Python for Beginners: Convert XML to INI Format in Python :
by:
blow post content copied from Planet Python
click here to view original post
We use XML and INI file formats for storing configuration data for software systems. This article discusses how to convert an XML file or string to an INI file in Python.
What is XML File Format?
XML stands for eXtensible Markup Language. XML files are plain text files that contain structured data in a hierarchical format.
At the most basic level, an XML file consists of a single root element that contains all the other elements in the file. Each element in the XML file is defined by a tag that identifies the element and its purpose. For example, we can define a tag <title>
to indicate that the enclosed text is the title of a document.
For instance, consider the following XML file.
<?xml version="1.0" encoding="UTF-8"?>
<data>
<employee>
<name>John Doe</name>
<age>35</age>
</employee>
<job>
<title>Software Engineer</title>
<department>IT</department>
<years_of_experience>10</years_of_experience>
</job>
<address>
<street>123 Main St.</street>
<city>San Francisco</city>
<state>CA</state>
<zip>94102</zip>
</address>
</data>
The above XML file represents a set of data related to an employee, their job, and their address.
- The first line of the XML file specifies the version and encoding of the XML file.
- The root element of the file is
<data>
. It contains three child elements namely<employee>
,<job>
, and<address>
. - The
<employee>
element contains two child elements i.e.<name>
and<age>
. The<name>
element contains the name of the employee as a text node, while the<age>
element contains the age of the employee as a text node. <job>
element contains three child elements i.e.<title>
,<department>
, and<years_of_experience>
. The<title>
element contains the job title as text data. The<department>
element contains the department name as a text node and the<years_of_experience>
element contains the number of years of experience as a text node.- The
<address>
element contains four child elements i.e.<street>
,<city>
,<state>
, and<zip>
. The<street>
element contains the street address as a text node, the<city>
element contains the city name as a text node, the<state>
element contains the state name as a text node, and the<zip>
element contains the zip code as a text node.
We can define the structure of an XML file by a Document Type Definition (DTD) or an XML Schema, which specifies the allowable elements, attributes, and values that can be used in the file. This helps to ensure that XML files are well-formed and consistent.
One of the benefits of using XML is that it is extensible. It means that we can define our own elements and attributes to represent data in a way that is specific to our needs. This flexibility makes XML a popular choice for exchanging data between different applications and systems.
What is the INI File Format?
INI stands for “initialization”. It refers to a file format used to store configuration settings for various applications and operating systems. The INI file format is a simple text-based format that consists of a set of sections, each containing a set of key-value pairs.
An INI file typically contains one or more sections. Here, each section is enclosed in square brackets ([]
). The section name appears on a line by itself enclosed in brackets. Each section declaration is followed by one or more key-value pairs.
Each key-value pair consists of a key and a value, separated by an equal sign (=). The key is a string that identifies the setting, and the value is the setting’s value.
For example, we can represent the data given in the XML file using the INI format as shown below.
[employee]
name=John Doe
age=35
[job]
title=Software Engineer
department=IT
years_of_experience=10
[address]
street=123 Main St.
city=San Francisco
state=CA
zip=94102
You can observe the increased readability and simplicity of the data.
The INI file format is simple and easy to read and write. This makes it popular for storing configuration settings for various applications and operating systems. However, it has some limitations, such as the lack of support for hierarchical structures and the inability to store complex data types. As a result, other formats, such as XML and JSON, are often used for more complex configurations.
In the next sections of the article, we discuss different ways to convert an XML file or string to INI format using the configparser module and the xmltodict module in Python.
XML String to INI File in Python
To convert an XML string to an INI file, we will use the xmltodict module and the configparser module. For this, we will use the following steps.
- First, we will open an INI file in write mode using the
open()
function to store the output file. Theopen()
function takes the file name as its first input argument and the Python literal“w”
as its second input argument. After execution, it returns a file pointer. - Next, we will create an empty
ConfigParser
object using theConfigParser()
function defined in the configparser module. We will use theConfigParser
object to create the INI file. - Now, we will use the
parse()
method defined in the xmltodict module to convert xml string to a Python dictionary. Theparse()
method takes the XML string as its input argument and returns a Python dictionary. - We know that the INI files do not contain hierarchical data. However, XML files are stored like a tree structure. Here, the root node in the xml file contains no data. So, we will remove the key corresponding to the root node of the xml file from the dictionary.
