Python Repeat String Until Length : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
The multiplication operator (*
) allows you to repeat a given string a certain number of times. However, if you want to repeat a string to a specific length, you might need to employ a different approach, such as string slicing or a while loop.
For example, while manipulating strings in Python, you may need to fill up a given output with a repeating sequence of characters. To do this, you can create a user-defined function that takes two arguments: the original string, and the desired length of the output. Inside the function, you can use the divmod()
function to determine the number of times the original string can be repeated in the output, as well as the remaining characters needed to reach the specified length. Combine this with string slicing to complete your output.
def repeat_to_length(string_to_expand, length): # Determine how many times the string should be repeated full_repeats, leftover_size = divmod(length, len(string_to_expand)) # Repeat the string fully and then add the leftover part result_string = string_to_expand * full_repeats + string_to_expand[:leftover_size] return result_string # Test the function original_string = "abc" desired_length = 10 output = repeat_to_length(original_string, desired_length) print(output) # Output: abcabcabca
In addition to using a custom function, you can also explore other Python libraries such as numpy
or itertools
for similar functionality. With a clear understanding of these techniques, you’ll be able to repeat strings to a specified length in Python with ease and improve your code’s efficiency and readability.
Understanding the Concept of Repeat String Until Length
In Python, you may often find yourself needing to repeat a string for a certain number of times or until it reaches a specified length. This can be achieved by using the repetition operator, denoted by an asterisk *
, which allows for easy string replication in your Python code.
Importance of Repeating a String Until a Specified Length
Repeating a string until a specific length is an essential technique in various programming scenarios. For example, you might need to create patterns or fillers in text, generate large amounts of text from templates, or add padding to align your output data.
Using Python’s string repetition feature, you can repeat a string an integer number of times. Take the following code snippet as an example:
string = 'abc' repeated_string = string * 7 print(repeated_string)
This code will output 'abcabcabcabcabcabcabc'
, as the specified string has been repeated seven times. However, let’s say you want to repeat the string until it reaches a certain length.
You can achieve this by using a combination of repetition and slicing:
string = 'abc' desired_length = 10 repeated_string = (string * (desired_length // len(string) + 1))[:desired_length] print(repeated_string)
This code will output 'abcabcabca'
, as the specified string has been repeated until it reaches the desired length of 10 characters.
Approach Using String Multiplication
Again, this method takes advantage of Python’s built-in multiplication operator *
to replicate and concatenate the string multiple times.
Suppose you have a string s
that you want to repeat until it reaches a length of n
. You can simply achieve this by multiplying the string s
by a value equal to or greater than n
divided by the length of s
.
Here’s an example:
def repeat_string(s, n): repeat_count = (n // len(s)) + 1 repeated_string = s * repeat_count return repeated_string[:n]
In this code snippet, (n // len(s)) + 1
determines the number of times the string s
should be repeated to reach a minimum length of n
. The string is then multiplied by this value using the *
operator, and the result is assigned to repeated_string
.
However, this repeated_string
may exceed the desired length n
. To fix this issue, simply slice the repeated string using Python’s list slicing syntax so that only the first n
characters are kept, as shown in repeated_string[:n]
.
Here’s how you can use this function:
original_string = "abc" desired_length = 7 result = repeat_string(original_string, desired_length) print(result) # Output: "abcabca"
In this example, the function repeat_string
repeats the original_string
"abc"
until it reaches the desired_length
of 7 characters.
Approach Using For Loop
In this method, you will use a for
loop to repeat a string until it reaches the desired length. The goal is to create a new string that extends the original string as many times as needed, while keeping the character order.
First, initialize an empty string called result
. Then, use a for loop with the range()
function to iterate until the length of the result
string is less than the specified length you desire. In each iteration of the loop, append a character from the original string to the result
string.
Here’s a step by step process on how to achieve this:
- Initialize an empty string named
result
. - Create a for loop with the
range()
function as its argument. Set the range to the desired length. - Inside the loop, calculate the index of the character from the original string that should be appended to the
result
string. You can achieve this by using the modulo operator, dividing the current index of the loop by the length of the original string. - Append the character found in step 3 to the
result
string. - Continue the loop until the length of the
result
string is equal to or greater than the desired length. - Finally, print or return the
result
string as needed.
