Python Create List From 1 to N with Step Size : Chris
by: Chris
blow post content copied from Be on the Right Side of Change
click here to view original post
Problem Formulation: How to create a list from 1 to n with a specified step in Python?
Let’s start with one of the easiest ways:
Method 1. Using range()
in List Comprehension
Using [i for i in range(1, n+1, step)]
is the most straightforward method using built-in Python functions: list comprehension and range()
.
list_with_step = [i for i in range(1, n + 1, step)]
Method 2. Using numpy.arange()
For those using the NumPy library, particularly effective for large data sets due to its optimized performance.
import numpy as np list_with_step = np.arange(1, n + 1, step).tolist()
Method 3. Using List Slicing
Generate a full list first and then use slicing to select every step
th element.
list_with_step = list(range(1, n + 1))[::step]
Method 4. Using itertools.islice
with itertools.count
Utilizes lazy evaluation, ideal for very large ranges.
from itertools import islice, count list_with_step = list(islice(count(1, step), (n - 1) // step + 1))
Method 5. Using a for
loop with Index Management
Explicitly controls the index, providing clarity at the cost of more verbose code.
list_with_step = [] for i in range(1, n + 1): if (i - 1) % step == 0: list_with_step.append(i)
Method 6. Using filter
with range
Applies a filter function to a range, extracting elements based on a condition.
list_with_step = list(filter(lambda x: (x - 1) % step == 0, range(1, n + 1)))
Method 7. Using a while
loop
This method manually controls the addition of elements to the list.
list_with_step = [] i = 1 while i <= n: list_with_step.append(i) i += step
Method 8. Using itertools.takewhile
with itertools.count
Combines two itertools functions to generate a controlled sequence that stops when a condition is met.
from itertools import takewhile, count list_with_step = list(takewhile(lambda x: x <= n, count(1, step)))
Method 9. Using map
and range
Incorporates a transformation directly within the map function for adjusting step intervals.
list_with_step = list(map(lambda x: 1 + (x * step), range((n + step - 1) // step)))
Method 10. Advanced Conditional List Comprehension
Incorporates condition checks directly within a list comprehension for greater control.
list_with_step = [i for i in range(1, n + 1) if (i - 1) % step == 0]
Also check out my related article:
Python Create List From 1 to N
April 22, 2024 at 05:24PM
Click here for more details...
=============================
The original post is available in Be on the Right Side of Change by Chris
this post has been published as it is through automation. Automation script brings all the top bloggers post under a single umbrella.
The purpose of this blog, Follow the top Salesforce bloggers and collect all blogs in a single place through automation.
============================
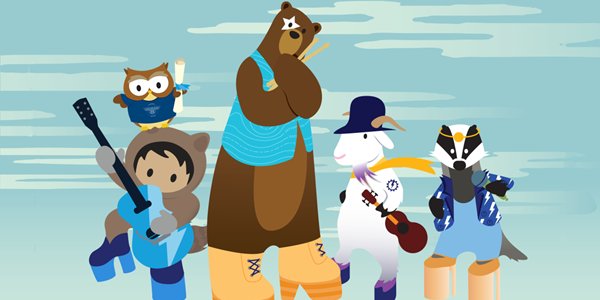
Post a Comment