- After removing the key containing the root node from the dictionary, we will read the data from the Python dictionary into the
ConfigParser
object. - First, we will add sections to the
ConfigParser
object. For this, we will use theadd_section()
method defined in the configparser module. Theadd_section()
method takes the section name as the input argument and adds it to theConfigParser
object. - To add the sections, we will first get the outer keys of the dictionary using the
keys()
method. Then, we will iterate over the list of keys and add them as sections in theConfigParser
object. - After adding sections, we will add fields to each section. For this, we will use the
set()
method. Theset()
method, when invoked on aConfigParser
object, takes three input arguments. The first argument is the section name, the second argument is the field name, and the third input argument is the field value. After execution, it adds the field to the corresponding section in theConfigParser
object. - To add the field names to the
ConfigParser
object, we will use the outer keys in the dictionary to access the inner dictionaries and iterate through the key-value pairs. For each section, we will iterate through the key-value pairs in the inner dictionary and add them to theConfigParser
object using theset()
method. - After reading all the data into the
ConfigParser
object, we will dump it into the INI file using thewrite()
method. Thewrite()
method, when invoked on aConfigParser
object, takes the file pointer to the INI file as its input. After execution, it writes the data from theConfigParser
object to the INI file. - Finally, we will
close()
the file using theclose()
method.
By using the above steps, we can easily convert an XML string to an INI file in Python. You can observe this in the following example.
import xmltodict
import configparser
xml_string="""<?xml version="1.0" encoding="utf-8"?>
<data>
<employee>
<name>John Doe</name>
<age>35</age>
</employee>
<job>
<title>Software Engineer</title>
<department>IT</department>
<years_of_experience>10</years_of_experience>
</job>
<address>
<street>123 Main St.</street>
<city>San Francisco</city>
<state>CA</state>
<zip>94102</zip>
</address>
</data>
"""
file =open("employee1.ini","w")
xml_dict=xmltodict.parse(xml_string)
outer_key=list(xml_dict.keys())[0]
xml_dict = xml_dict[outer_key]
config_object = configparser.ConfigParser()
sections=list(xml_dict.keys())
for section in sections:
config_object.add_section(section)
for section in sections:
inner_dict=xml_dict[section]
fields=inner_dict.keys()
for field in fields:
value=inner_dict[field]
config_object.set(section, field, str(value))
config_object.write(file)
file.close()
The output INI file looks as follows.
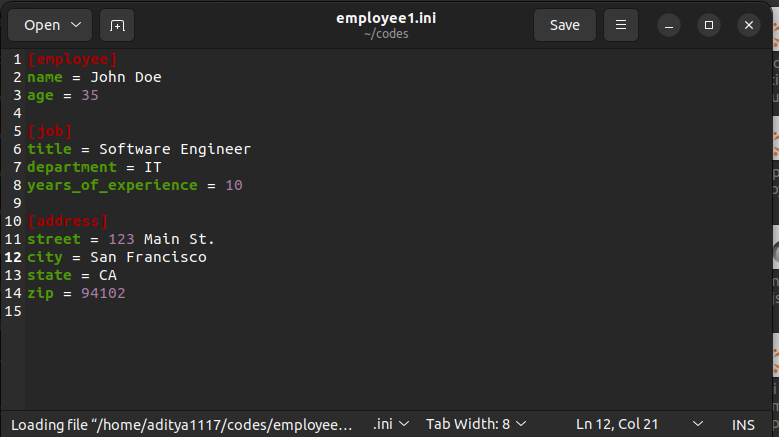
The code used in the above example has a higher execution time. We can rewrite the above code in a more Pythonic way as shown below.
import xmltodict
import configparser
xml_string="""<?xml version="1.0" encoding="utf-8"?>
<data>
<employee>
<name>John Doe</name>
<age>35</age>
</employee>
<job>
<title>Software Engineer</title>
<department>IT</department>
<years_of_experience>10</years_of_experience>
</job>
<address>
<street>123 Main St.</street>
<city>San Francisco</city>
<state>CA</state>
<zip>94102</zip>
</address>
</data>
"""
file =open("employee1.ini","w")
xml_dict=xmltodict.parse(xml_string)
outer_key=list(xml_dict.keys())[0]
xml_dict = xml_dict[outer_key]
config_object = configparser.ConfigParser()
sections=list(xml_dict.keys())
for section, options in xml_dict.items():
config_object.add_section(section)
for key, value in options.items():
config_object.set(section, key, str(value))
config_object.write(file)
file.close()
The output of the above code will be the same as the previous code.