Here’s a sample Python code illustrating this approach:
def repeat_string(string, length): result = "" for i in range(length): index = i % len(string) result += string[index] return result original_string = "abc" desired_length = 7 repeated_string = repeat_string(original_string, desired_length) print(repeated_string) # Output: 'abcabca'
Approach Using While Loop
In this approach, you will learn how to repeat a string to a certain length using a while loop in Python. This method makes use of a string variable and an integer to represent the desired length of the repeated string.
First, you need to define a function that takes two arguments: the input string, and the target length. Inside the function, create an empty result string and initialize a variable called index
to zero. This variable will be used to keep track of our position in the input string.
def repeat_string_while(input_string, target_length): result = "" index = 0
Next, use a while
loop to repeat the string until the result string reaches the specified length. In each iteration of the loop, append the character at the current index to the result. Increment the index after each character is added and use the modulus operator %
to wrap the index around when you reach the end of the input string.
while len(result) < target_length: result += input_string[index] index = (index + 1) % len(input_string)
Finally, return the resulting repeated string.
return result # Example usage: repeated_string = repeat_string_while("abc", 7) print(repeated_string) # Output: "abcabca"
In this example, the input string "abc"
is repeated until the target length of 7 is achieved. The resulting string is "abcabca"
. By using a while loop and some basic arithmetic, you can easily repeat a string to a specific length.
Here’s the complete example code:
def repeat_string_while(input_string, target_length): result = "" index = 0 while len(result) < target_length: result += input_string[index] index = (index + 1) % len(input_string) return result # Example usage: repeated_string = repeat_string_while("abc", 7) print(repeated_string) # Output: "abcabca"
Approach Using User-Defined Function
In this section, we will discuss an approach to repeat a string until it reaches a desired length using a user-defined function in Python. This method is helpful when you want to create a custom solution to meet specific requirements, while maintaining a clear and concise code.
First, let’s define a user-defined function called repeat_string_to_length
.
This function will take two arguments: the string_to_repeat
and the desired length
.
Inside the function, you can calculate the number of times the string must be repeated to reach the desired length. To achieve this, you can use the formula: (length // len(string_to_repeat)) + 1
. The double slashes //
represent integer division, ensuring the result is an integer.
Once you have determined the number of repetitions, you can repeat the input string using the Python string repetition operator (*
). Multiply the string_to_repeat
by the calculated repetitions and then slice the result to ensure it matches the desired length.
Here is an example of how your function may look:
def repeat_string_to_length(string_to_repeat, length): repetitions = (length // len(string_to_repeat)) + 1 repeated_string = string_to_repeat * repetitions return repeated_string[:length]
Now that you have defined the repeat_string_to_length
function, you can use it with various input strings and lengths. Here’s an example of how to use the function:
input_string = "abc" desired_length = 7 result = repeat_string_to_length(input_string, desired_length) print(result) # Output: "abcabca"
Exploring ‘Divmod’ and ‘Itertools’
In your journey with Python, you must have come across two handy built-in functions: divmod
and itertools
. These functions make it easier for you to manipulate sequences and perform calculations related to division and modulo operations.
divmod()
is a Python built-in function that accepts two numeric arguments and returns a tuple containing the quotient and the remainder when performing integer division. For example divmod(7, 3)
would return (2, 1)
, as 7 divided by 3 gives a quotient of 2 with a remainder of 1.
Here’s how to use it in your code:
quotient, remainder = divmod(7, 3) print(quotient, remainder)
On the other hand, you have the powerful itertools
module, which provides an assortment of functions for working with iterators. These functions create efficient looping mechanisms and can be combined to produce complex iterator algorithms.
For instance, the itertools.repeat()
function allows you to create an iterator that endlessly repeats a given element. However, when combined with other functions, you can generate a sequence of a specific length.
Now, let’s say you want to repeat a string until it reaches a certain length. You can utilize the itertools
module along with divmod to achieve this. First, calculate how many times you need to repeat the string and then use the itertools.chain()
function to create a string with the desired length:
import itertools def repeat_string_until_length(string, target_length): repetitions, remainder = divmod(target_length, len(string)) repeated_string = string * repetitions return ''.join(itertools.chain(repeated_string, string[:remainder])) result = repeat_string_until_length('Hello', 13) print(result)
This function takes a string and a target length and uses divmod to get the number of times the string must be repeated (repetitions) and the remainder. Next, it concatenates the repeated string and the appropriate slice of the original string to achieve the desired length.
