Mobile App Development with Python and Kivy: Your Guide to Building Apps Easily : Emily Rosemary Collins
by: Emily Rosemary Collins
blow post content copied from Be on the Right Side of Change
click here to view original post
Getting Started with Kivy and Python

Embarking on your app development journey with Python can be an exciting venture, especially with the Kivy framework. Kivy is a free and open-source Python library that simplifies the process of building cross-platform applications. With this guide, you’ll set up your development environment and create your first basic application.
Setting Up the Development Environment
Before you dive into creating your first mobile app, you need to prepare your development environment. This setup is crucial for a smooth Kivy experience. Start by ensuring you have Python installed on your system. Kivy is compatible across Linux, Windows, and macOS platforms, and it’s vital to download the correct Python version for your operating system.
Once Python is set up, the next step is to establish a virtual environment. This is a sandbox that allows you to manage dependencies for different projects without conflicts.
You can create a virtual environment using python -m venv kivy_venv
and activate it with
source kivy_venv/bin/activate
on Linux/macOS or
kivy_venv\Scripts\activate
on Windows.
Now it’s time to install Kivy. With your virtual environment active, use pip, the Python package installer, to add Kivy to your project by running pip install kivy
.
Creating Your First Kivy App
After setting up your environment, you’re ready to create your inaugural Kivy app.
Begin by importing Kivy’s App class alongside other necessary components, like the Label class, which you’ll use to display text.
The outline of your first ‘HelloWorld’ app would look like this:
from kivy.app import App from kivy.uix.label import Label class HelloWorldApp(App): def build(self): return Label(text='Hello, World!') if __name__ == '__main__': HelloWorldApp().run()
In this simple app, the build
method of your HelloWorldApp
class returns a Label with the text 'Hello, World!'
.
When you run this script, a window should pop up displaying your message.
To add interactive elements, like a button, you would import the Button
class and attach an event. The on_press
event triggers a function whenever the button is pressed:
from kivy.uix.button import Button class HelloWorldApp(App): def build(self): def on_button_press(instance): print('The button was pressed!') button = Button(text='Press me') button.bind(on_press=on_button_press) return button
With these steps completed, you’ve successfully built a Kivy application that responds to user interactions. As you grow more comfortable with Kivy’s components and event handling, you’ll be able to expand your ‘HelloWorld’ app into more complex projects.
Designing the User Interface

When you’re creating a mobile app with Kivy, the user interface (UI) is where users interact with your application. It’s important to make this experience intuitive and engaging.
Understanding Kivy Widgets
Widgets are the building blocks of your UI in Kivy. Each widget is an element such as a Button, Label, or TextInput, which users will interact with. It’s your job to decide where these widgets go and how they behave.
For example, a Button widget is not only defined by its size and color, but also by the events it triggers when touched. Here’s a quick list of common widgets you might use:
- Button: Triggers an action on press.
- Label: Displays text.
- TextInput: Allows text input from users.
Kivy allows you to customize these widgets with various properties, such as text
, font_size
, and background_color
, to fit the design and functionality of your app.
Managing Layouts and Events
Managing Layouts: The layout of your widgets is vital for a polished UI. You’ll work with layouts like BoxLayout and GridLayout to structure your widgets.
BoxLayout arranges widgets in a horizontal or vertical line, based on the orientation
property.
To arrange widgets in a matrix, you’d use GridLayout, configuring its cols
or rows
properties to set the number of columns or rows.
- BoxLayout: Simple linear arrangement, either vertical or horizontal.
- GridLayout: More complex matrix-style arrangements.
Managing Events: Widgets also have events that your app must handle. When a user presses a button, the button dispatches an ‘on_press
‘ event. Here’s how you might bind a function to a Button’s event:
def on_button_press(instance): print('The button <%s> was pressed!' % instance.text) btn = Button(text='Click me!') btn.bind(on_press=on_button_press)
In this example, pressing the button will trigger the on_button_press
function, which can contain the logic for what should happen next.
Structuring Your Kivy Project

