5 Best Ways to Convert a Python HTML String to JSON : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: Developers often need to parse HTML content and extract information in a structured JSON format. For instance, you might have an HTML table that you want to convert into a JSON array of objects. This article discusses how to take an HTML string in Python – like
'<div>Example</div>'
– and convert it into a JSON format, such as '{"element": "Example"}'
.
Method 1: Using BeautifulSoup and json libraries
BeautifulSoup is a Python library for parsing HTML and XML documents. It provides tools for navigating and searching the parse tree. After extracting the desired data using BeautifulSoup, you can format and dump the data into JSON using the json
library.
Here’s an example:
from bs4 import BeautifulSoup import json # Your HTML string html_string = '<div>Example</div>' soup = BeautifulSoup(html_string, "html.parser") # Extract the text within the div tag data_text = soup.find("div").text # Convert the extracted text to a JSON object json_output = json.dumps({"element": data_text}) print(json_output)
The output of this code snippet:
{"element": "Example"}
This code snippet demonstrates the extraction of text from a <div>
tag in the HTML string and then converts that text into a Python dictionary which is thereafter serialized to a JSON string.
Method 2: Using lxml and json.dumps
The lxml
library allows for easy handling of XML and HTML files, and it is known for its performance. You can parse the HTML and extract elements using XPaths. After organizing the extracted data as a Python dictionary, you can use json.dumps
to create the JSON representation.
Here’s an example:
from lxml import etree import json html_string = '<div>Example</div>' root = etree.HTML(html_string) # Assuming you want to extract the content of the div extracted_data = root.xpath('//div/text()')[0] json_output = json.dumps({"element": extracted_data}) print(json_output)
The output of this code snippet:
{"element": "Example"}
In this snippet, the lxml
library parses the HTML and extracts the desired text using XPath. The result is then turned into a JSON string using json.dumps
.
Method 3: Using html.parser and json modules
The built-in HTML parser in Python can be used to parse HTML data. The html.parser
module offers a class with methods that can be overridden to handle start tags, end tags, data, and more. After parsing, the data is converted into JSON with the json
module.
Here’s an example:
from html.parser import HTMLParser import json class MyHTMLParser(HTMLParser): def __init__(self): super().__init__() self.data = [] def handle_data(self, data): self.data.append(data) html_string = '<div>Example</div>' parser = MyHTMLParser() # Feed the HTML content to the parser parser.feed(html_string) # After parsing, the data is in a list that can be converted into a JSON json_output = json.dumps({"element": parser.data[0]}) print(json_output)
The output of this code snippet:
{"element": "Example"}
This example demonstrates creating a custom parser by subclassing HTMLParser
and then using it to extract data from an HTML string. The extracted data is then packaged in a JSON format.
Method 4: Using regex and json modules
Regular expressions can be utilized for simple HTML strings to extract the content of tags, although it is generally not recommended for complex HTML parsing due to the potential for irregularities in HTML structures. Once the needed content is matched using regex, the json
module can be used to convert the data to JSON.
Here’s an example:
import re import json html_string = '<div>Example</div>' match = re.search(r'<div>(.*?)</div>', html_string) json_output = json.dumps({"element": match.group(1)}) print(json_output)
The output of this code snippet:
{"element": "Example"}
This snippet uses a regular expression to find a pattern in an HTML string that gets the content inside a <div>
tag. The matched data is then encoded into JSON format using json.dumps
.
Bonus One-Liner Method 5: Using a list comprehension and json.dump
For particularly straightforward HTML, a Python one-liner using list comprehension and json.dump
can be an elegant and concise solution. This method is ideal for quickly converting strings and is not suited for complex parsing tasks.
Here’s an example:
import json html_string = '<div>Example</div>' json_output = json.dumps([{"element": content} for content in re.findall(r'<div>(.*?)</div>', html_string)]) print(json_output)
The output of this code snippet:
[{"element": "Example"}]
This snippet uses a list comprehension to search for all occurrences of a pattern in the HTML string and constructs a list of dictionaries, which is then dumped to a JSON formatted string.
Summary/Discussion
- Method 1: BeautifulSoup and json. Strengths: Robust and reliable for complex HTML. Weaknesses: External dependency, may be overkill for simple tasks.
- Method 2: lxml and json.dumps. Strengths: Very fast and efficient. Weaknesses: External dependency and requires some familiarity with XPaths.
- Method 3: html.parser and json modules. Strengths: No external dependencies, built into Python. Weaknesses: Can be verbose for simple tasks.
- Method 4: regex and json modules. Strengths: Quick for simple HTML strings. Weaknesses: Inadvisable for complex HTML, risks improper parsing.
- Bonus Method 5: One-liner with list comprehension and json.dumps. Strengths: Extremely concise. Weaknesses: Not suitable for complex parsing, limited by regex matching capabilities.
February 18, 2024 at 07:11PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
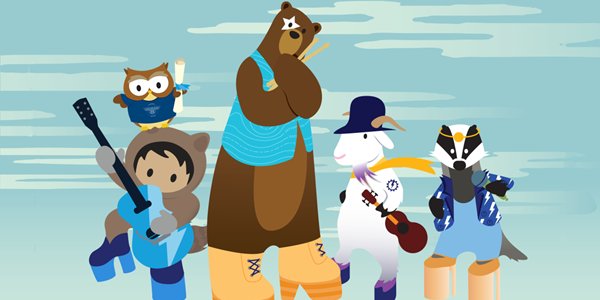
Post a Comment