Usage of Integer Division
In Python, you can use integer division to efficiently repeat a string until it reaches a certain length. This operation, denoted by the double slash //
, divides the operands and returns the quotient as an integer, effectively ignoring the remainder.
Suppose you have a string a
and you want to repeat it until the resulting string has a length of at least ra
. Begin by calculating the number of repetitions needed using integer division:
num_repeats = ra // len(a)
Now, if ra
is not divisible by the length of a
, the above division will not account for the remainder. To include the remaining characters, increment num_repeats
by one:
if ra % len(a) != 0: num_repeats += 1
Finally, you can create the repeated string by multiplying a
with num_repeats
:
repeated_string = a * num_repeats
This method allows you to quickly and efficiently generate a string that meets your length requirements.
Using Numpy for String Repetition
Numpy, a popular numerical computing library in Python, provides an efficient way to repeat the string until a specified length. Although Numpy is primarily used for numerical operations, you can leverage it for string repetition with some modifications. Let’s dive into the process.
First, you would need to install Numpy if you haven’t already. Simply run the following command in your terminal:
pip install numpy
To begin with, you can create a function that will utilize the Numpy function numpy.tile()
to repeat a given string. Because Numpy does not directly handle strings, you can break them into Unicode characters and then use Numpy to manipulate the array.
Here’s a sample function:
import numpy as np def repeat_string_numpy(string, length): input_array = np.array(list(string), dtype='U1') repetitions = (length // len(string)) + 1 repeated_chars = np.tile(input_array, repetitions) return ''.join(repeated_chars[:length])
In this function, your string is converted to an array of characters using list()
, and the data type is set to Unicode with ‘U1’. Next, you calculate the number of repetitions required to reach the desired length. The numpy.tile()
function then repeats the array accordingly.
Finally, you slice the resulting array to match the desired length and join the characters back into a single string.
Here’s an example of how to use this function:
result = repeat_string_numpy("abc", 7) print(result) # Output: 'abcabca'
Example Scenarios
In this section, we’ll discuss a few Practical Coding Examples to help you better understand how to repeat a string in Python until it reaches a desired length. We will walk through various scenarios, detailing the code and its corresponding output.
Practical Coding Examples
- Simple repetition using the multiplication operator One of the most basic ways to repeat a string in Python is by using the multiplication operator. This will help you quickly achieve your desired string length.
Here’s an example:
string = "abc" repetitions = 3 result = string * repetitions print(result)
Output:
abcabcabc
- Repeating a string until it matches the length of another string Suppose you have two strings and you’d like to repeat one of them until its length matches that of the other. You can achieve this by calculating the number of repetitions required and using the remainder operator to slice the string accordingly.
Here’s an example:
string1 = "The earth is dying" string2 = "trees" length1 = len(string1) length2 = len(string2) repetitions = length1 // length2 + 1 result = (string2 * repetitions)[:length1] print(result)
Output:
treestreest
- Repeating a string to an exact length If you want to repeat a string until it reaches an exact length, you can calculate the necessary number of repetitions and use slicing to obtain the desired result.
Here’s an example:
string = "abc" desired_length = 7 length = len(string) repetitions = desired_length // length + 1 result = (string * repetitions)[:desired_length] print(result)
Output:
abcabca
Recommended: How to Repeat a String Multiple Times in Python
The post Python Repeat String Until Length appeared first on Be on the Right Side of Change.
September 04, 2023 at 04:00PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
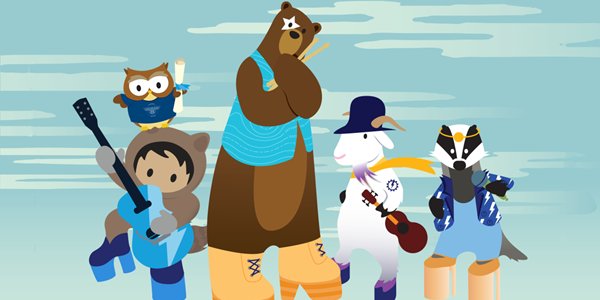
Post a Comment