: Using the “in” Keyword
The “in
” keyword in Python is a straightforward and readable way to check if a substring exists within a string. It returns a boolean value: True if the substring is found, and False otherwise. This method is highly readable and is considered Pythonic.
Here’s an example:
text = "Hello, welcome to the world of Python" substring = "Python" # Check if the substring is in the text result = substring in text print(result)
Output:
True
This code snippet demonstrates the simplicity of using the “in” keyword. We define a string and a substring, and then simply check for membership by using the keyword. The print statement will output True if the substring exists within the string, or False if it doesn’t.
Method 2: Using str.find()
The str.find()
method returns the lowest index of the substring if it is found in the string. If it’s not found, it returns -1. It’s a simple method that not only checks for the presence of a substring but also gives its position.
Here’s an example:
message = "Searching for a needle in a haystack" search = "needle" # Find the position of the substring in the string position = message.find(search) print(position != -1)
Output:
True
Here, the .find()
method is used to search for the substring. If 'search'
exists in 'message'
, position is the index of the first character of the first occurrence of 'search'
. If ‘search’ is not found, position is -1, and thus the print statement outputs False
.
Method 3: Using str.index()
The str.index()
function is similar to str.find()
in its operation, but instead of returning -1 when the substring is not found, it raises a ValueError
. This could be useful if you’d like your program to handle the absence of a substring differently than simply acknowledging its non-existence.
Here’s an example:
quote = "Insanity is doing the same thing over and over again and expecting different results." word = "Insanity" try: position = quote.index(word) result = True except ValueError: result = False print(result)
Output:
True
The example uses a try-except block because str.index()
will throw an error if the substring is not found. If ‘word’ is in ‘quote’, the variable ‘position’ gets the index, otherwise an exception is caught and ‘result’ is set to False.
Method 4: Using Regular Expressions
Regular expressions (regex) provide a powerful way to search for patterns within a string. The re.search()
method from Python’s re
module can be used to check for a substring match. It’s especially useful when the search pattern is complex.
Here’s an example:
import re lyrics = "I can't get no satisfaction" pattern = 'satisfaction' # Check for substring using regular expression search match = re.search(pattern, lyrics) print(match is not None)
Output:
True
By importing the re module, we can use the re.search()
method to look for ‘pattern
‘ in ‘lyrics
‘. If a match is found, re.search()
returns a match object; otherwise, it returns None
. We then simply check if ‘match
‘ is not None
to confirm the substring’s presence.
Bonus One-Liner Method 5: Using str.__contains__()
The magic method str.__contains__()
is actually what’s invoked in the background when we use the “in
” keyword. It’s not commonly used directly since it’s less readable, but it’s worth mentioning for its direct approach.
Here’s an example:
saying = "A stitch in time saves nine" phrase = "time" # Directly using the magic method contains = saying.__contains__(phrase) print(contains)
Output:
True
In direct use of the str.__contains__()
method, we can pass ‘phrase
‘ to the method associated with ‘saying
‘. The result is the same boolean output as you would get using the “in
” keyword.
Summary/Discussion
- Method 1: “
in
” Keyword. This method is Pythonic and straightforward, with no frills attached. However, it does not provide the substring’s position. - Method 2:
str.find()
. Returns the position of the substring, which can be useful. But its return value of -1 when the substring is not found may be inadvertently interpreted as a true boolean in a non-direct comparison. - Method 3:
str.index()
. Similar tostr.find()
but raises an exception if the substring isn’t found. It is useful when you need to catch the absence of a substring as an error condition in your program. - Method 4: Regular Expressions. Best for complex search patterns and offers sophisticated pattern matching options. However, it can be slower than the other methods and requires importing an additional module.
- Bonus Method 5:
str.__contains__()
. It offers a direct and explicit check but is generally not recommended for regular use due to lesser readability when compared to the “in
” keyword.
February 14, 2024 at 11:38PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
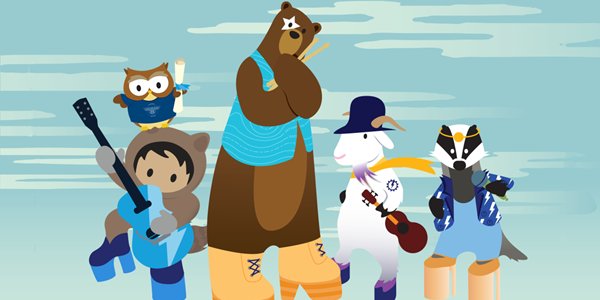
Post a Comment