5 Best Ways to Join a List of Strings with New Lines in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post

["apple", "banana", "cherry"]
, the desired output is a single string where each fruit is on a new line:
apple banana cherry
Method 1: Using a for loop and string concatenation
Python string concatenation is straightforward with a for loop. You can iterate through each string in the list and append it to an initially empty string, adding a newline character (‘\\n
‘) after each element except the last one.
Here’s an example:
fruits = ["apple", "banana", "cherry"] result = "" for fruit in fruits: result += fruit + "\n" print(result.strip())
Output:
apple banana cherry
This code snippet intelligently constructs a new string by adding each fruit name to it, followed by a newline. The trailing newline is removed with strip()
to ensure the string ends correctly.
Method 2: Using the str.join() method
Python’s str.join()
method is a cleaner, more Pythonic way to concatenate items from a list into a single string, separated by a specified string – in this case, a newline character.
Here’s an example:
fruits = ["apple", "banana", "cherry"] result = "\n".join(fruits) print(result)
Output:
apple banana cherry
With join()
, we specify the newline as the separator and join the list of strings into a single string. This method operates cleanly without any need for post-processing.
Method 3: Using a list comprehension
A list comprehension in Python is a compact way of creating lists. It can be used to create the list of strings with newlines and then join them using the str.join()
method.
Here’s an example:
fruits = ["apple", "banana", "cherry"] result = "\n".join([fruit for fruit in fruits]) print(result)
Output:
apple banana cherry
This method is similar to Method 2, but illustrates the use of a list comprehension, which can come in handy if you need to process the strings in some way before joining them.
Method 4: Using map and str.join()
The Python map()
function is useful for applying a function to every item of an iterable. Here we apply the str function to ensure each list element is a string, and then join them with newlines.
Here’s an example:
fruits = ["apple", "banana", "cherry"] result = "\n".join(map(str, fruits)) print(result)
Output:
apple banana cherry
The map()
function iterates over each element in the list and ensures it is a string. This is useful if the list contains non-string elements. The join()
method then concatenates them as before.
Bonus One-Liner Method 5: Using a generator expression with str.join()
A generator expression is a memory-efficient and concise way to iterate through items in a container. Paired with str.join()
, it can join a list of strings with new lines in one concise line of code.
Here’s an example:
fruits = ["apple", "banana", "cherry"] result = "\n".join(fruit for fruit in fruits) print(result)
Output:
apple banana cherry
A generator expression is used within the join()
method to iterate through the list without creating an intermediate list, saving memory when dealing with large lists.
Summary/Discussion
- Method 1: For Loop Concatenation. Straightforward but not the most efficient. It is verbose and can be slow for large lists due to string immutability.
- Method 2: str.join(). Pythonic and efficient. This is generally the best method for simple concatenation.
- Method 3: List Comprehension. Offers more flexibility than
join()
alone if additional processing is needed. Still concise and efficient. - Method 4: map with str.join(). Useful if the list contains non-strings. However, it abstracts away the operation, which can be less clear to read.
- Bonus Method 5: Generator Expression. Memory-efficient and Pythonic, suitable for very large lists.
February 18, 2024 at 07:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
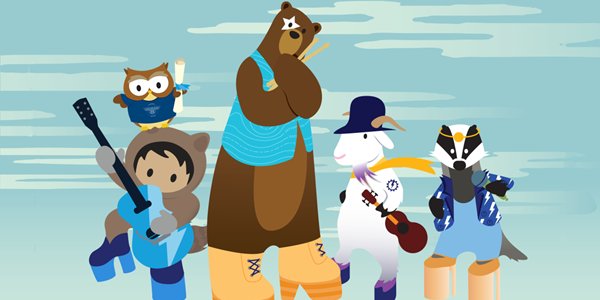
Post a Comment