5 Best Ways to Create a List of Dictionaries in Python : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Often in Python, we need to organize data in a way that pairs each key with a corresponding value, making dictionaries the ideal structure. However, when one needs to store multiple such records, a list of dictionaries becomes essential. This article solves the problem of manipulating a collection of records such as [{‘name’: ‘Alice’, ‘age’: 25}, {‘name’: ‘Bob’, ‘age’: 30}, …] by showcasing different methods to create a list of dictionaries in Python.
Method 1: Using a Loop and Append
This method involves initializing an empty list and then using a for loop to create dictionaries, which are appended to the list. This is the most intuitive method and is most suitable when adding dictionaries procedurally based on some logic or conditions during iteration.
Here’s an example:
list_of_dicts = [] for i in range(5): list_of_dicts.append({'id': i, 'square': i**2}) print(list_of_dicts)
Output:
[{'id': 0, 'square': 0}, {'id': 1, 'square': 1}, {'id': 2, 'square': 4}, {'id': 3, 'square': 9}, {'id': 4, 'square': 16}]
This code snippet starts by initializing an empty list. The loop goes from 0 to 4, and in each iteration, it appends a new dictionary to the list, containing an ‘id’ and its ‘square’. It is easy to understand and control, especially for those new to Python.
Method 2: List Comprehension
List comprehension in Python allows you to write concise and readable code for generating lists. By incorporating dictionary creation into list comprehension, you can construct a list of dictionaries in a single line of code.
Here’s an example:
list_of_dicts = [{'id': i, 'square': i**2} for i in range(5)] print(list_of_dicts)
Output:
[{'id': 0, 'square': 0}, {'id': 1, 'square': 1}, {'id': 2, 'square': 4}, {'id': 3, 'square': 9}, {'id': 4, 'square': 16}]
This code utilizes list comprehension to create the same list of dictionaries as Method 1 but in a more succinct manner. The expression inside the brackets defines the structure of each dictionary, which is generated for each value of ‘i’ in the specified range.
Method 3: Using the map()
Function
The map()
function can be used to apply a function to each item in an iterable. When combined with a lambda function that returns dictionaries, map()
can efficiently create a list of dictionaries.
Here’s an example:
list_of_dicts = list(map(lambda i: {'id': i, 'square': i**2}, range(5))) print(list_of_dicts)
Output:
[{'id': 0, 'square': 0}, {'id': 1, 'square': 1}, {'id': 2, 'square': 4}, {'id': 3, 'square': 9}, {'id': 4, 'square': 16}]
By mapping a lambda function over a range of numbers, dictionaries are created for each number and then converted into a list. This method is elegant for those who prefer functional programming.
Method 4: Using the dict()
Function and a Zip
You can pair the dict()
function with the zip()
function to construct dictionaries from two parallel lists, one containing keys and the other containing values. This is useful when your data is already segregated into separate lists.
Here’s an example:
keys = ['id', 'square'] values = [[i, i**2] for i in range(5)] list_of_dicts = [dict(zip(keys, v)) for v in values] print(list_of_dicts)
Output:
[{'id': 0, 'square': 0}, {'id': 1, 'square': 1}, {'id': 2, 'square': 4}, {'id': 3, 'square': 9}, {'id': 4, 'square': 16}]
This snippet creates a list of keys and a list of values. It then zips the keys with each sublist of values, generating a dictionary, and proceeds to aggregate these into a list using list comprehension. This method is highly readable and useful for parallel data sequences.
Bonus One-Liner Method 5: Using a Generator
A generator expression can be utilized to generate a sequence of dictionaries. When typecast to a list, it becomes a compact and memory-efficient way to build a list of dictionaries, especially useful for large datasets.
Here’s an example:
list_of_dicts = list({'id': i, 'square': i**2} for i in range(5)) print(list_of_dicts)
Output:
[{'id': 0, 'square': 0}, {'id': 1, 'square': 1}, {'id': 2, 'square': 4}, {'id': 3, 'square': 9}, {'id': 4, 'square': 16}]
The generator expression within the list function is a more memory-efficient alternative to the list comprehension method. It avoids creating the entire list in memory at once, making it preferable when working with a huge number of dictionaries.
Summary/Discussion
- Method 1: Using a Loop and Append. Intuitive and easy for beginners. Con: Can be verbose for simple cases.
- Method 2: List Comprehension. Compact and Pythonic. Con: May be less readable for complex logic.
- Method 3: Using the
map()
Function. Functional programming style. Con: Some findmap()
less intuitive than comprehensions. - Method 4: Using the
dict()
Function and a Zip. Clean and readable for parallel data. Con: Requires data to be well-organized in advance. - Method 5: Using a Generator. Memory-efficient for large datasets. Con: Slightly less straightforward than list comprehension for small datasets.
February 22, 2024 at 07:25PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
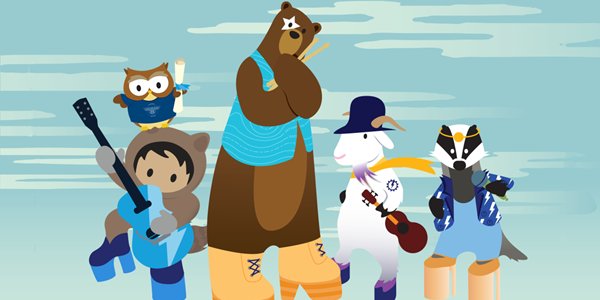
Post a Comment