How to Open Multiple Text Files in Python (6 Best Methods) : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Method 1: Multiple Context Managers
To open multiple text files in Python, you can use the open()
function for each file.
Here’s a basic example of how to do it:
# Open the first file with open('file1.txt', 'r') as file1: contents1 = file1.read() # Open the second file with open('file2.txt', 'r') as file2: contents2 = file2.read() # Open the third file with open('file3.txt', 'r') as file3: contents3 = file3.read() # Now contents1, contents2, and contents3 have the contents of file1.txt, file2.txt, and file3.txt, respectively
In this code:
'r'
indicates that the file is being opened in read mode.with open(...) as ...
is a context manager that automatically handles closing the file once you exit the block.file1.read()
,file2.read()
, andfile3.read()
read the entire contents of each file.
Replace 'file1.txt'
, 'file2.txt'
, and 'file3.txt'
with the actual names of your files.
Background information on opening files with Python in this video:

Method 2: A Single Context Manager
You can open multiple files within a single context manager by using a tuple.
Here’s how you can do it:
with open('file1.txt', 'r') as file1, open('file2.txt', 'r') as file2, open('file3.txt', 'r') as file3: contents1 = file1.read() contents2 = file2.read() contents3 = file3.read() # Now contents1, contents2, and contents3 have the contents of file1.txt, file2.txt, and file3.txt, respectively
This approach ensures that all files are opened in read mode and are automatically closed when the block is exited, efficiently using a single context manager.
Method 3: Nested Context Managers
You can use nested context managers to open multiple files in Python. This approach is useful when you want to handle each file separately within its own context.
Here’s an example:
# Open the first file with open('file1.txt', 'r') as file1: contents1 = file1.read() # Nested context manager for the second file with open('file2.txt', 'r') as file2: contents2 = file2.read() # Nested context manager for the third file with open('file3.txt', 'r') as file3: contents3 = file3.read() # At this point, contents1, contents2, and contents3 have the contents of file1.txt, file2.txt, and file3.txt, respectively
In this structure, each file is opened within the context of the previous file’s context manager. This nesting ensures that each file is properly opened and closed in sequence.
Method 4: List and Loop
You can store the filenames in a list and loop through them, opening and reading each file within the loop. This is particularly useful when dealing with a large number of files or when the file names can be dynamically generated.
filenames = ['file1.txt', 'file2.txt', 'file3.txt'] contents = [] for filename in filenames: with open(filename, 'r') as file: contents.append(file.read()) # contents[0], contents[1], and contents[2] have the contents of file1.txt, file2.txt, and file3.txt, respectively
Method 5: Using map with a Function
If you prefer a more functional programming approach, you can use map
with a function that opens and reads a file.
def read_file(filename): with open(filename, 'r') as file: return file.read() filenames = ['file1.txt', 'file2.txt', 'file3.txt'] contents = list(map(read_file, filenames)) # contents[0], contents[1], and contents[2] have the contents of file1.txt, file2.txt, and file3.txt, respectively
Method 6: File Object List
You can create a list of file objects and iterate over them. This method is less commonly used and is generally less preferred due to the manual handling of file closures.
files = [open('file1.txt', 'r'), open('file2.txt', 'r'), open('file3.txt', 'r')] contents = [file.read() for file in files] # Close the files for file in files: file.close() # contents[0], contents[1], and contents[2] have the contents of file1.txt, file2.txt, and file3.txt, respectively
December 14, 2023 at 08:05PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
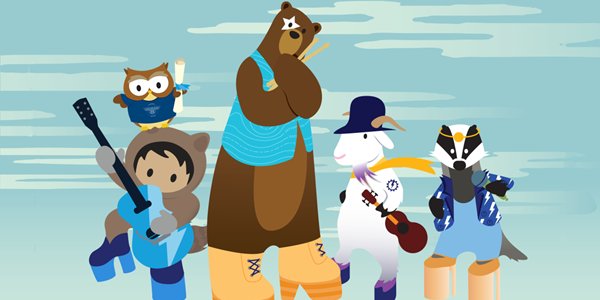
Post a Comment