When you start your Kivy project, remember that a solid structure is the backbone of any good application. Organize your files by separating your app logic from your app class, and make sure to follow object-oriented programming (OOP) principles. This approach not only makes your code cleaner but also easier to manage.
A common project structure might look like this:
- main.py: The entry point of your app which runs the app class.
- YourAppName/: A directory containing:
- app_class.py: Defines your app class.
- screens/: Contains different screen files for your app.
- uix/: Custom widgets or user interface elements go here.
- assets/: All your images, fonts, and other static resources.
- data/: For non-static data files, like configuration or JSON files.
Refer to this tutorial on Python Kivy projects that can provide some excellent examples to get you started.
Debugging and Optimization
As you develop your application, you’ll inevitably encounter bugs. Use Kivy’s built-in debugging tools to help you troubleshoot issues in real-time.
Tools like the Kivy Inspector allow you to interactively alter widget properties to see immediate changes. For optimization, profile your application to identify bottlenecks. Remember to:
- Write efficient logic by reducing redundant operations.
- Use compile-time techniques, such as Cython to speed up performance.
Resource optimization is particularly critical; ensure that graphics and sound files are optimally compressed without losing quality.
Deploying to Mobile Platforms
With a stable and polished application in hand, you’re ready to deploy to mobile platforms like Android and iOS. You’ll utilize tools like Buildozer or Python-for-Android to package your Kivy application. It’s crucial to test on actual devices to ensure compatibility across different platforms and screen sizes. Here’s what you need to do:
- Install all necessary dependencies for compiling the app for your target platform.
- Configure your build specifications appropriately in your
buildozer.spec
file. - Run the build command and fix any issues that arise during the compilation process.
For more details on the process, especially for Android, you can view this guide on building an Android application with Kivy Python framework.
By adhering to these project development and best practices, you’ll maximize the benefits of using Kivy in the mobile app development space and set your mobile applications up for success across various platforms.
Extended Learning and Community Resources

As you delve deeper into mobile app development with Python using Kivy, it’s valuable to connect with the community and enhance your skills through additional resources. Beyond just constructing a basic calculator application, you have the opportunity to contribute to a bustling open-source community and further your learning through comprehensive materials.
Contributing to Open Source
Kivy falls under the MIT License, making it a prime candidate for your contributions. You can give back and support Kivy, which remains open-source and relies on community efforts for growth and improvement. Begin by exploring Kivy’s official website, which includes detailed information on how to contribute, whether it’s by submitting patches for bugs or suggesting new features.
Beeware and PyQt are other Python GUI frameworks that welcome contributions as well. Each project has its own set of guidelines for contributors, which can typically be found on their respective websites or repositories.
Further Learning Resources
Tutorials and Examples:
For a structured approach to learning, consider diving into an in-depth video course such as the one found on Udemy for Python KivyMD. Courses like this provide hands-on examples, including building a graphical user interface and creating cross-platform applications.
Community and Packages:
Joining forums and discussions can provide real-world insights and help. The Kivy community can be found on platforms like Kivy’s Google Group or the Python Discord, where you can engage with other developers, ask questions, and share your experiences.
Web Development with Flask:
If you’re interested in extending your Python knowledge to web applications, explore Flask. Although not directly related to Kivy, Flask is a lightweight web application framework that can complement your understanding of Python in client-side and server-side environments. You’ll find extensive documentation and tutorials that can teach you the ropes of web development.
Remember, the purpose of these resources is to empower your learning journey—you’re not just passively consuming information, but actively engaging in the vibrant Python community.
Frequently Asked Questions

In this section, you’ll find common questions and clear answers to help you navigate mobile app development using Python and Kivy.
How can I create a mobile app using Kivy in Python?
To create a mobile app using Kivy in Python, you need to install Kivy on your machine, design your app’s layout using Kv language, and then add functionality with Python code. Kivy’s documentation provides a step-by-step guide on how to get started.
What are the benefits of using Python with Kivy for mobile app development?
Using Python with Kivy offers benefits such as cross-platform compatibility, allowing you to write your app once and deploy it on multiple platforms. Kivy’s use of Python makes it highly readable and maintainable, which can speed up development. For detailed benefits, you can refer to this informative article.
Where can I find examples of mobile apps built with Kivy?
You can find examples of mobile apps developed with Kivy by exploring projects shared in the Kivy community or by checking out showcase apps on the official Kivy website.
Can you provide a tutorial to help beginners start with Kivy for mobile app development?
Absolutely, for beginners looking to start with Kivy, there are tutorials available that guide you through building attractive mobile apps in Python. Here is one such Kivy Python tutorial that demonstrates the process.
Is the Kivy framework actively maintained and updated for developing mobile apps?
Yes, Kivy is an open-source framework that is actively maintained and frequently updated, ensuring it remains a relevant tool for mobile app development. You can follow their updates and contributions on the Kivy GitHub repository.
How do I download and set up the Kivy environment for Android app development?
To download and set up Kivy for Android app development, follow the instructions on their installation page, which includes steps for setting up your environment on various operating systems, including Android.
December 25, 2023 at 10:46PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Emily Rosemary Collins
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
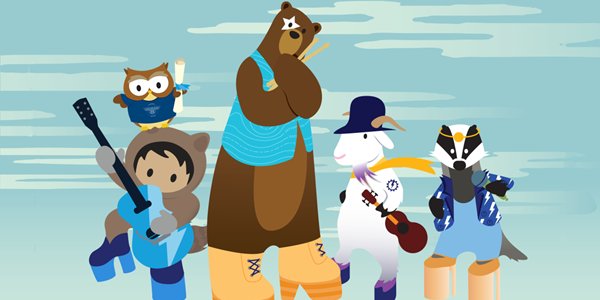
Post a Comment