Convert XML File to INI File in Python
To convert an XML file to an INI file, we will open the XML file using the open()
function. Next, we will use the read()
method to read the contents of the XML file in a string. Once, we get the XML string from the file, we can convert the string to an INI file using the approach discussed above.
For example, consider that we have the following XML file.
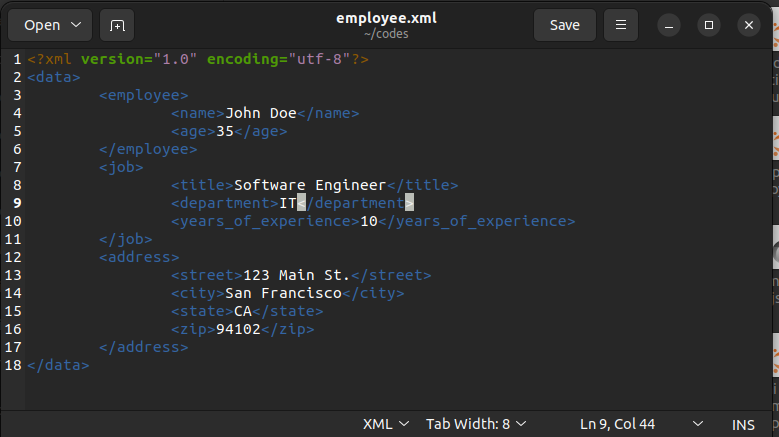
We can convert the above XML file into an INI file in Python as shown below.
import xmltodict
import configparser
xml_file=open("employee.xml","r")
xml_string=xml_file.read()
xml_dict=xmltodict.parse(xml_string)
outer_key=list(xml_dict.keys())[0]
xml_dict = xml_dict[outer_key]
config_object = configparser.ConfigParser()
for section, options in xml_dict.items():
config_object.add_section(section)
for key, value in options.items():
config_object.set(section, key, str(value))
file =open("employee.ini","w")
config_object.write(file)
file.close()
The output file looks as follows.
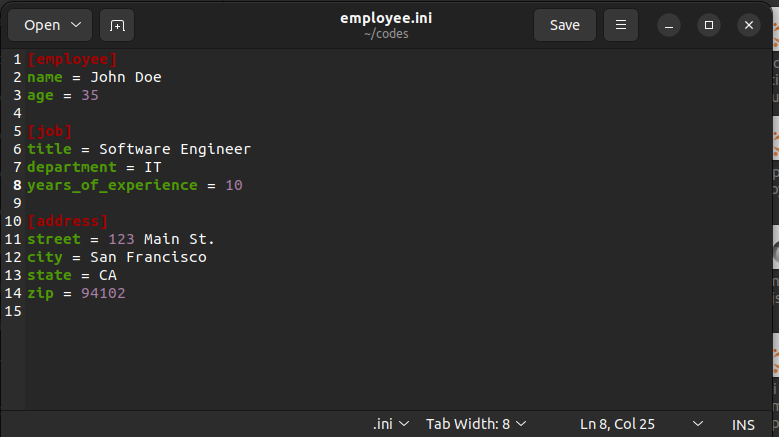
Conclusion
In this article, we discussed different ways to convert an XML file or string to an INI file in Python. To learn more about file conversions, you can read this article on how to convert ini to xml in Python. You might also like this article on how to insert a row in a pandas dataframe.
I hope you enjoyed reading this article. Stay tuned for more informative articles.
Happy Learning!
The post Convert XML to INI Format in Python appeared first on PythonForBeginners.com.
April 07, 2023 at 06:30PM
Click here for more details...
=============================
The original post is available in Planet Python by
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
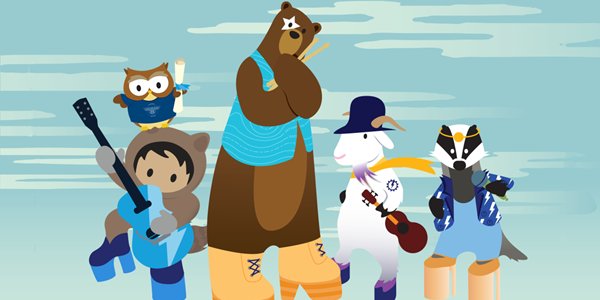
Post a Comment