5 Best Ways to Group a List of Dicts by Value in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Python developers often need to organize data efficiently. Grouping a list of dictionaries by shared values is a common task. For example, given a list of dictionaries representing employees with ‘name’ and ‘department’ keys, the goal is to group these records by their ‘department’ value to easily manage the departmental headcounts, resources, or other department-specific computations.
Method 1: Using defaultdict
The collections.defaultdict
function can simplify the process of grouping a list of dictionaries by a specific key’s value. By passing a list to defaultdict
, you create a dictionary whose default values are lists. Items can then be appended to these lists based on their grouping key.
Here’s an example:
from collections import defaultdict # Sample list of dictionaries employees = [ {'name': 'Alice', 'department': 'Engineering'}, {'name': 'Bob', 'department': 'Sales'}, {'name': 'Charlie', 'department': 'Engineering'} ] # Using defaultdict to group by 'department' department_groups = defaultdict(list) for emp in employees: department_groups[emp['department']].append(emp) # Printing grouped dictionaries print(dict(department_groups))
Output:
{ 'Engineering': [{'name': 'Alice', 'department': 'Engineering'}, {'name': 'Charlie', 'department': 'Engineering'}], 'Sales': [{'name': 'Bob', 'department': 'Sales'}] }
This code snippet iterates through the list of employee dictionaries. For each employee, it appends their dictionary to a list in department_groups
under the corresponding department key. By converting the defaultdict back to a regular dict with dict(department_groups)
, we get a clear snapshot of the employees organized by department.
Method 2: Using itertools.groupby
The itertools.groupby
function is a versatile tool for grouping iterable elements. When working with a list of dictionaries, one aspect to note is that the list must first be sorted by the key intended for grouping since groupby
groups consecutive items with the same key.
Here’s an example:
from itertools import groupby from operator import itemgetter # Sample list of dictionaries employees = [ {'name': 'Alice', 'department': 'Engineering'}, {'name': 'Bob', 'department': 'Sales'}, {'name': 'Charlie', 'department': 'Engineering'} ] # Sorting by 'department' for grouping employees.sort(key=itemgetter('department')) # Grouping by 'department' using groupby grouped_employees = {k: list(g) for k, g in groupby(employees, itemgetter('department'))} # Printing grouped dictionaries print(grouped_employees)
Output:
{ 'Engineering': [{'name': 'Alice', 'department': 'Engineering'}, {'name': 'Charlie', 'department': 'Engineering'}], 'Sales': [{'name': 'Bob', 'department': 'Sales'}] }
In this example, the itemgetter
utility helps to sort the list of employees by the ‘department’ key. Then groupby
is applied to the sorted list to group the dictionaries. Finally, we create a dictionary comprehension to convert the grouped items into a single dictionary that maps each department to a list of its employees’ dictionaries.
Method 3: Using a simple for loop
Sometimes simplest solutions are the best. You can use a straight-forward approach with a for loop to iterate over the list and group the dictionaries. This method requires no additional imports and leverages basic Python data structures.
Here’s an example:
# Sample list of dictionaries employees = [ {'name': 'Alice', 'department': 'Engineering'}, {'name': 'Bob', 'department': 'Sales'}, {'name': 'Charlie', 'department': 'Engineering'} ] # Grouping by 'department' using a for loop department_groups = {} for emp in employees: key = emp['department'] if key not in department_groups: department_groups[key] = [] department_groups[key].append(emp) # Printing grouped dictionaries print(department_groups)
Output:
{ 'Engineering': [{'name': 'Alice', 'department': 'Engineering'}, {'name': 'Charlie', 'department': 'Engineering'}], 'Sales': [{'name': 'Bob', 'department': 'Sales'}] }
The for loop in this example checks if the ‘department’ already has a key in the department_groups
dictionary. If not, it initializes an empty list. Regardless, the employee’s dictionary is appended to the list corresponding to their department.
Method 4: Using pandas DataFrame
For those who work with data manipulation, pandas is an invaluable resource. Using a DataFrame, you can easily group by any column and handle complex data operations, making it ideal for more sophisticated tasks beyond simple grouping.
Here’s an example:
import pandas as pd # Sample list of dictionaries employees = [ {'name': 'Alice', 'department': 'Engineering'}, {'name': 'Bob', 'department': 'Sales'}, {'name': 'Charlie', 'department': 'Engineering'} ] # Create a DataFrame and group by 'department' df = pd.DataFrame(employees) grouped_df = df.groupby('department').apply(lambda x: x.to_dict('records')).to_dict() # Printing grouped dictionaries print(grouped_df)
Output:
{ 'Engineering': [{'name': 'Alice', 'department': 'Engineering'}, {'name': 'Charlie', 'department': 'Engineering'}], 'Sales': [{'name': 'Bob', 'department': 'Sales'}] }
The pandas example creates a DataFrame from the list of dictionaries and then uses groupby
followed by apply
to transform each group into a list of dictionaries. The to_dict
method at the end returns the result in a dictionary format, grouped by the specified column.
Bonus One-Liner Method 5: Using a generator expression
Python’s generator expressions can be cleverly used to create compact, one-liner solutions for problems like grouping dictionaries by a key’s value. Here, a dictionary comprehension and a generator expression are combined for a succinct solution.
Here’s an example:
# Sample list of dictionaries employees = [ {'name': 'Alice', 'department': 'Engineering'}, {'name': 'Bob', 'department': 'Sales'}, {'name': 'Charlie', 'department': 'Engineering'} ] # One-liner grouping by 'department' grouped_employees = {k: [d for d in employees if d['department'] == k] for k in set(d['department'] for d in employees)} # Printing the result print(grouped_employees)
Output:
{ 'Engineering': [{'name': 'Alice', 'department': 'Engineering'}, {'name': 'Charlie', 'department': 'Engineering'}], 'Sales': [{'name': 'Bob', 'department': 'Sales'}] }
This compact example relies on a set to create a unique set of department names, then for each unique department, it includes a list of dictionaries where the ‘department’ key matches. It’s an excellent demonstration of the power of Python comprehensions.
Summary/Discussion
- Method 1: Using
collections.defaultdict
. Strengths: It’s simple and relatively efficient. Weaknesses: Requires import fromcollections
module. - Method 2: Using
itertools.groupby
. Strengths: Very efficient for large datasets, especially when they are already sorted. Weaknesses: Requires the data to be sorted on the group key. - Method 3: Using a simple for loop. Strengths: Requires no imports and is easy to understand. Weaknesses: Possibly less efficient with very large datasets.
- Method 4: Using pandas DataFrame. Strengths: Ideal for complex data manipulations and integrates well with other data analysis tasks. Weaknesses: Overhead of using a large external library.
- Bonus Method 5: Using a generator expression. Strengths: Compact and pythonic. Weaknesses: Can be less readable for those unfamiliar with comprehensions.
February 22, 2024 at 07:25PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
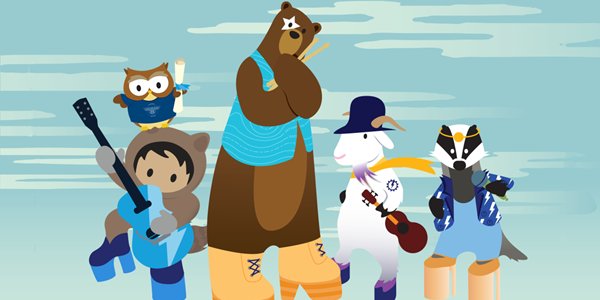
Post a Comment